You are looking at the floor plan of the Summer Informatics School's new building. You were tasked with SIS logistics, so you really care about travel time between different locations: it is important to know how long it would take to get from the lecture room to the canteen, or from the gym to the server room.
The building consists of n towers, h floors each, where the towers are labeled from 1 to n, the floors are labeled from 1 to h. There is a passage between any two adjacent towers (two towers i and i + 1 for all i: 1 ≤ i ≤ n - 1) on every floor x, where a ≤ x ≤ b. It takes exactly one minute to walk between any two adjacent floors of a tower, as well as between any two adjacent towers, provided that there is a passage on that floor. It is not permitted to leave the building.
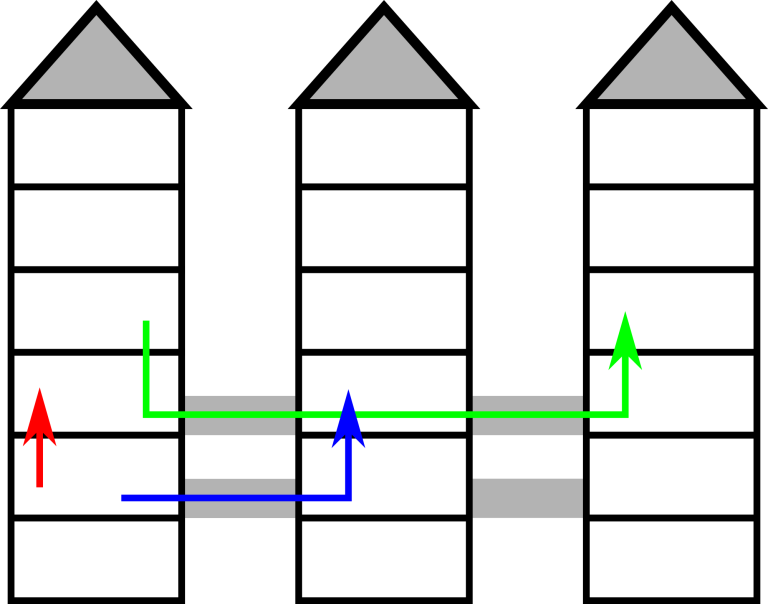
The picture illustrates the first example.
You have given k pairs of locations (ta, fa), (tb, fb): floor fa of tower ta and floor fb of tower tb. For each pair you need to determine the minimum walking time between these locations.
The first line of the input contains following integers:
- n: the number of towers in the building (1 ≤ n ≤ 108),
- h: the number of floors in each tower (1 ≤ h ≤ 108),
- a and b: the lowest and highest floor where it's possible to move between adjacent towers (1 ≤ a ≤ b ≤ h),
- k: total number of queries (1 ≤ k ≤ 104).
Next k lines contain description of the queries. Each description consists of four integers ta, fa, tb, fb (1 ≤ ta, tb ≤ n, 1 ≤ fa, fb ≤ h). This corresponds to a query to find the minimum travel time between fa-th floor of the ta-th tower and fb-th floor of the tb-th tower.
For each query print a single integer: the minimum walking time between the locations in minutes.
3 6 2 3 3
1 2 1 3
1 4 3 4
1 2 2 3
1
4
2
询问从t1的f1层到t2的f2层的最短时间,注意特判下在同一座塔的情况。
1 #include<iostream>
2 #include<cstring>
3 #include<cstdio>
4 #include<map>
5 #include<set>
6 #include<queue>
7 #include<vector>
8 #include<algorithm>
9 #include<cmath>
10 #include<deque>
11 #include<list>
12 using namespace std;
13 #define LL long long
14 #define PI acos(-1.0)
15 #define inf 0x3f3f3f3f
16 #define pb push_back
17 #define mp make_pair
18 int main()
19 {
20 int n,h,a,b,k,i,j;
21 int t1,f1,t2,f2;
22 while(scanf("%d%d%d%d%d",&n,&h,&a,&b,&k)!=EOF){
23 while(k--){
24 scanf("%d%d%d%d",&t1,&f1,&t2,&f2);
25 if(t1>t2){
26 swap(t1,t2),swap(f1,f2);
27 }
28 if(t1==t2){
29 cout<<abs(f1-f2)<<endl;
30 }
31 else{
32 if(f1>=a&&f1<=b){
33 cout<<t2-t1+abs(f1-f2)<<endl;
34 }
35 else{
36 cout<<min(abs(a-f1)+t2-t1+abs(a-f2),
37 abs(b-f1)+t2-t1+abs(b-f2))<<endl;
38 }
39 }
40 }
41 }
42 return 0;
43 }
In Summer Informatics School, if a student doesn't behave well, teachers make a hole in his badge. And today one of the teachers caught a group of nn students doing yet another trick.
Let's assume that all these students are numbered from 11 to nn. The teacher came to student aa and put a hole in his badge. The student, however, claimed that the main culprit is some other student papa.
After that, the teacher came to student papa and made a hole in his badge as well. The student in reply said that the main culprit was student ppappa.
This process went on for a while, but, since the number of students was finite, eventually the teacher came to the student, who already had a hole in his badge.
After that, the teacher put a second hole in the student's badge and decided that he is done with this process, and went to the sauna.
You don't know the first student who was caught by the teacher. However, you know all the numbers pipi. Your task is to find out for every student aa, who would be the student with two holes in the badge if the first caught student was aa.
The first line of the input contains the only integer nn (1≤n≤10001≤n≤1000) — the number of the naughty students.
The second line contains nn integers p1p1, ..., pnpn (1≤pi≤n1≤pi≤n), where pipi indicates the student who was reported to the teacher by student ii.
For every student aa from 11 to nn print which student would receive two holes in the badge, if aa was the first student caught by the teacher.
