结构型模式涉及到如何组合类和对象以获得更大的结构。结构型类模式采用继承机制来组合接口或实现。
Adapter模式:
适配器使得一个接口(adaptee的接口)与其他接口兼容,从而给出了多个不同接口的统一抽象。为此,类适配器对一个adaptee类进行私有继承。这样,适配器就可以用adaptee的接口标识它的接口。
在android编程的时候,写列表ListView时经常碰到Adapter,当时还不懂。。。。
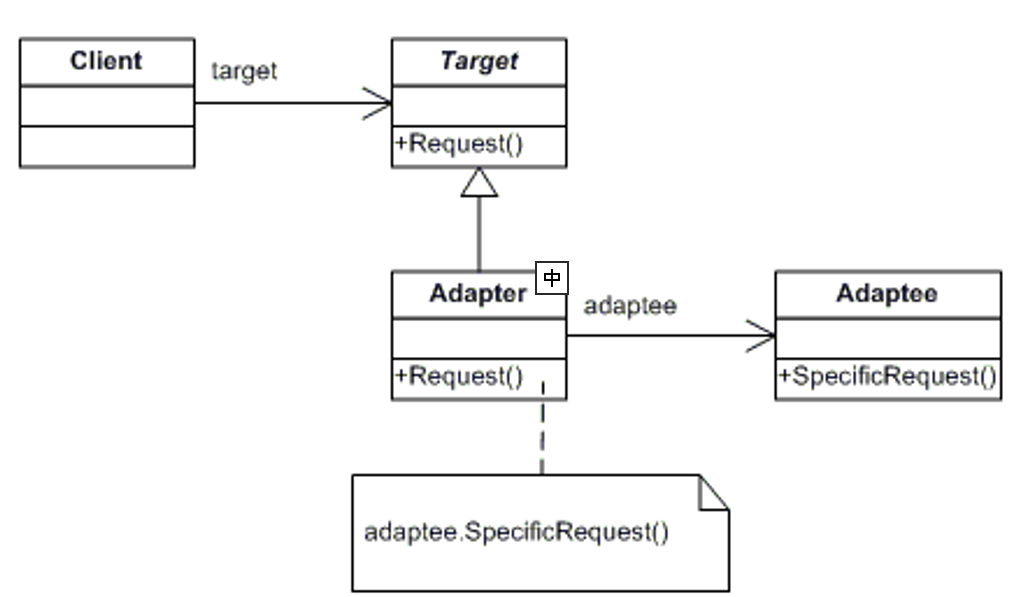
代码:
using System; namespace DoFactory.GangOfFour.Adapter.Structural { /// <summary> /// MainApp startup class for Structural /// Adapter Design Pattern. /// </summary> class MainApp { /// <summary> /// Entry point into console application. /// </summary> static void Main() { // Create adapter and place a request Target target = new Adapter(); target.Request(); // Wait for user Console.ReadKey(); } } /// <summary> /// The 'Target' class /// </summary> class Target { public virtual void Request() { Console.WriteLine("Called Target Request()"); } } /// <summary> /// The 'Adapter' class /// </summary> class Adapter : Target { private Adaptee _adaptee = new Adaptee(); public override void Request() { // Possibly do some other work // and then call SpecificRequest _adaptee.SpecificRequest(); } } /// <summary> /// The 'Adaptee' class /// </summary> class Adaptee { public void SpecificRequest() { Console.WriteLine("Called SpecificRequest()"); } } }
输出:
Called SpecificRequest()
2.Composite模式:
描述了如何构造一个类层次结构,这一结构由两种类型的对象(基元对象和组合对象)所对应的类构成。其中的组合对象使得你可以组合基元对象以及其他的组合对象,从而形成任意复杂的结构。
将对象组合成树形结构以表示“部分-整体”的层次结构。Composite使得用户对单个对象和组合对象的使用具有一致性。
代码:java实现,前边的都是C#
1)Client类
public class Client { public static void main(String[] args) { Leaf pLeaf1 = new Leaf(); Leaf pLeaf2 = new Leaf(); Composite composite = new Composite(); composite.Add(pLeaf1); composite.Add(pLeaf2); composite.Operation(); composite.GetChild(1).Operation(); } }
2)Component类
/** * 组件基类 * @author jyc * */ public abstract class Component { public Component(){ } public abstract void Operation(); public abstract void Add(Component pChild); public abstract void Remove(Component pChild); public abstract Component GetChild(int nIndex); }
3)Leaf类
//派生自Component,是其中的叶子组件的基类 public class Leaf extends Component { public Leaf(){ System.out.println("leaf构造方法 "); } public void Operation() { System.out.println("Operation by leaf "); } public void Add(Component pChild) { // TODO 自动生成的方法存根 } public void Remove(Component pChild) { // TODO 自动生成的方法存根 } public Component GetChild(int nIndex) { // TODO 自动生成的方法存根 return null; } }
4)Composite类
import java.util.ArrayList; import java.util.Collection; import java.util.Iterator; import java.util.List; import java.util.ListIterator; //派生自Component,是其中的含有子件的组件的基类 public class Composite extends Component { private List<Component> m_ListOfComponent;//集合 // 采用list容器去保存子组件 public Composite(){ m_ListOfComponent = new ArrayList<Component>(); } /* * 调用m_ListOfComponent中所有的元素的Operation * (非 Javadoc) * @see Compsite.Component#Operation() */ public void Operation() { System.out.println( "Operation by Composite "); for (Component component : m_ListOfComponent) { component.Operation(); } } public void Add(Component pChild) { // TODO 自动生成的方法存根 m_ListOfComponent.add(pChild); } public void Remove(Component pChild) { // TODO 自动生成的方法存根 m_ListOfComponent.remove(pChild); } public Component GetChild(int nIndex) { if (nIndex <= 0 || nIndex > m_ListOfComponent.size()) return null; return m_ListOfComponent.get(nIndex); } /*protected void finalize() throws Throwable { super.finalize(); Iterator iter1,iter2,temp; for(iter1 = m_ListOfComponent.iterator();;){} }*/ }
输出:
leaf构造方法
leaf构造方法
Operation by Composite
Operation by leaf
Operation by leaf
Operation by leaf
3. 装饰模式(DECORATOR)-对象结构型模式
意图
动态地给一个对象添加一些额外的职责。装饰模式充分利用了继承和聚合的优势,创造出无与论比的设计美学。就增加功能来说,Decorator模式相比生成子类更为灵活。
参与者
1. Component
定义一个对象接口,可以给这些对象动态地添加职责。
2. ConcreteComponent
定义一个对象,可以给这个对象添加一些职责。
3. Decorator
维持一个指向Component对象的指针,并定义一个与Component接口一致的接口。
4. ConcreteDecorator
向组件添加职责。
C#代码:
using System; namespace DoFactory.GangOfFour.Decorator.Structural { /// <summary> /// MainApp startup class for Structural /// Decorator Design Pattern. /// </summary> class MainApp { /// <summary> /// Entry point into console application. /// </summary> static void Main() { // Create ConcreteComponent and two Decorators ConcreteComponent c = new ConcreteComponent(); ConcreteDecoratorA d1 = new ConcreteDecoratorA(); ConcreteDecoratorB d2 = new ConcreteDecoratorB(); // Link decorators d1.SetComponent(c); d2.SetComponent(d1); d2.Operation(); // Wait for user Console.ReadKey(); } } /// <summary> /// The 'Component' abstract class /// </summary> abstract class Component { public abstract void Operation(); } /// <summary> /// The 'ConcreteComponent' class /// </summary> class ConcreteComponent : Component { public override void Operation() { Console.WriteLine("ConcreteComponent.Operation()"); } } /// <summary> /// The 'Decorator' abstract class /// </summary> abstract class Decorator : Component { protected Component component; public void SetComponent(Component component) { this.component = component; } public override void Operation() { if (component != null) { component.Operation(); } } } /// <summary> /// The 'ConcreteDecoratorA' class /// </summary> class ConcreteDecoratorA : Decorator { public override void Operation() { base.Operation(); Console.WriteLine("ConcreteDecoratorA.Operation()"); } } /// <summary> /// The 'ConcreteDecoratorB' class /// </summary> class ConcreteDecoratorB : Decorator { public override void Operation() { base.Operation(); AddedBehavior(); Console.WriteLine("ConcreteDecoratorB.Operation()"); } void AddedBehavior() { } } }
输出:
ConcreteComponent.Operation()
ConcreteDecoratorA.Operation()
ConcreteDecoratorB.Operation()