1.使用quartz
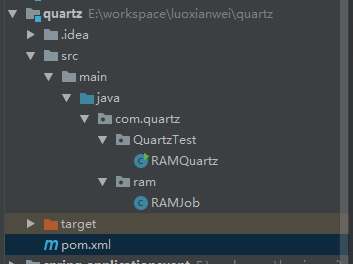
1.1 pom.xml
1 <?xml version="1.0" encoding="UTF-8"?>
2 <project xmlns="http://maven.apache.org/POM/4.0.0"
3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
4 xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
5 <modelVersion>4.0.0</modelVersion>
6
7 <groupId>com.quartz</groupId>
8 <artifactId>quartz</artifactId>
9 <version>1.0-SNAPSHOT</version>
10
11 <dependencies>
12 <!-- quartz -->
13 <dependency>
14 <groupId>org.quartz-scheduler</groupId>
15 <artifactId>quartz</artifactId>
16 <version>2.2.1</version>
17 </dependency>
18 <dependency><!-- 该依赖必加,里面有sping对schedule的支持 -->
19 <groupId>org.springframework</groupId>
20 <artifactId>spring-context-support</artifactId>
21 <version>4.3.13.RELEASE</version>
22 </dependency>
23 </dependencies>
24
25 </project>
1.2 RAMJob.class
1 package com.quartz.ram;
2
3 import org.quartz.Job;
4 import org.quartz.JobExecutionContext;
5 import org.quartz.JobExecutionException;
6 import org.slf4j.Logger;
7 import org.slf4j.LoggerFactory;
8
9 import java.util.Date;
10
11 public class RAMJob implements Job {
12
13 private static Logger _log = LoggerFactory.getLogger(RAMJob.class);
14
15 //@Override
16 public void execute(JobExecutionContext arg0) throws JobExecutionException {
17 System.out.println("执行定时任务");
18 _log.info("Say hello to Quartz" + new Date());
19 }
20
21 }
1.3 RAMQuartz.class
1 package com.quartz.QuartzTest;
2
3 import com.quartz.ram.RAMJob;
4 import org.quartz.*;
5 import org.quartz.impl.StdSchedulerFactory;
6 import org.slf4j.Logger;
7 import org.slf4j.LoggerFactory;
8
9 import java.util.Date;
10
11 /**
12 * This is a RAM Store Quartz!
13 *
14 * @author dufy
15 * @date 2017.02.04
16 */
17 public class RAMQuartz {
18
19 private static Logger _log = LoggerFactory.getLogger(RAMQuartz.class);
20
21 public static void main(String[] args) throws SchedulerException {
22 //1.创建Scheduler的工厂
23 SchedulerFactory sf = new StdSchedulerFactory();
24 //2.从工厂中获取调度器实例
25 Scheduler scheduler = sf.getScheduler();
26
27
28 //3.创建JobDetail
29 JobDetail jb = JobBuilder.newJob(RAMJob.class)
30 .withDescription("this is a ram job") //job的描述
31 .withIdentity("ramJob", "ramGroup") //job 的name和group
32 .build();
33
34 //任务运行的时间,SimpleSchedle类型触发器有效
35 long time = System.currentTimeMillis() + 3 * 1000L; //3秒后启动任务
36 Date statTime = new Date(time);
37
38 //4.创建Trigger
39 //使用SimpleScheduleBuilder或者CronScheduleBuilder
40 Trigger t = TriggerBuilder.newTrigger()
41 .withDescription("")
42 .withIdentity("ramTrigger", "ramTriggerGroup")
43 //.withSchedule(SimpleScheduleBuilder.simpleSchedule())
