在google地图上画出两个地点的行走路线
Google Direction API提供了更丰富的路线信息数据。由于访问量过大吧,谷歌现在不提供了查询路线的接口API,现在要想获得一个路线我们可以通过URL访问google然后解析得到的KML文件,得到路线的每个点的经纬度,然互在一个图层上画出这些点。代码来自于对一些网上资源的整理,一共提供了连个方法,一个通过两个地方的名字,一个是通过两个地点的经纬度,但是有时候会出现错误的别是对中国的城市,还有就是别用中文,要不会很惨,希望有人给出一个更好的方法
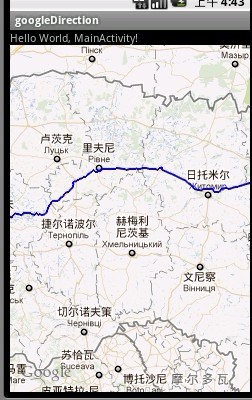
[代码] 新建一个图层
01 |
package com.android.antkingwei.google.direction; |
03 |
import android.graphics.Canvas; |
04 |
import android.graphics.Color; |
05 |
import android.graphics.Paint; |
06 |
import android.graphics.Point; |
08 |
import com.google.android.maps.GeoPoint; |
09 |
import com.google.android.maps.MapView; |
10 |
import com.google.android.maps.Overlay; |
11 |
import com.google.android.maps.Projection; |
13 |
public class DirectionPathOverlay extends Overlay { |
17 |
public DirectionPathOverlay(GeoPoint gp1, GeoPoint gp2) { |
23 |
public boolean draw(Canvas canvas, MapView mapView, boolean shadow, long when) { |
24 |
Projection projection = mapView.getProjection(); |
26 |
Paint paint = new Paint(); |
27 |
paint.setAntiAlias( true ); |
28 |
Point point = new Point(); |
29 |
projection.toPixels(gp1, point); |
30 |
paint.setColor(Color.BLUE); |
31 |
Point point2 = new Point(); |
32 |
projection.toPixels(gp2, point2); |
33 |
paint.setStrokeWidth( 2 ); |
34 |
canvas.drawLine(( float ) point.x, ( float ) point.y, ( float ) point2.x, ( float ) point2.y, paint); |
36 |
return super .draw(canvas, mapView, shadow, when); |
40 |
public void draw(Canvas canvas, MapView mapView, boolean shadow) { |
41 |
super .draw(canvas, mapView, shadow); |
[代码] 主类
001 |
package com.android.antkingwei.google.direction; |
003 |
import java.net.HttpURLConnection; |
006 |
import javax.xml.parsers.DocumentBuilder; |
007 |
import javax.xml.parsers.DocumentBuilderFactory; |
009 |
import org.w3c.dom.Document; |
010 |
import org.w3c.dom.Node; |
011 |
import org.w3c.dom.NodeList; |
013 |
import com.google.android.maps.GeoPoint; |
014 |
import com.google.android.maps.MapActivity; |
015 |
import com.google.android.maps.MapController; |
016 |
import com.google.android.maps.MapView; |
018 |
import android.app.Activity; |
019 |
import android.os.Bundle; |
020 |
import android.util.Log; |
022 |
public class MainActivity extends MapActivity { |
023 |
private MapView myMapView; |
024 |
private GeoPoint geoPoint; |
025 |
private MapController myMC; |
026 |
private String[] pairs; |
027 |
/** Called when the activity is first created. */ |
029 |
public void onCreate(Bundle savedInstanceState) { |
030 |
super .onCreate(savedInstanceState); |
031 |
setContentView(R.layout.main); |
033 |
myMapView = (MapView) findViewById(R.id.mapview); |
035 |
myMapView.setSatellite( false ); |
039 |
pairs = getUrl( 22.5348 , 113.97246 , 35.422006 , 119.524095 ); |
040 |
String[] lngLat = pairs[ 0 ].split( "," ); |
042 |
GeoPoint startGP = new GeoPoint(( int )(Double.parseDouble(lngLat[ 1 ]) * 1E6), ( int ) (Double.parseDouble(lngLat[ 0 ]) * 1E6)); |
043 |
myMC = myMapView.getController(); |
045 |
myMC.setCenter(geoPoint); |
047 |
myMapView.getOverlays().add( new DirectionPathOverlay(startGP, startGP)); |
050 |
GeoPoint gp2 = startGP; |
051 |
for ( int i = 1 ; i < pairs.length; i++) { |
052 |
lngLat = pairs[i].split( "," ); |
055 |
gp2 = new GeoPoint(( int ) (Double.parseDouble(lngLat[ 1 ]) * 1E6),( int ) (Double.parseDouble(lngLat[ 0 ]) * 1E6)); |
056 |
myMapView.getOverlays().add( new DirectionPathOverlay(gp1, gp2)); |
057 |
Log.d( "xxx" , "pair:" + pairs[i]); |
060 |
myMapView.getOverlays().add( new DirectionPathOverlay(gp2, gp2)); |
061 |
myMapView.getController().animateTo(startGP); |
062 |
myMapView.setBuiltInZoomControls( true ); |
063 |
myMapView.displayZoomControls( true ); |
067 |
protected boolean isRouteDisplayed() { |
072 |
* 通过四个坐标点,获得KML然后通过DOM解析 |
079 |
public String[] getUrl( double fromLat, double fromLon, double toLat, double toLon){ |
080 |
String urlString = "http://maps.google.com/maps?f=d&hl=en&saddr=" +fromLat+ "," +fromLon+ "&daddr=" +toLat+ "," +toLon+ "&ie=UTF8&0&om=0&output=kml" ; |
081 |
Log.d( "URL" , urlString); |
083 |
HttpURLConnection urlConnection = null ; |
085 |
String pathConent = "" ; |
088 |
url = new URL(urlString.toString()); |
