项目中,在微服务内部通信使用Grpc来实现。Grpc和WebApi相比有以下特点:基于Http/2,
支持C++,Python,Ruby,Golang,java,nodejs,C# 等主流开发语言,Protobuf的格式比Json在数据传输上的性能更高。
下面介绍Grpc的使用:
服务端 :
(1).创建服务端,新建Grpc服务MicService.GrpcServer
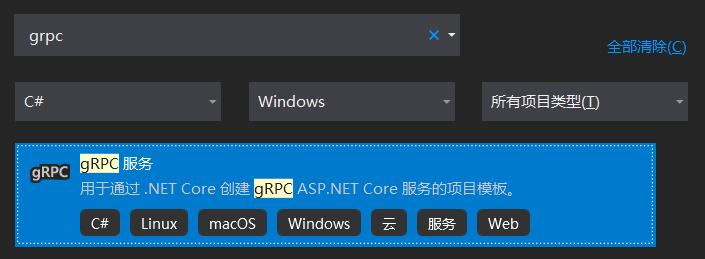
默认会生成以下几个文件 greet.proto和GreeterService
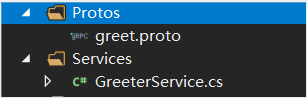
greet.proto内容如下:
1 syntax = "proto3"; 2 3 option csharp_namespace = "MicService.GrpcServer"; 4 5 package greet; 6 7 // The greeting service definition. 8 service Greeter { 9 // Sends a greeting 10 rpc SayHello (HelloRequest) returns (HelloReply); 11 } 12 13 // The request message containing the user's name. 14 message HelloRequest { 15 string name = 1; 16 } 17 18 // The response message containing the greetings. 19 message HelloReply { 20 string message = 1; 21 }
GreeterService.cs内容如下:
1 public class GreeterService : Greeter.GreeterBase 2 { 3 private readonly ILogger<GreeterService> _logger; 4 public GreeterService(ILogger<GreeterService> logger) 5 { 6 _logger = logger; 7 } 8 9 public override Task<HelloReply> SayHello(HelloRequest request, ServerCallContext context) 10 { 11 return Task.FromResult(new HelloReply 12 { 13 Message = "Hello " + request.Name 14 }); 15 } 16 }
(2)Startup配置
可以看到在“ConfigureServices.cs”中增加了services.AddGrpc();
public void ConfigureServices(IServiceCollection services) { services.AddGrpc(); }
在Configure中添加EndPoint
1 public void Configure(IApplicationBuilder app, IWebHostEnvironment env) 2 { 3 if (env.IsDevelopment()) 4 { 5 app.UseDeveloperExceptionPage(); 6 } 7 8 app.UseRouting(); 9 10 app.UseEndpoints(endpoints => 11 { 12 endpoints.MapGrpcService<GreeterService>(); 13 14 endpoints.MapGet("/", async context => 15 { 16 await context.Response.WriteAsync("Communication with gRPC endpoints must be made through a gRPC client. To learn how to create a client, visit: https://go.microsoft.com/fwlink/?linkid=2086909"); 17 }); 18 }); 19 }
(3)项目文件配置:
注意:GrpcServices服务端为Server
<ItemGroup> <Protobuf Include="Protosgreet.proto" GrpcServices="Server" /> </ItemGroup>
客户端:
这里创建一个控制台程序MicService.GrpcClient
(1)引入三个Nuget包
Google.Protobuf
Grpc.Net.Client
Grpc.Tools
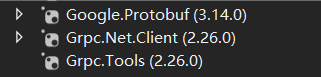
(2)将服务端的Proto文件拷贝至项目中,并修改项目文件配置
注意:GrpcServices客户端为Client
<ItemGroup> <Protobuf Include="Protosgreet.proto" GrpcServices="Client" /> </ItemGroup>
(3)和服务端通信
1 //创建通道 基于grpc地址 2 using var channel = GrpcChannel.ForAddress("https://localhost:5001"); 3 4 //创建客户端代理 5 var client = new GreeterClient(channel); 6 7 //服务端调用 8 var reply = await client.SayHelloAsync(new HelloRequest { Name = "客户端传参" }); 9 10 ////客户端等待返回结果 11 Console.WriteLine(reply.Message); 12 13 Console.ReadKey();
开始测试:
配置多个项目启动项,其中服务端要优先于客户端
结果如下:
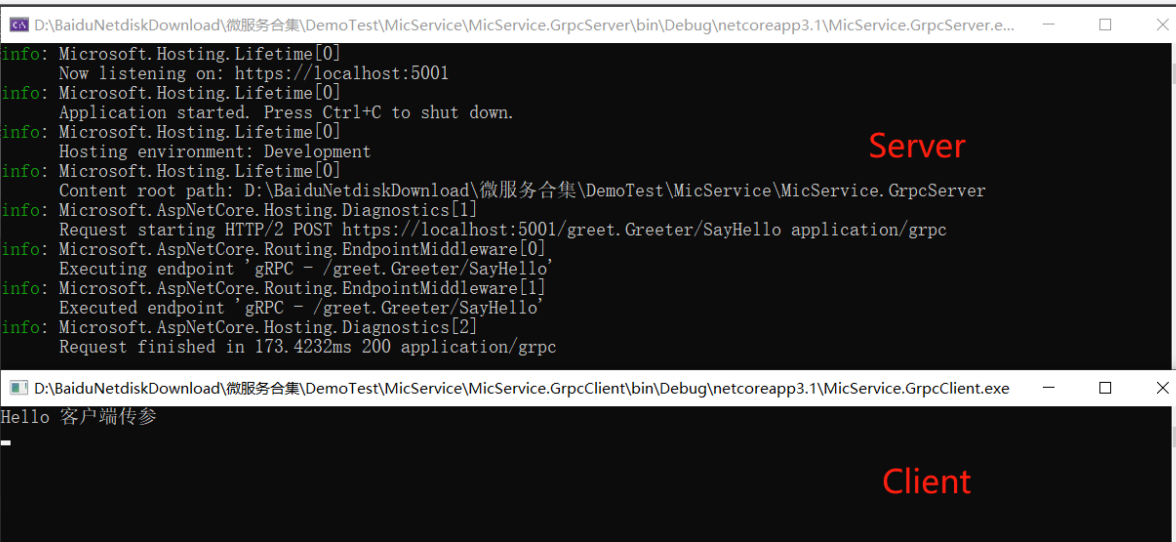
注:可能会发生的错误
不能创建SSL连接
Grpc.Core.RpcException:“Status(StatusCode="Unavailable", Detail="Error starting gRPC call. HttpRequestException: An error occurred while sending the request. IOException: The response ended prematurely.",
解决方案:在客户端设置允许不安全的HTTP2支持
AppContext.SetSwitch("System.Net.Http.SocketsHttpHandler.Http2UnencryptedSupport", true);
附源码:
链接:https://pan.baidu.com/s/19gGkpevQH-EJaJJ1XTT5sg
提取码:h18f
以上,仅用于学习和总结!