44 .startAt(statTime) //默认当前时间启动
45 .withSchedule(CronScheduleBuilder.cronSchedule("0/2 * * * * ?")) //两秒执行一次
46 .build();
47
48 //5.注册任务和定时器
49 scheduler.scheduleJob(jb, t);
50
51 //6.启动 调度器
52 scheduler.start();
53 _log.info("启动时间 : " + new Date());
54
55 }
56 }
1.4 使用方法启动RAMQuartz.class
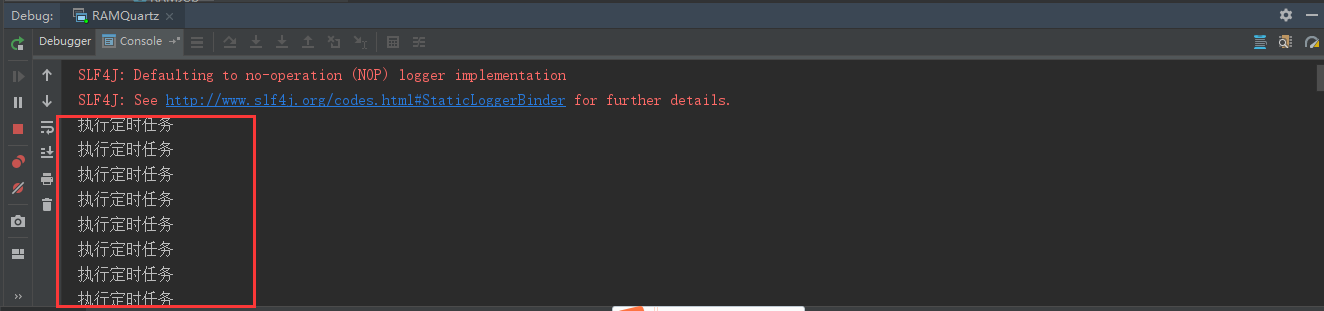
2.springboot-quartz springboot结合quartz完成定时任务
2.1 项目结构
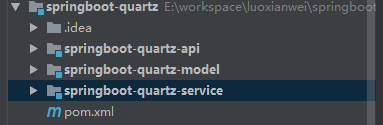
2.2 springboot-quartz pom.xml
1 <?xml version="1.0" encoding="UTF-8"?>
2 <project xmlns="http://maven.apache.org/POM/4.0.0"
3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
4 xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
5 <modelVersion>4.0.0</modelVersion>
6
7 <groupId>com.springboot.quartz</groupId>
8 <artifactId>springboot-quartz</artifactId>
9 <packaging>pom</packaging>
10 <version>1.0-SNAPSHOT</version>
11 <modules>
12 <module>springboot-quartz-api</module>
13 <module>springboot-quartz-model</module>
14 <module>springboot-quartz-service</module>
15 </modules>
16
17 <!-- Spring boot 父引用-->
18 <parent>
19 <groupId>org.springframework.boot</groupId>
20 <artifactId>spring-boot-starter-parent</artifactId>
21 <version>1.4.1.RELEASE</version>
22 </parent>
23
24 <build>
25 <plugins>
26 <plugin>
27 <groupId>org.springframework.boot</groupId>
28 <artifactId>spring-boot-maven-plugin</artifactId>
29 <executions>
30 <execution>
31 <goals>
32 <goal>repackage</goal>
33 </goals>
34 </execution>
35 </executions>
36 <configuration>
37 <executable>true</executable>
38 </configuration>
39 </plugin>
40 </plugins>
41 </build>
42
43 </project>
2.3 springboot-quartz-model模块结构
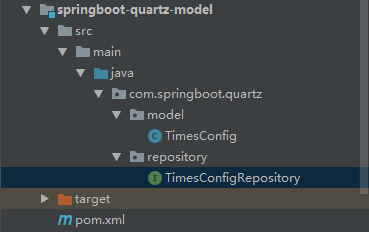
2.3.1 pom.xml
1 <?xml version="1.0" encoding="UTF-8"?>
2 <project xmlns="http://maven.apache.org/POM/4.0.0"
3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
4 xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
5 <parent>
6 <artifactId>springboot-quartz</artifactId>
7 <groupId>com.springboot.quartz</groupId>
8 <version>1.0-SNAPSHOT</version>
9 </parent>
10 <modelVersion>4.0.0</modelVersion>
11
12 <artifactId>springboot-quartz-model</artifactId>
13
14 <dependencies>
15 <dependency>
16 <groupId>org.springframework.boot</groupId>
17 <artifactId>spring-boot-starter-data-jpa</artifactId>
18 </dependency>
19 <dependency>
20 <groupId>org.projectlombok</groupId>
21 <artifactId>lombok</artifactId>
22 <version>1.16.18</version>
23 </dependency>
24 </dependencies>
25
26 </project>
2.3.2 TimesConfig.class
1 package com.springboot.quartz.model;
2
3 import lombok.Data;
4 import org.hibernate.annotations.GenericGenerator;
5
