1、系统方式
- 将要删除的数据添加到待删数组中,从数据源中删除待删数组中包含的数据,刷新表格。
- OC 中可设置编辑模式为 UITableViewCellEditingStyleDelete | UITableViewCellEditingStyleInsert; 或者设置 myTableView.allowsMultipleSelectionDuringEditing = YES; 进入多选模式。
-
1.1 待删数据数组初始化
// 声明待删数据数组
@property(nonatomic, retain)NSMutableArray *tempDeleteArray;
// 初始化待删数据数组
tempDeleteArray = [[NSMutableArray alloc] init];
// 编辑按钮点击响应事件
- (void)editClick:(UIButton *)button {
// 改变编辑开关状态
[myTableView setEditing:!myTableView.editing animated:YES];
// 设置编辑模式,允许编辑时多选,或者在协议方法中设置
myTableView.allowsMultipleSelectionDuringEditing = YES;
// 当编辑状态发生改变的时候,清空待删数组
[tempDeleteArray removeAllObjects];
[myTableView reloadData];
}
// 删除按钮点击响应事件
- (void)deleteClick:(UIButton *)button {
// 从数据源中删除待选数组中包含的数据
[myDataArray removeObjectsInArray:tempDeleteArray];
// 清空待删数组
[tempDeleteArray removeAllObjects];
[myTableView reloadData];
}
// 设置编辑模式
/*
删除、插入、多选删除,不设置默认时为删除,
或者在编辑按钮点击事件中直接设置 myTableView.allowsMultipleSelectionDuringEditing = YES;
*/
- (UITableViewCellEditingStyle)tableView:(UITableView *)tableView editingStyleForRowAtIndexPath:(NSIndexPath *)indexPath {
// 多选删除
return UITableViewCellEditingStyleDelete | UITableViewCellEditingStyleInsert;
}
// 表格选中点击响应事件
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath {
// 判断 tableView 的编辑状态,表格处于编辑状态
if (tableView.isEditing) {
// 选中 cell 的时候,将对应的数据源模型添加到待删除数组中
[tempDeleteArray addObject:[myDataArray objectAtIndex:indexPath.row]];
}
else {
// 恢复未选中状态时的颜色
[tableView deselectRowAtIndexPath:indexPath animated:YES];
}
}
// 表格取消选中点击响应事件
- (void)tableView:(UITableView *)tableView didDeselectRowAtIndexPath:(NSIndexPath *)indexPath {
// 判断 tableView 的编辑状态,表格处于编辑状态
if (tableView.isEditing) {
// 将对应的数据模型从待删除数组中移除
[tempDeleteArray removeObject:[myDataArray objectAtIndex:indexPath.row]];
}
}
-
1.4 运行效果
------ 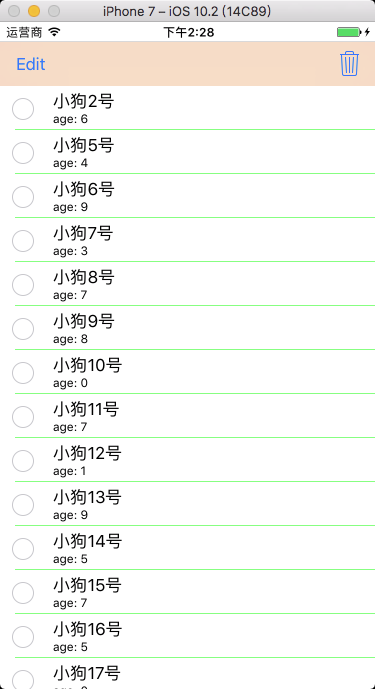
2、自定义方式 1
#import <Foundation/Foundation.h>
@interface XMGDeal : NSObject
@property (strong, nonatomic) NSString *buyCount;
@property (strong, nonatomic) NSString *price;
@property (strong, nonatomic) NSString *title;
@property (strong, nonatomic) NSString *icon;
/** 状态量标识有无被打钩 */
@property (assign, nonatomic, getter=isChecked) BOOL checked;
+ (instancetype)dealWithDict:(NSDictionary *)dict;
@end
#import "XMGDeal.h"
@implementation XMGDeal
+ (instancetype)dealWithDict:(NSDictionary *)dict {
XMGDeal *deal = [[self alloc] init];
[deal setValuesForKeysWithDictionary:dict];
return deal;
}
@end
-
2.3 XMGDealCell.xib
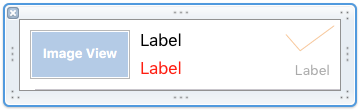
-
2.4 XMGDealCell.h
#import <UIKit/UIKit.h>
@class XMGDeal;
@interface XMGDealCell : UITableViewCell
/** 团购模型数据 */
@property (nonatomic, strong) XMGDeal *deal;
+ (instancetype)cellWithTableView:(UITableView *)tableView;
@end
#import "XMGDealCell.h"
#import "XMGDeal.h"
@interface XMGDealCell()
@property (weak, nonatomic) IBOutlet UIImageView *iconView;
@property (weak, nonatomic) IBOutlet UILabel *titleLabel;
@property (weak, nonatomic) IBOutlet UILabel *buyCountLabel;
@property (weak, nonatomic) IBOutlet UIImageView *checkView;
@property (weak, nonatomic) IBOutlet UILabel *priceLabel;
@end
@implementation XMGDealCell
