using System; using System.Data; using System.Configuration; using System.Web; using System.Web.Security; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Web.UI.HtmlControls; using System.Data.SqlClient; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } public void getData() { SqlConnection cn = new SqlConnection("server=localhost;database=Northwind;user=grid;password=grid"); SqlCommand cmd = new SqlCommand("select top 30 * from Customers", cn); SqlDataAdapter da = new SqlDataAdapter(cmd); DataSet ds = new DataSet(); da.Fill(ds, "Customers"); this.GridView1.DataSource = ds.Tables[0].DefaultView; this.DataBind(); } protected void Button1_Click(object sender, EventArgs e) { getData(); } protected void GridView1_PageIndexChanging(object sender, GridViewPageEventArgs e) { this.GridView1.PageIndex = e.NewPageIndex; getData(); } protected void GridView1_SelectedIndexChanging(object sender, GridViewSelectEventArgs e) { } protected void GridView1_RowDeleting(object sender, GridViewDeleteEventArgs e) { string CustomerID = this.GridView1.DataKeys[e.RowIndex][0].ToString(); deleteCustomers(CustomerID); getData(); } protected void GridView1_RowEditing(object sender, GridViewEditEventArgs e) { this.GridView1.EditIndex = e.NewEditIndex; getData(); } protected void GridView1_RowUpdating(object sender, GridViewUpdateEventArgs e) { string CustomerID = this.GridView1.DataKeys[e.RowIndex][0].ToString(); string CompanyName = ((TextBox)this.GridView1.Rows[e.RowIndex].Cells[0].Controls[0]).Text.ToString(); string ContactName = ((TextBox)this.GridView1.Rows[e.RowIndex].Cells[1].Controls[0]).Text.ToString(); string Address = ((TextBox)this.GridView1.Rows[e.RowIndex].Cells[2].Controls[0]).Text.ToString(); //Response.Write(Cus + "---" + CompanyName); updateCustomers(CustomerID, CompanyName, ContactName, Address); this.GridView1.EditIndex = -1; getData(); } protected void GridView1_RowCancelingEdit(object sender, GridViewCancelEditEventArgs e) { this.GridView1.EditIndex = -1; getData(); } public void updateCustomers(string