给定一个二叉树,判断它是否是高度平衡的二叉树。
本题中,一棵高度平衡二叉树定义为:
一个二叉树每个节点 的左右两个子树的高度差的绝对值不超过 1 。
示例 1:
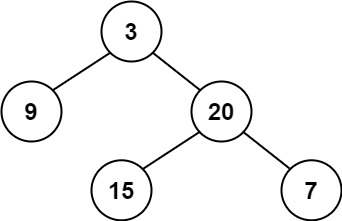
输入:root = [3,9,20,null,null,15,7] 输出:true
示例 2:
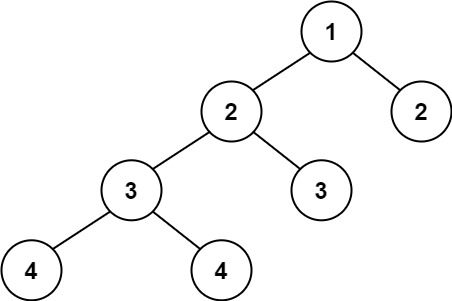
输入:root = [1,2,2,3,3,null,null,4,4] 输出:false
示例 3:
输入:root = [] 输出:true
提示:
- 树中的节点数在范围
[0, 5000]
内 -104 <= Node.val <= 104
C#代码
/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
public bool IsBalanced(TreeNode root) {
if(root == null) return true;
if(Math.Abs(MaxDepth(root.left) - MaxDepth(root.right)) > 1) return false;
return IsBalanced(root.left) && IsBalanced(root.right);
}
private Dictionary<TreeNode,int> dic = new Dictionary<TreeNode,int>();
public int MaxDepth(TreeNode root){
if(root == null) return 0;
if(dic.ContainsKey(root)) return dic[root];
int depth = Math.Max(MaxDepth(root.left), MaxDepth(root.right)) + 1;
dic.Add(root,depth);
return depth;
}
}