Radar Installation
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 49381 | Accepted: 11033 |
Description
Assume the coasting is an infinite straight line. Land is in one side of coasting, sea in the other. Each small island is a point locating in the sea side. And any radar installation, locating on the coasting, can only cover d distance, so an island in the sea can be covered by a radius installation, if the distance between them is at most d.
We use Cartesian coordinate system, defining the coasting is the x-axis. The sea side is above x-axis, and the land side below. Given the position of each island in the sea, and given the distance of the coverage of the radar installation, your task is to write a program to find the minimal number of radar installations to cover all the islands. Note that the position of an island is represented by its x-y coordinates.
Figure A Sample Input of Radar Installations
We use Cartesian coordinate system, defining the coasting is the x-axis. The sea side is above x-axis, and the land side below. Given the position of each island in the sea, and given the distance of the coverage of the radar installation, your task is to write a program to find the minimal number of radar installations to cover all the islands. Note that the position of an island is represented by its x-y coordinates.
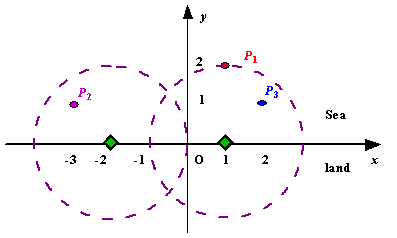
Figure A Sample Input of Radar Installations
Input
The
input consists of several test cases. The first line of each case
contains two integers n (1<=n<=1000) and d, where n is the number
of islands in the sea and d is the distance of coverage of the radar
installation. This is followed by n lines each containing two integers
representing the coordinate of the position of each island. Then a blank
line follows to separate the cases.
The input is terminated by a line containing pair of zeros
The input is terminated by a line containing pair of zeros
Output
For
each test case output one line consisting of the test case number
followed by the minimal number of radar installations needed. "-1"
installation means no solution for that case.
Sample Input
3 2 1 2 -3 1 2 1 1 2 0 2 0 0
Sample Output
Case 1: 2 Case 2: 1
这个题可以用贪心做,我们以每个岛作为圆心,以r为半径画圆,会交到横坐标上两点(l,r),将两点存起来然后排序,可以按l排,也可以按r来排,这个随意,如果没有交点,则输出-1.
排完序之后,会发现有很多重叠的区域,找到有几个重叠区域即可。
/*====================================================================== * Author : kevin * Filename : RadarInstallation.cpp * Creat time : 2014-05-14 15:46 * Description : ========================================================================*/ #include <iostream> #include <algorithm> #include <cstdio> #include <cstring> #include <queue> #include <cmath> #define clr(a,b) memset(a,b,sizeof(a)) #define M 1005 #define INF (1<<30) using namespace std; struct Node { double x,y; double l,r; }node[M]; int n,m; bool cmp(struct Node a,struct Node b) { if(a.r == b.r){ return a.l < b.l; } return a.r>b.r; } void calcu(int i) { double r = sqrt(m*m-node[i].y*node[i].y); node[i].l = node[i].x-r; node[i].r = node[i].x+r; } int main() { int cas = 1; while(scanf("%d%d",&n,&m)!=EOF && n+m){ clr(node,0); int flag = 0; for(int i = 0; i < n; i++){ scanf("%lf%lf",&node[i].x,&node[i].y); if(node[i].y > m){ flag = 1; } calcu(i); } if(flag){ printf("Case %d: -1 ",cas++); continue; } sort(node,node+n,cmp); int cnt = 1; int s = 0; for(int i = 1; i < n; i++){ if(node[i].r < node[s].l){ cnt++; s = i; } else if(node[i].l >= node[s].l){ s = i; } } printf("Case %d: %d ",cas++,cnt); } return 0; }