CustomerID,string CompanyName,string ContactName,string Address) { SqlConnection cn = new SqlConnection("server=localhost;database=Northwind;user=grid;password=grid"); SqlCommand cmd = new SqlCommand("update Customers set CompanyName='" + CompanyName + "', ContactName='" + ContactName+"',Address='"+Address +"' where CustomerID='"+CustomerID +"'", cn); cn.Open(); cmd.ExecuteNonQuery(); cn.Close(); } public void deleteCustomers(string CustomerID) { SqlConnection cn = new SqlConnection("server=localhost;database=Northwind;user=grid;password=grid"); SqlCommand cmd = new SqlCommand("delete from Customers where CustomerID='" + CustomerID + "'", cn); cn.Open(); cmd.ExecuteNonQuery(); cn.Close(); } }
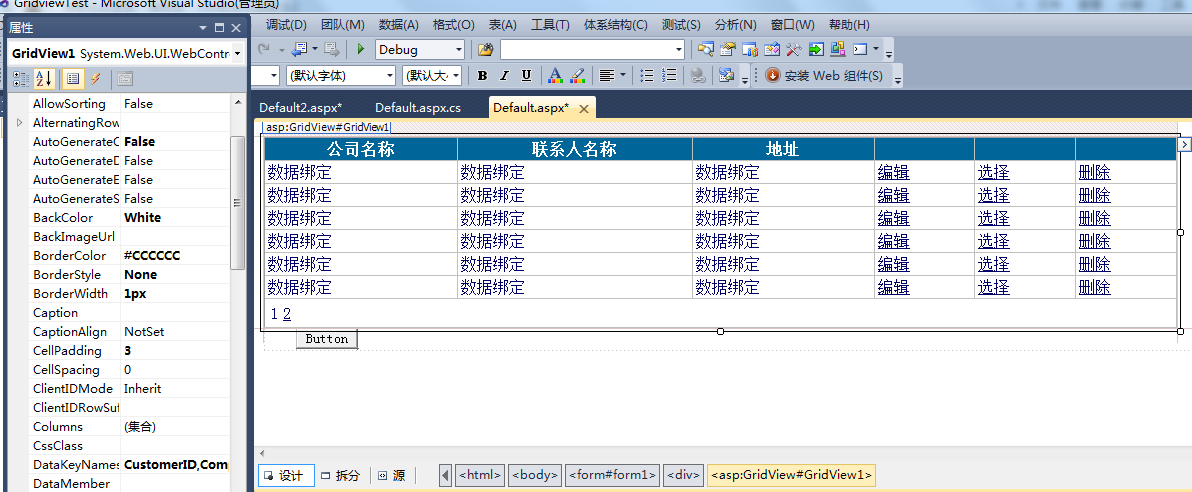
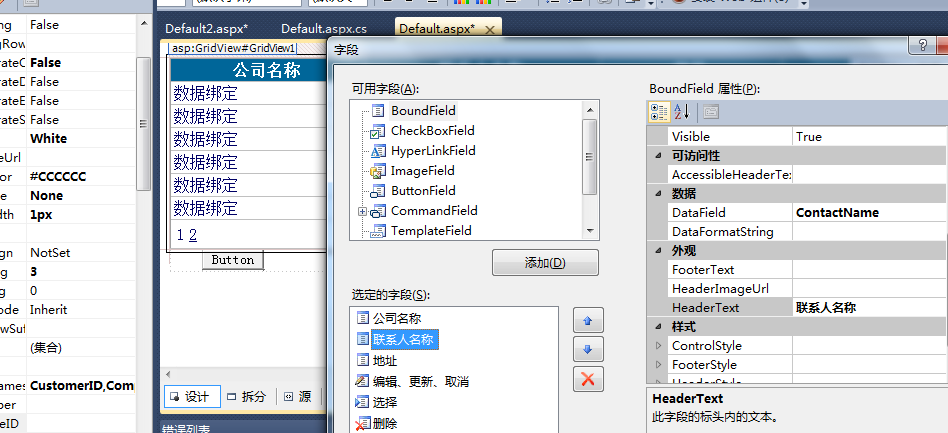
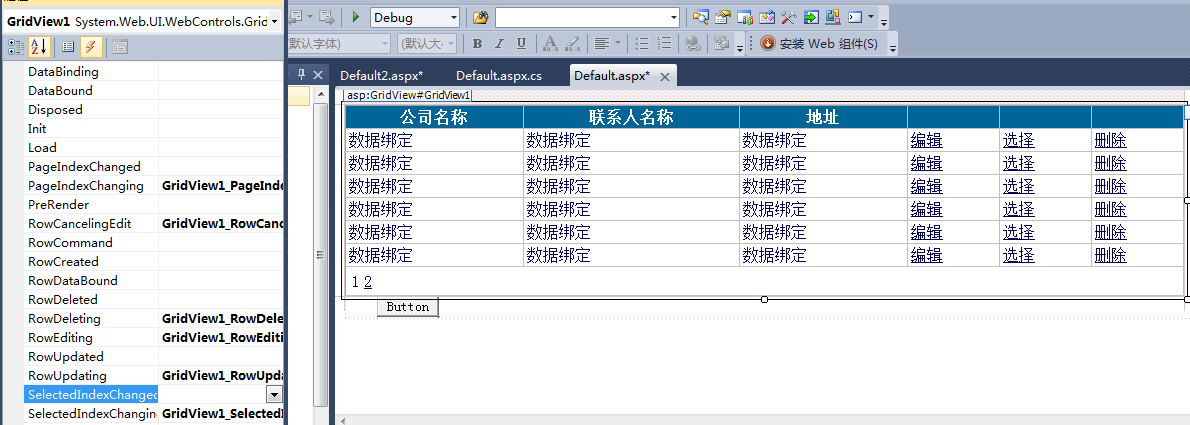
<asp:GridView ID="GridView1" runat="server" Width ="86%" BackColor="White" BorderColor="#CCCCCC" BorderStyle="None" BorderWidth="1px" CellPadding="3" AutoGenerateColumns="False" AllowPaging="True" OnPageIndexChanging="GridView1_PageIndexChanging" PageSize="6" DataKeyNames="CustomerID,CompanyName,ContactName,Address" OnRowCancelingEdit="GridView1_RowCancelingEdit" OnRowDeleting="GridView1_RowDeleting" OnRowEditing="GridView1_RowEditing" OnRowUpdating="GridView1_RowUpdating" OnSelectedIndexChanging="GridView1_SelectedIndexChanging" > <FooterStyle BackColor="White" ForeColor="#000066" /> <RowStyle ForeColor="#000066" /> <SelectedRowStyle BackColor="#669999" Font-Bold="True" ForeColor="White" /> <PagerStyle BackColor="White" ForeColor="#000066" HorizontalAlign="Left" /> <HeaderStyle BackColor="#006699" Font-Bold="True" ForeColor="White" /> <Columns> <asp:BoundField DataField="CompanyName" HeaderText="公司名称" /> <asp:BoundField DataField="ContactName" HeaderText="联系人名称" /> <asp:BoundField DataField="Address" HeaderText="地址" /> <asp:CommandField ShowEditButton="True" /> <asp:CommandField ShowSelectButton="True" /> <asp:CommandField ShowDeleteButton="True" /> </Columns> </asp:GridView>