089 |
urlConnection = (HttpURLConnection) url.openConnection(); |
090 |
urlConnection.setRequestMethod( "GET" ); |
091 |
urlConnection.setDoOutput( true ); |
092 |
urlConnection.setDoInput( true ); |
093 |
urlConnection.connect(); |
094 |
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance(); |
095 |
DocumentBuilder db = dbf.newDocumentBuilder(); |
096 |
doc = db.parse(urlConnection.getInputStream()); |
097 |
} catch (Exception e){} |
098 |
NodeList nl = doc.getElementsByTagName( "LineString" ); |
099 |
for ( int s= 0 ; s< nl.getLength(); s++){ |
100 |
Node rootNode = nl.item(s); |
101 |
NodeList configItems = rootNode.getChildNodes(); |
102 |
for ( int x= 0 ; x < configItems.getLength(); x++) { |
103 |
Node lineStringNode = configItems.item(x); |
104 |
NodeList path = lineStringNode.getChildNodes(); |
105 |
pathConent = path.item( 0 ).getNodeValue(); |
108 |
String[] tempContent = pathConent.split( " " ); |
112 |
* 通过连个地名获得KML然后进行DOM解析 |
117 |
private String[] getDirectionData(String srcPlace, String destPlace) { |
118 |
String urlString = "http://maps.google.com/maps?f=d&hl=en&saddr=" +srcPlace+ "&daddr=" +destPlace+ "&ie=UTF&om=0&output=kml" ; |
119 |
Log.d( "URL" , urlString); |
121 |
HttpURLConnection urlConnection = null ; |
123 |
String pathConent = "" ; |
125 |
url = new URL(urlString.toString()); |
126 |
urlConnection = (HttpURLConnection) url.openConnection(); |
127 |
urlConnection.setRequestMethod( "GET" ); |
128 |
urlConnection.setDoOutput( true ); |
129 |
urlConnection.setDoInput( true ); |
130 |
urlConnection.connect(); |
131 |
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance(); |
132 |
DocumentBuilder db = dbf.newDocumentBuilder(); |
133 |
doc = db.parse(urlConnection.getInputStream()); |
134 |
} catch (Exception e){} |
135 |
NodeList nl = doc.getElementsByTagName( "LineString" ); |
136 |
for ( int s= 0 ; s< nl.getLength(); s++){ |
137 |
Node rootNode = nl.item(s); |
138 |
NodeList configItems = rootNode.getChildNodes(); |
139 |
for ( int x= 0 ; x < configItems.getLength(); x++) { |
140 |
Node lineStringNode = configItems.item(x); |
141 |
NodeList path = lineStringNode.getChildNodes(); |
142 |
pathConent = path.item( 0 ).getNodeValue(); |
145 |
String[] tempContent = pathConent.split( " " ); |
[代码] main.xml
01 |
<? xml version = "1.0" encoding = "utf-8" ?> |
02 |
< LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android" |
03 |
android:orientation = "vertical" |
04 |
android:layout_width = "fill_parent" |
05 |
android:layout_height = "fill_parent" |
08 |
android:layout_width = "fill_parent" |
09 |
android:layout_height = "wrap_content" |
10 |
android:text = "@string/hello" |
12 |
< com.google.android.maps.MapView |
13 |
xmlns:android = "http://schemas.android.com/apk/res/android" |
14 |
android:id = "@+id/mapview" |
15 |
android:layout_width = "fill_parent" |
16 |
android:layout_height = "fill_parent" |
17 |
android:enabled = "true" |
18 |
android:clickable = "true" |
19 |
android:apiKey = "0FZLYf-YM4SRrJrJum55MeeaO4Gd_IitVFmtUeA" /> |
[代码] AndroidManifest.xml
01 |
<? xml version = "1.0" encoding = "utf-8" ?> |
02 |
< manifest xmlns:android = "http://schemas.android.com/apk/res/android" |
03 |
package = "com.android.antkingwei.google.direction" |
04 |
android:versionCode = "1" |
05 |
android:versionName = "1.0" > |
06 |
< uses-sdk android:minSdkVersion = "7" /> |
08 |
< application android:icon = "@drawable/icon" android:label = "@string/app_name" > |
09 |
< activity android:name = ".MainActivity" |
10 |
android:label = "@string/app_name" > |
12 |
< action android:name = "android.intent.action.MAIN" /> |
13 |
< category android:name = "android.intent.category.LAUNCHER" /> |
17 |
< uses-library android:name = "com.google.android.maps" /> |
19 |
< uses-permission android:name = "android.permission.INTERNET" ></ uses-permission > |
20 |
< uses-permission android:name = "android.permission.ACCESS_NETWORK_STATE" /> |
21 |
< uses-permission android:name = "android.permission.ACCESS_COARSE_LOCATION" /> |
22 |
< uses-permission android:name = "android.permission.ACCESS_FINE_LOCATION" /> |
一起学习GIS及其二次开发,一起进步!
原文地址:https://www.cnblogs.com/tuncaysanli/p/2469020.html