6 import javax.persistence.*;
7 import java.io.Serializable;
8 import java.util.Date;
9
10 @Entity
11 @Data
12 public class TimesConfig implements Serializable {
13
14 /**
15 * 主键
16 */
17 @Id
18 @GenericGenerator(name = "PKUUID", strategy = "uuid2")
19 @GeneratedValue(generator = "PKUUID")
20 @Column(columnDefinition = "varchar(255) comment '主键'")
21 private String id;
22
23 /**
24 * 触发器Cron代码
25 */
26 @Column(columnDefinition = "varchar(255) comment '触发器Cron代码'")
27 private String cron;
28
29 /**
30 * 创建日期
31 */
32 @Column(columnDefinition = "timestamp comment '创建日期'")
33 private Date dateCreated;
34
35 /**
36 * 最后更新日期
37 */
38 @Column(columnDefinition = "timestamp comment '最后更新日期'")
39 private Date lastUpdated;
40
41 /**
42 * 删除日期
43 */
44 @Column(columnDefinition = "timestamp comment '删除日期'")
45 private Date deleteDate;
46
47 /**
48 * 删除标记
49 */
50 @Column(columnDefinition = "int(1) comment '删除标记'")
51 private Boolean isDelete = false;
52
53 @PrePersist
54 protected void prePersist() {
55 dateCreated = new Date();
56 }
57
58 }
2.3.3 TimesConfigRepository.class
1 package com.springboot.quartz.repository;
2
3 import com.springboot.quartz.model.TimesConfig;
4 import org.springframework.data.jpa.repository.JpaRepository;
5
6 import java.util.List;
7
8 /**
9 * @author luoxianwei
10 * @date 2018/6/3
11 */
12 public interface TimesConfigRepository extends JpaRepository<TimesConfig, String> {
13
14 List<TimesConfig> findByIsDelete(Boolean isDelete);
15
16 TimesConfig findByIdAndIsDelete(String id, Boolean isDelete);
17
18 }
2.4 springboot-quartz-api 模块结构
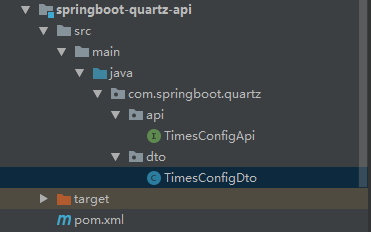
2.4.1 pom.xml
1 <?xml version="1.0" encoding="UTF-8"?>
2 <project xmlns="http://maven.apache.org/POM/4.0.0"
3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
4 xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
5 <parent>
6 <artifactId>springboot-quartz</artifactId>
7 <groupId>com.springboot.quartz</groupId>
8 <version>1.0-SNAPSHOT</version>
9 </parent>
10 <modelVersion>4.0.0</modelVersion>
11
12 <artifactId>springboot-quartz-api</artifactId>
13 <dependencies>
14 <dependency>
15 <groupId>org.projectlombok</groupId>
16 <artifactId>lombok</artifactId>
17 <version>1.16.18</version>
18 </dependency>
19 </dependencies>
20
21 </project>
2.4.2 TimesConfigDto.class
1 package com.springboot.quartz.dto;
2
3 import lombok.Data;
4
5 import java.io.Serializable;
6 import java.util.Date;
7
8 /**
9 * @author luoxianwei
10 * @date 2018/6/3
11 */
12 @Data
13 public class TimesConfigDto implements Serializable {
14
15 /**
16 * 主键
17 */
18 private String id;
19
20 /**
21 * 触发器Cron代码
