第1章 WCF简介
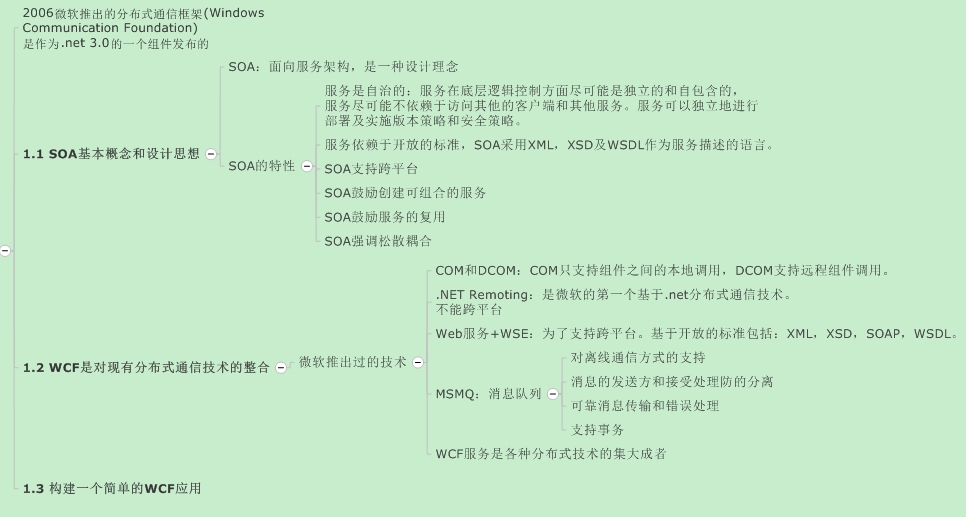
面向服务架构(SOA)是近年来备受业界关注的一个主题,它代表了软件架构的一种方向。顺应SOA发展潮流,微软于2006年年底推出了一种新的分布式通信框架Windows Communication Foundation,简称WCF。WCF是作为.NET Framework3.0的一个组件发布的。
1.1 SOA基本概念和设计思想
SOA就是采用Web服务的架构吗?
面向服务(Service Orientation,SO)代表的是一种设计理念,和面向对象(Object Orientation,OO),面向组件(Component Orientation,CO)一样,体现的是一种对关注点进行分解的思想,面向服务是和技术无关的。
服务是自治的
服务的自治原则要求单个服务在底层逻辑控制方面尽可能是独立和自包含的,服务尽可能不依赖于访问它的客户端和其他服务。服务可以独立地进行部署及实施版本策略和安全策略。
SOA依赖于开发的标准
SOA的一个目标是让不同厂商开发的服务能够进行互操作。SOA采用基于消息的通信方式。SOA采用XML,XSD及WSDL作为服务描述的“语言”。
SOA支持跨平台
SOA鼓励创建可组合的服务
SOA鼓励服务的复用
SOA强调松耦合
1.2 WCF是对现有分布式通信技术的整合
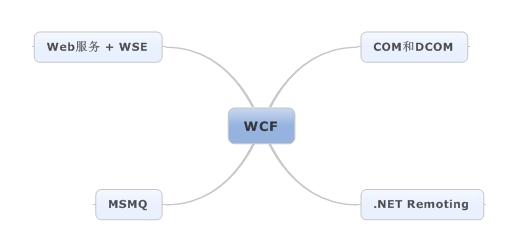
图1-1 WCF是对现有分布式通信技术的整合
1.3 构建一个简单的WCF应用
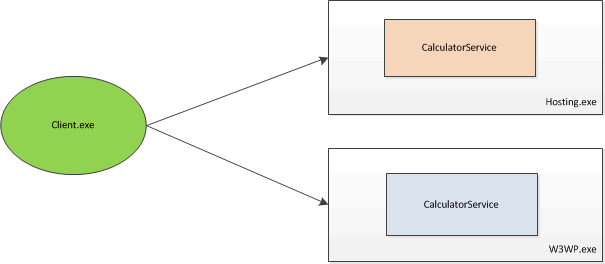
图1-2 客户端与服务进程之间的调用关系
添加配置文件App.config如下:
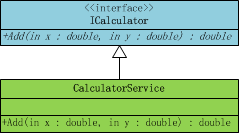
图1-3 WCF契约的类图
1.3.1 新建WCF服务
(1)、新建一个解决方案,ConsoleHost,同时新建一个控制台应用程序ConsoleHost。
(2)、添加一个类库 IService
新建一个接口ICalculator,注意添加服务引用,System.ServiceModel, 代码如下:
using System.ServiceModel; namespace IService { [ServiceContract(Name="CalculatorService", Namespace="http://www.artech.com")] public interface ICalculator { [OperationContract] double Add(double x, double y); } }
(3)、添加一个类库 Service
新建一个类CalculatorService是实现接口ICalculator。代码如下:
using IService; namespace Service { public class CalculatorService:ICalculator { public double Add(double x, double y) { return x + y; } } }
(4)、完善 ConsoleHost 的代码
添加对Service类库的引用,代码如下:
using System; using System.ServiceModel; using Service; namespace ConsoleHost { class Program { static void Main(string[] args) { using (ServiceHost host = new ServiceHost(typeof(CalculatorService))) { host.Opened += delegate { Console.WriteLine("CalculatorService已经启动,按任意键终止服务!"); }; host.Open(); Console.Read(); } } } }
<?xml version="1.0" encoding="utf-8" ?> <configuration> <system.serviceModel> <behaviors> <serviceBehaviors> <behavior name="metadataBehavior"> <serviceMetadata httpGetEnabled="false"/> </behavior> </serviceBehaviors> </behaviors> <services> <service name="Service.CalculatorService" behaviorConfiguration="metadataBehavior"> <endpoint address="http://127.0.0.1:3721/calculatorservice" binding="wsHttpBinding" contract="IService.ICalculator"/> </service> </services> </system.serviceModel> </configuration>