+ (instancetype)cellWithTableView:(UITableView *)tableView {
static NSString *ID = @"deal";
XMGDealCell *cell = [tableView dequeueReusableCellWithIdentifier:ID];
if (cell == nil) {
cell = [[[NSBundle mainBundle] loadNibNamed:NSStringFromClass([XMGDealCell class])
owner:nil
options:nil] lastObject];
}
return cell;
}
- (void)setDeal:(XMGDeal *)deal {
_deal = deal;
// 设置数据
self.iconView.image = [UIImage imageNamed:deal.icon];
self.titleLabel.text = deal.title;
self.priceLabel.text = [NSString stringWithFormat:@"¥%@", deal.price];
self.buyCountLabel.text = [NSString stringWithFormat:@"%@人已购买", deal.buyCount];
// 设置打钩控件的显示和隐藏
self.checkView.hidden = !deal.isChecked;
}
@end
-
2.6 XMGDealsViewController.m
#import "XMGDealsViewController.h"
#import "XMGDeal.h"
#import "XMGDealCell.h"
@interface XMGDealsViewController () <UITableViewDataSource, UITableViewDelegate>
@property (weak, nonatomic) IBOutlet UITableView *tableView;
/** 所有的团购数据 */
@property (nonatomic, strong) NSMutableArray *deals;
@end
@implementation XMGDealsViewController
- (NSMutableArray *)deals {
if (_deals == nil) {
// 加载plist中的字典数组
NSString *path = [[NSBundle mainBundle] pathForResource:@"deals.plist" ofType:nil];
NSArray *dictArray = [NSArray arrayWithContentsOfFile:path];
// 字典数组 -> 模型数组
NSMutableArray *dealArray = [NSMutableArray array];
for (NSDictionary *dict in dictArray) {
XMGDeal *deal = [XMGDeal dealWithDict:dict];
[dealArray addObject:deal];
}
_deals = dealArray;
}
return _deals;
}
- (IBAction)remove {
// 临时数组:存放即将需要删除的团购数据
NSMutableArray *deletedDeals = [NSMutableArray array];
for (XMGDeal *deal in self.deals) {
if (deal.isChecked) [deletedDeals addObject:deal];
}
// 删除模型数据
[self.deals removeObjectsInArray:deletedDeals];
// 刷新表格
[self.tableView reloadData];
}
#pragma mark - Table view data source
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
return self.deals.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
XMGDealCell *cell = [XMGDealCell cellWithTableView:tableView];
// 取出模型数据
cell.deal = self.deals[indexPath.row];
return cell;
}
#pragma mark - TableView 代理方法
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath {
// 取消选中这一行
[tableView deselectRowAtIndexPath:indexPath animated:YES];
// 模型的打钩属性取反
XMGDeal *deal = self.deals[indexPath.row];
deal.checked = !deal.isChecked;
// 刷新表格
[tableView reloadData];
}
@end
-
2.7 运行效果
------ 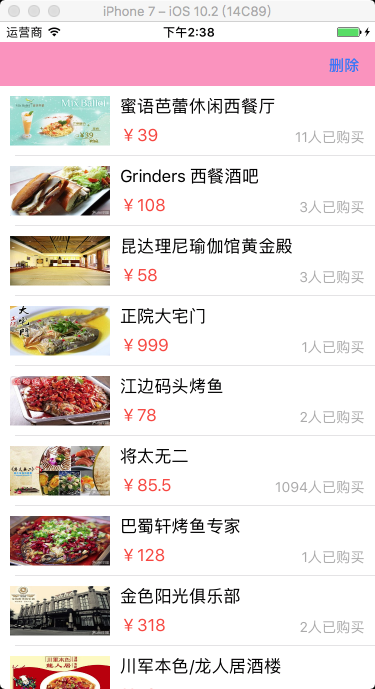
3、自定义方式 2
#import <Foundation/Foundation.h>
@interface XMGDeal : NSObject
@property (strong, nonatomic) NSString *buyCount;
@property (strong, nonatomic) NSString *price;
@property (strong, nonatomic) NSString *title;
@property (strong, nonatomic) NSString *icon;
+ (instancetype)dealWithDict:(NSDictionary *)dict;
@end
#import "XMGDeal.h"
@implementation XMGDeal
+ (instancetype)dealWithDict:(NSDictionary *)dict {
XMGDeal *deal = [[self alloc] init];
[deal setValuesForKeysWithDictionary:dict];
return deal;
}
@end
-
3.3 XMGDealCell.xib
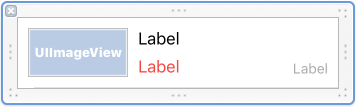
-
3.4 XMGDealCell.h
#import <UIKit/UIKit.h>
@class XMGDeal;
@interface XMGDealCell : UITableViewCell
/** 团购模型数据 */