22 */
23 private String cron;
24
25 /**
26 * 创建日期
27 */
28 private Date dateCreated;
29
30 /**
31 * 最后更新日期
32 */
33 private Date lastUpdated;
34
35 /**
36 * 删除日期
37 */
38 private Date deleteDate;
39
40 /**
41 * 删除标记
42 */
43 private Boolean isDelete = false;
44 }
2.4.3 TimesConfigApi.class
1 package com.springboot.quartz.api;
2
3 import com.springboot.quartz.dto.TimesConfigDto;
4
5 import java.util.List;
6
7 /**
8 * @date 2018/6/3
9 */
10 public interface TimesConfigApi {
11
12 /**
13 * 获取定时任务信息
14 * @return
15 */
16 List<TimesConfigDto> getAllTimerInfo();
17
18 /**
19 * 通过主键查询定时任务配置
20 * @param id
21 * @return
22 */
23 TimesConfigDto findById(String id);
24
25 /**
26 * 新增定时任务
27 * @param timesConfigDto
28 * @return
29 */
30 TimesConfigDto save(TimesConfigDto timesConfigDto);
31
32 }
2.5 springboot-quartz-service 模块结构
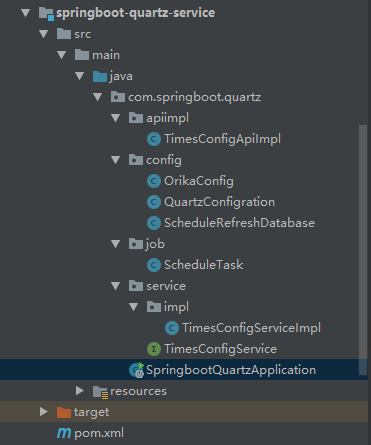
2.5.1 pom.xml
1 <?xml version="1.0" encoding="UTF-8"?>
2 <project xmlns="http://maven.apache.org/POM/4.0.0"
3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
4 xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
5 <parent>
6 <artifactId>springboot-quartz</artifactId>
7 <groupId>com.springboot.quartz</groupId>
8 <version>1.0-SNAPSHOT</version>
9 </parent>
10 <modelVersion>4.0.0</modelVersion>
11
12 <artifactId>springboot-quartz-service</artifactId>
13
14 <dependencies>
15 <!--springboot自动配置 不需要web依赖-->
16 <dependency>
17 <groupId>org.springframework.boot</groupId>
18 <artifactId>spring-boot-starter</artifactId>
19 </dependency>
20 <dependency>
21 <groupId>ma.glasnost.orika</groupId>
22 <artifactId>orika-core</artifactId>
23 <version>1.5.2</version>
24 </dependency>
25 <dependency>
26 <groupId>mysql</groupId>
27 <artifactId>mysql-connector-java</artifactId>
28 </dependency>
29 <!-- quartz -->
30 <dependency>
31 <groupId>org.quartz-scheduler</groupId>
32 <artifactId>quartz</artifactId>
33 <version>2.2.1</version>
34 </dependency>
35 <dependency><!-- 该依赖必加,里面有sping对schedule的支持 -->
36 <groupId>org.springframework</groupId>
37 <artifactId>spring-context-support</artifactId>
38 </dependency>
39
40 <!--依赖关系-->
41 <dependency>
42 <groupId>com.springboot.quartz</groupId>
43 <artifactId>springboot-quartz-model</artifactId>
44 <version>1.0-SNAPSHOT</version>
45 </dependency>
46 <dependency>
47 <groupId>com.springboot.quartz</groupId>
48 <artifactId>springboot-quartz-api</artifactId>
49 <version>1.0-SNAPSHOT</version>
50 </dependency>
51 </dependencies>
52
53 </project>
2.5.2 TimesConfigService.class
1 package com.springboot.quartz.service;
2
3 import com.springboot.quartz.dto.TimesConfigDto;
4