(5)、Web宿主,添加新建项目,选择WCF服务应用程序,项目名称为 WebHost
添加对Service类库的引用,同时创建一个CalculatorService.svc文件,代码如下:
<%@ ServiceHost Language="C#" Debug="true" Service="Service.CalculatorService"%>
注意修改Web.config里面的代码,关键部分如下:
<system.serviceModel> <services> <service name="Service.CalculatorService" behaviorConfiguration="WebHost.Service1Behavior"> <!-- Service Endpoints --> <endpoint address="" binding="wsHttpBinding" contract="IService.ICalculator"> <!-- 部署时,应删除或替换下列标识元素,以反映 在其下运行部署服务的标识。删除之后,WCF 将 自动推导相应标识。 --> <identity> <dns value="localhost" /> </identity> </endpoint> <endpoint address="mex" binding="mexHttpBinding" contract="IMetadataExchange" /> </service> </services> <behaviors> <serviceBehaviors> <behavior name="WebHost.Service1Behavior"> <!-- 为避免泄漏元数据信息,请在部署前将以下值设置为 false 并删除上面的元数据终结点--> <serviceMetadata httpGetEnabled="true" /> <!-- 要接收故障异常详细信息以进行调试,请将以下值设置为 true。在部署前设置为 false 以避免泄漏异常信息--> <serviceDebug includeExceptionDetailInFaults="false" /> </behavior> </serviceBehaviors> </behaviors> </system.serviceModel>
1.3.2 运行WCF服务
(1)、运行控制台宿主服务
控制台运行WCF服务比较简单,之间运行ConsoleHost项目启动即可,运行成功如下图:
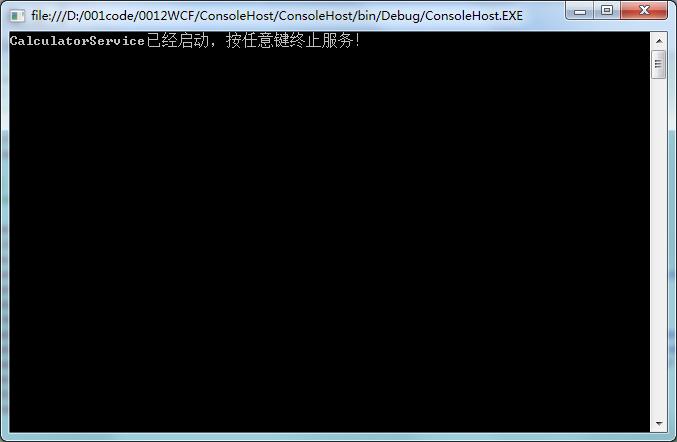
(2)、部署IIS上的WCF服务
第一步、添加网站
第二步、修改版本
第三步、启动IIS成功
1.3.3 客户端调用WCF服务
新建一个解决方案WCFClient,添加一个控制台项目WCFClient。在解决方案下面新建一个文件夹DLL,将IService的dll,拷贝到DLL文件夹下,并添加对IService的引用,同时还要添加对System.ServiceModel的引用,代码如下:
using System; using System.ServiceModel; using IService; namespace WCFClient { class Program { static void Main(string[] args) { using(ChannelFactory<ICalculator> channelFactory = new ChannelFactory<ICalculator>("calculatorservice")) { ICalculator proxy = channelFactory.CreateChannel(); Console.WriteLine("3 + 1 = {0}", proxy.Add(3, 1)); } Console.Read(); } } }
App.config文件的代码如下:
<?xml version="1.0" encoding="utf-8" ?> <configuration> <system.serviceModel> <client> <endpoint name="calculatorservice" address="http://127.0.0.1:3721/calculatorservice" binding="wsHttpBinding" contract="IService.ICalculator" /> </client> </system.serviceModel> </configuration>
配置文件配的是调用控制台宿主的WCF服务,如果要调用IIS的WCF就把黄色部分的代码改为:http://127.0.0.1:8024/CalculatorService.svc,Console的端口号是在ConsoleHost项目下的App.config中配置的,而IIS的端口号是搭建Web的时候,配置的。
运行结果如下:
WCF全面解析
amy
2014年09月16日
amy
2014年09月16日