@property (nonatomic, strong) XMGDeal *deal;
+ (instancetype)cellWithTableView:(UITableView *)tableView;
@end
#import "XMGDealCell.h"
#import "XMGDeal.h"
@interface XMGDealCell()
@property (weak, nonatomic) IBOutlet UIImageView *iconView;
@property (weak, nonatomic) IBOutlet UILabel *titleLabel;
@property (weak, nonatomic) IBOutlet UILabel *buyCountLabel;
@property (weak, nonatomic) IBOutlet UIImageView *checkView;
@property (weak, nonatomic) IBOutlet UILabel *priceLabel;
@end
@implementation XMGDealCell
+ (instancetype)cellWithTableView:(UITableView *)tableView {
static NSString *ID = @"deal";
XMGDealCell *cell = [tableView dequeueReusableCellWithIdentifier:ID];
if (cell == nil) {
cell = [[[NSBundle mainBundle] loadNibNamed:NSStringFromClass([XMGDealCell class])
owner:nil
options:nil] lastObject];
}
return cell;
}
- (void)setDeal:(XMGDeal *)deal {
_deal = deal;
// 设置数据
self.iconView.image = [UIImage imageNamed:deal.icon];
self.titleLabel.text = deal.title;
self.priceLabel.text = [NSString stringWithFormat:@"¥%@", deal.price];
self.buyCountLabel.text = [NSString stringWithFormat:@"%@人已购买", deal.buyCount];
}
@end
-
3.6 XMGDealsViewController.m
#import "XMGDealsViewController.h"
#import "XMGDeal.h"
#import "XMGDealCell.h"
@interface XMGDealsViewController () <UITableViewDataSource, UITableViewDelegate>
@property (weak, nonatomic) IBOutlet UITableView *tableView;
/** 所有的团购数据 */
@property (nonatomic, strong) NSMutableArray *deals;
/** 即将要删除的团购 */
@property (nonatomic, strong) NSMutableArray *deletedDeals;
@end
@implementation XMGDealsViewController
- (NSMutableArray *)deletedDeals {
if (!_deletedDeals) {
_deletedDeals = [NSMutableArray array];
}
return _deletedDeals;
}
- (NSMutableArray *)deals {
if (_deals == nil) {
// 加载plist中的字典数组
NSString *path = [[NSBundle mainBundle] pathForResource:@"deals.plist" ofType:nil];
NSArray *dictArray = [NSArray arrayWithContentsOfFile:path];
// 字典数组 -> 模型数组
NSMutableArray *dealArray = [NSMutableArray array];
for (NSDictionary *dict in dictArray) {
XMGDeal *deal = [XMGDeal dealWithDict:dict];
[dealArray addObject:deal];
}
_deals = dealArray;
}
return _deals;
}
- (IBAction)remove {
// 删除模型数据
[self.deals removeObjectsInArray:self.deletedDeals];
// 刷新表格
[self.tableView reloadData];
// 清空数组
[self.deletedDeals removeAllObjects];
}
#pragma mark - Table view data source
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
return self.deals.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
XMGDealCell *cell = [XMGDealCell cellWithTableView:tableView];
// 取出模型数据
cell.deal = self.deals[indexPath.row];
cell.checkView.hidden = ![self.deletedDeals containsObject:cell.deal];
return cell;
}
#pragma mark - TableView代理方法
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath {
// 取消选中这一行
[tableView deselectRowAtIndexPath:indexPath animated:YES];
// 取出模型
XMGDeal *deal = self.deals[indexPath.row];
if ([self.deletedDeals containsObject:deal]) {
[self.deletedDeals removeObject:deal];
} else {
[self.deletedDeals addObject:deal];
}
// 刷新表格
[tableView reloadData];
}
@end
-
3.7 运行效果
------ 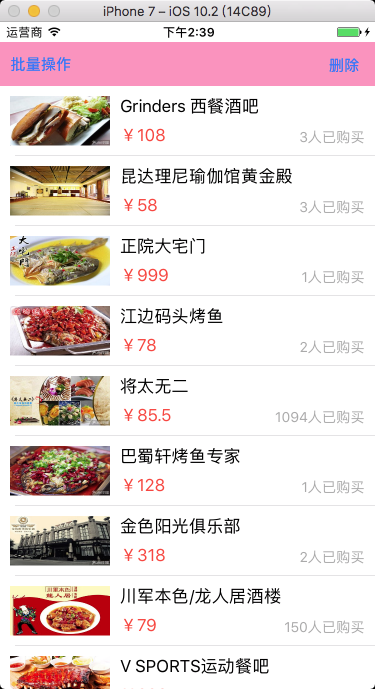