5 import java.util.List;
6
7 /**
8 * @date 2018/6/3
9 */
10 public interface TimesConfigService {
11
12 /**
13 * 获取全部未删除的配置
14 *
15 * @return
16 */
17 List<TimesConfigDto> getAll();
18
19 /**
20 * 通过主键查询定时任务配置
21 */
22 TimesConfigDto findByIdAndIsDelete(String id);
23
24 /**
25 * 新增定时任务
26 * @param timesConfigDto
27 * @return
28 */
29 TimesConfigDto save(TimesConfigDto timesConfigDto);
30
31 }
2.5.3 TimesConfigServiceImpl.class
1 package com.springboot.quartz.service.impl;
2
3 import com.springboot.quartz.dto.TimesConfigDto;
4 import com.springboot.quartz.model.TimesConfig;
5 import com.springboot.quartz.repository.TimesConfigRepository;
6 import com.springboot.quartz.service.TimesConfigService;
7 import ma.glasnost.orika.MapperFacade;
8 import org.springframework.beans.factory.annotation.Autowired;
9 import org.springframework.stereotype.Service;
10
11 import java.util.List;
12
13 /**
14 * @date 2018/6/3
15 */
16 @Service
17 public class TimesConfigServiceImpl implements TimesConfigService {
18
19 @Autowired
20 TimesConfigRepository timesConfigRepository;
21
22 @Autowired
23 MapperFacade mapperFacade;
24
25 /**
26 * 获取全部未删除的配置
27 *
28 * @return
29 */
30 @Override
31 public List<TimesConfigDto> getAll() {
32 List<TimesConfig> timesConfigs = timesConfigRepository.findByIsDelete(false);
33 return mapperFacade.mapAsList(timesConfigs, TimesConfigDto.class);
34 }
35
36 /**
37 * 通过主键查询定时任务配置
38 * @param id
39 * @return
40 */
41 @Override
42 public TimesConfigDto findByIdAndIsDelete(String id) {
43 TimesConfig timesConfig = timesConfigRepository.findByIdAndIsDelete(id,false);
44 return mapperFacade.map(timesConfig,TimesConfigDto.class);
45 }
46
47 /**
48 * 新增定时任务
49 * @param timesConfigDto
50 * @return
51 */
52 @Override
53 public TimesConfigDto save(TimesConfigDto timesConfigDto) {
54 TimesConfig timesConfig = mapperFacade.map(timesConfigDto,TimesConfig.class);
55 TimesConfig save = timesConfigRepository.save(timesConfig);
56 return mapperFacade.map(save,TimesConfigDto.class);
57 }
58 }
2.5.4 TimesConfigApiImpl.class
1 package com.springboot.quartz.apiimpl;
2
3 import com.springboot.quartz.api.TimesConfigApi;
4 import com.springboot.quartz.dto.TimesConfigDto;
5 import com.springboot.quartz.service.TimesConfigService;
6 import org.springframework.beans.factory.annotation.Autowired;
7 import org.springframework.stereotype.Service;
8
9 import java.util.List;
10
11 /**
12 * @date 2018/6/3
13 */
14 @Service
15 public class TimesConfigApiImpl implements TimesConfigApi {
16
17 @Autowired
18 TimesConfigService timesConfigService;
19
20 /**
21 * 获取定时任务信息
22 * @return
23 */