3
2 3 2
2 2 3
3
1 2 3
1 2 3
The picture corresponds to the first example test case.

When a=1a=1, the teacher comes to students 11, 22, 33, 22, in this order, and the student 22 is the one who receives a second hole in his badge.
When a=2a=2, the teacher comes to students 22, 33, 22, and the student 22 gets a second hole in his badge. When a=3a=3, the teacher will visit students 33, 22, 33 with student 33 getting a second hole in his badge.
For the second example test case it's clear that no matter with whom the teacher starts, that student would be the one who gets the second hole in his badge.
直接模拟,找第一个被访问两次的点就好了。
1 #include<iostream>
2 #include<cstring>
3 #include<cstdio>
4 #include<map>
5 #include<set>
6 #include<queue>
7 #include<vector>
8 #include<algorithm>
9 #include<cmath>
10 #include<deque>
11 #include<list>
12 using namespace std;
13 #define LL long long
14 #define PI acos(-1.0)
15 #define inf 0x3f3f3f3f
16 #define pb push_back
17 #define mp make_pair
18 bool vis[1100];
19 int main()
20 {
21 int n,m,i,j,k,p[1111];
22 while(scanf("%d",&n)==1){
23 for(i=1;i<=n;++i)scanf("%d",p+i);
24 for(i=1;i<=n;++i){
25 memset(vis,0,sizeof(vis));
26 j=i;
27 while(!vis[j]){
28 vis[j]=1;
29 j=p[j];
30 }
31 printf("%d%c",j,i==n?'
':' ');
32 }
33 }
34 return 0;
35 }
As you know, majority of students and teachers of Summer Informatics School live in Berland for the most part of the year. Since corruption there is quite widespread, the following story is not uncommon.
Elections are coming. You know the number of voters and the number of parties — nn and mm respectively. For each voter you know the party he is going to vote for. However, he can easily change his vote given a certain amount of money. In particular, if you give ii-th voter cicibytecoins you can ask him to vote for any other party you choose.
The United Party of Berland has decided to perform a statistical study — you need to calculate the minimum number of bytecoins the Party needs to spend to ensure its victory. In order for a party to win the elections, it needs to receive strictly more votes than any other party.
The first line of input contains two integers nn and mm (1≤n,m≤30001≤n,m≤3000) — the number of voters and the number of parties respectively.
Each of the following nn lines contains two integers pipi and cici (1≤pi≤m1≤pi≤m, 1≤ci≤1091≤ci≤109) — the index of this voter's preferred party and the number of bytecoins needed for him to reconsider his decision.
The United Party of Berland has the index 11.
Print a single number — the minimum number of bytecoins needed for The United Party of Berland to win the elections.
1 2
1 100
0
5 5
2 100
3 200
4 300
5 400
5 900
500
5 5
2 100
3 200
4 300
5 800
5 900
600
In the first sample, The United Party wins the elections even without buying extra votes.
In the second sample, The United Party can buy the votes of the first and the fourth voter. This way The Party gets two votes, while parties 33, 44 and 55 get one vote and party number 22 gets no votes.
In the third sample, The United Party can buy the votes of the first three voters and win, getting three votes against two votes of the fifth party.
考虑枚举1的最终得票数,然后计算是否能当选以及最小的费用,选取一个最小的作为答案。
1 #include<iostream> 2 #include<cstring> 3 #include<cstdio> 4 #include<map> 5 #include<set> 6 #include<queue> 7 #include<vector> 8 #include<algorithm> 9 #include<cmath> 10 #include<deque> 11 #include<list> 12 #include<bits/stdc++.h> 13 using namespace std; 14 #define LL long long 15 #define PI acos(-1.0) 16 #define inf 0x3f3f3f3f 17 #define pb push_back 18 #define mp make_pair 19 int p[3333],c[3333],tot[3333],n,m; 20 LL ans; 21 priority_queue<int,vector<int>,greater<int> >q[3333]; 22 vector<LL> v[3333];38 int main() 39 { 40 int i,j; 41 scanf("%d%d",&n,&m); 42 for(i=1;i<=n;++i)scanf("%d%d",p+i,c+i),tot[p[i]]++,q[p[i]].push(c[i]); 43 for(i=1;i<=m;++i){ 44 v[i].push_back(0); 45 while(!q[i].empty()){ 46 v[i].push_back(*(v[i].end()-1)+q[i].top()); 47 q[i].pop(); 48 } 49 } 50 LL ans=1LL*1000000000000000000; 51 for(i=tot[1];i<=n;++i){ 52 LL res=0,buy=0; 53 for(j=2;j<=m;++j){ 54 if(tot[j]>=i){ 55 buy+=tot[j]-i+1; 56 res+=v[j][tot[j]-i+1]; 57 } 58 } 59 if(buy>i-tot[1]) continue; 60 //q[0].clear(); 61 while(!q[0].empty())q[0].pop(); 62 //cout<<"i="<<i<<' '<<res<<' '<<buy<<endl; 63 for(int ii=2;ii<=m;++ii){ 64 j=1; 65 if(tot[ii]>=i) j=tot[ii]-i+1+1; 66 for(;j<v[ii].size();++j){ 67 q[0].push(v[ii][j]-v[ii][j-1]); 68 } 69 } 70 while(!q[0].empty()&&buy<i-tot[1]){ 71 //cout<<"JI"<<q[0].top()<<endl; 72 res+=q[0].top(); 73 q[0].pop(); 74 buy++; 75 } 76 if(tot[1]+buy<i)continue; 77 //cout<<i<<' '<<res<<endl; 78 if(res<ans)ans=res; 79 } 80 cout<<ans<<endl; 81 return 0; 82 }