24 @Override
25 public List<TimesConfigDto> getAllTimerInfo() {
26 return timesConfigService.getAll();
27 }
28
29 /**
30 * 通过主键查询定时任务配置
31 * @param id
32 * @return
33 */
34 @Override
35 public TimesConfigDto findById(String id) {
36 return timesConfigService.findByIdAndIsDelete(id);
37 }
38
39 @Override
40 public TimesConfigDto save(TimesConfigDto timesConfigDto) {
41 return timesConfigService.save(timesConfigDto);
42 }
43 }
2.5.5 OrikaConfig.class
1 package com.springboot.quartz.config;
2
3 import ma.glasnost.orika.MapperFacade;
4 import ma.glasnost.orika.MapperFactory;
5 import ma.glasnost.orika.impl.DefaultMapperFactory;
6 import ma.glasnost.orika.metadata.ClassMapBuilder;
7 import org.springframework.context.annotation.Bean;
8 import org.springframework.context.annotation.Configuration;
9
10 import java.util.LinkedList;
11 import java.util.List;
12
13
14 @Configuration
15 public class OrikaConfig {
16
17 @Bean
18 MapperFactory mapperFactory() {
19 MapperFactory mapperFactory = new DefaultMapperFactory.Builder().build();
20 List<ClassMapBuilder> builders = new LinkedList<>();
21
22 for (ClassMapBuilder builder : builders) {
23 builder.byDefault().register();
24 }
25 return mapperFactory;
26 }
27
28 @Bean
29 MapperFacade mapperFacade() {
30 MapperFacade mapper = mapperFactory().getMapperFacade();
31 return mapper;
32 }
33
34
35 }
2.5.6 QuartzConfigration.class
1 package com.springboot.quartz.config;
2
3 import com.springboot.quartz.job.ScheduleTask;
4 import org.quartz.Trigger;
5 import org.springframework.context.annotation.Bean;
6 import org.springframework.context.annotation.Configuration;
7 import org.springframework.scheduling.quartz.CronTriggerFactoryBean;
8 import org.springframework.scheduling.quartz.MethodInvokingJobDetailFactoryBean;
9 import org.springframework.scheduling.quartz.SchedulerFactoryBean;
10
11 /**
12 * Quartz配置类
13 */
14 @Configuration
15 public class QuartzConfigration {
16 /**
17 * 配置定时任务
18 *
19 * @param task
20 * @return
21 */
22 @Bean(name = "jobDetail")
23 public MethodInvokingJobDetailFactoryBean detailFactoryBean(ScheduleTask task) {// ScheduleTask为需要执行的任务
24 MethodInvokingJobDetailFactoryBean jobDetail = new MethodInvokingJobDetailFactoryBean();
25
26 /**
27 * 是否并发执行
28 * 例如每5s执行一次任务,但是当前任务还没有执行完,就已经过了5s了,
29 * 如果此处为true,则下一个任务会执行,如果此处为false,则下一个任务会等待上一个任务执行完后,再开始执行
30 */
31 jobDetail.setConcurrent(false);
32
33 jobDetail.setName("srd-chhliu");// 设置任务的名字
34 jobDetail.setGroup("srd");// 设置任务的分组,这些属性都可以存储在数据库中,在多任务的时候使用
35
36 /**
37 * 为需要执行的实体类对应的对象
38 */
39 jobDetail.setTargetObject(task);
40
41 /**
42 * sayHello为需要执行的方法
43 * 通过这几个配置,告诉JobDetailFactoryBean我们需要执行定时执行ScheduleTask类中的sayHello方法
44 */
45 jobDetail.setTargetMethod("sayHello");
46 return jobDetail;
47 }
48
49 /**
50 * 配置定时任务的触发器,也就是什么时候触发执行定时任务
51 *
52 * @param jobDetail
53 * @return
54 */
55 @Bean(name = "jobTrigger")
56 public CronTriggerFactoryBean cronJobTrigger(MethodInvokingJobDetailFactoryBean jobDetail) {
57 CronTriggerFactoryBean tigger = new CronTriggerFactoryBean();
58 tigger.setJobDetail(jobDetail.getObject());
59 tigger.setCronExpression("0 30 20 * * ?");// 初始时的cron表达式
60 tigger.setName("srd-chhliu");// trigger的name
61 return tigger;
62
63 }
64
65 /**
66 * 定义quartz调度工厂
67 *
68 * @param cronJobTrigger
69 * @return
70 */
71 @Bean(name = "scheduler")
72 public SchedulerFactoryBean schedulerFactory(Trigger cronJobTrigger) {
73 SchedulerFactoryBean bean = new SchedulerFactoryBean();
74 //用于quartz集群,QuartzScheduler 启动时更新己存在的Job
75 bean.setOverwriteExistingJobs(true);
76 // 延时启动,应用启动1秒后
77 bean.setStartupDelay(1);
78 // 注册触发器
79 bean.setTriggers(cronJobTrigger);
80 return bean;
81 }
82 }
2.5.7 ScheduleRefreshDatabase.class
1 package com.springboot.quartz.config;
2
3 import com.springboot.quartz.api.TimesConfigApi;
4 import com.springboot.quartz.dto.TimesConfigDto;
5 import org.quartz.*;
6 import org.springframework.beans.factory.annotation.Autowired;
7 import org.springframework.context.annotation.Configuration;
8 import org.springframework.scheduling.annotation.EnableScheduling;
9 import org.springframework.scheduling.annotation.Scheduled;
10 import org.springframework.stereotype.Component;
11
12 import javax.annotation.Resource;
13
14 /**
15 * 定时查库,并更新任务
16 */
17 @Configuration
18 @EnableScheduling
19 @Component
20 public class ScheduleRefreshDatabase {
21
22 @Autowired
23 private TimesConfigApi timesConfigApi;
24
25 @Resource(name = "jobDetail")
26 private JobDetail jobDetail;
27
28 @Resource(name = "jobTrigger")
29 private CronTrigger cronTrigger;
30
31 @Resource(name = "scheduler")
32 private Scheduler scheduler;
33
34 @Scheduled(fixedRate = 5000) // 每隔5s查库,并根据查询结果决定是否重新设置定时任务
35 public void scheduleUpdateCronTrigger() throws SchedulerException {
36 CronTrigger trigger = (CronTrigger) scheduler.getTrigger(cronTrigger.getKey());
37 String currentCron = trigger.getCronExpression();// 当前Trigger使用的
38 // String searchCron = repository.findOne("1").getCron();// 从数据库查询出来的
39 TimesConfigDto timesConfigDto = timesConfigApi.findById("1");
40 String searchCron = timesConfigDto.getCron();
41 //System.out.println(currentCron);
42 //System.out.println(searchCron);
43 if (currentCron.equals(searchCron)) {
44 // 如果当前使用的cron表达式和从数据库中查询出来的cron表达式一致,则不刷新任务
45 } else {
46 // 表达式调度构建器
47 CronScheduleBuilder scheduleBuilder = CronScheduleBuilder.cronSchedule(searchCron);
48 // 按新的cronExpression表达式重新构建trigger
49 trigger = (CronTrigger) scheduler.getTrigger(cronTrigger.getKey());
50 trigger = trigger.getTriggerBuilder().withIdentity(cronTrigger.getKey())
51 .withSchedule(scheduleBuilder).build();
52 // 按新的trigger重新设置job执行
53 scheduler.rescheduleJob(cronTrigger.getKey(), trigger);
54 currentCron = searchCron;
55 }
56 }
57 }
2.5.8 ScheduleTask.class
1 package com.springboot.quartz.job;
2
3 import com.springboot.quartz.api.TimesConfigApi;
4 import com.springboot.quartz.dto.TimesConfigDto;
5 import org.slf4j.Logger;
6 import org.slf4j.LoggerFactory;
7 import org.springframework.beans.factory.annotation.Autowired;
8 import org.springframework.context.annotation.Configuration;
9 import org.springframework.scheduling.annotation.EnableScheduling;
10 import org.springframework.stereotype.Component;
11
12 import java.util.List;
13
14 /**
15 * 任务类
16 */
17 @Configuration
18 @Component // 此注解必加
19 @EnableScheduling // 此注解必加
20 public class ScheduleTask {
21 private static final Logger LOGGER = LoggerFactory.getLogger(ScheduleTask.class);
22
23 @Autowired
24 private TimesConfigApi timesConfigApi;
25
26 public void sayHello() {
27 /* TimesConfigDto timesConfigDto = new TimesConfigDto();
28 timesConfigDto.setCron("0/5 * * * * ?");
29 timesConfigApi.save(timesConfigDto);
30 System.out.println("添加成功");*/
31 List<TimesConfigDto> allTimerInfo = timesConfigApi.getAllTimerInfo();
32 System.out.println("执行成功");
33 LOGGER.info("Hello world, i'm the king of the world!!!");
34 }
35 }
2.5.9 application.properties
1 # 服务器端口号
2 server.port=7903
3 #配置数据源
4 spring.datasource.driver-class-name=com.mysql.jdbc.Driver
5 spring.datasource.url=jdbc:mysql://localhost:3306/time1
6 spring.datasource.username=root
7 spring.datasource.password=root
8 spring.jpa.hibernate.ddl-auto=update
9 spring.jpa.show-sql=false
10
11 #在控制台输出彩色日志
12 spring.output.ansi.enabled=always
2.5.10 SpringbootQuartzApplication.class
1 package com.springboot.quartz;
2
3 import org.springframework.boot.SpringApplication;
4 import org.springframework.boot.autoconfigure.SpringBootApplication;
5
6 /**
7 * @date 2018/6/2
8 */
9 @SpringBootApplication
10 public class SpringbootQuartzApplication {
11 public static void main(String[] args) {
12 SpringApplication.run(SpringbootQuartzApplication.class, args);
13 }
14 }
2.6 启动 SpringbootQuartzApplication.class
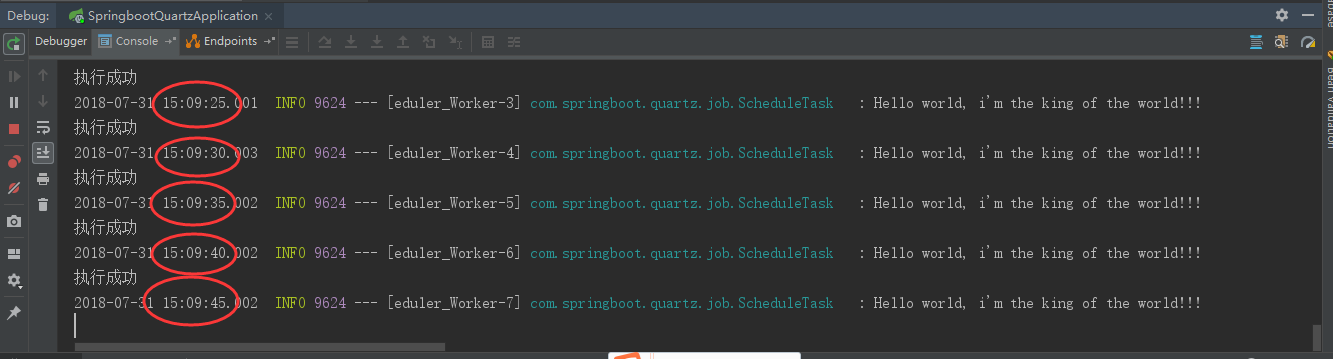
3. springboot-timedTask springboot自带的定时任务
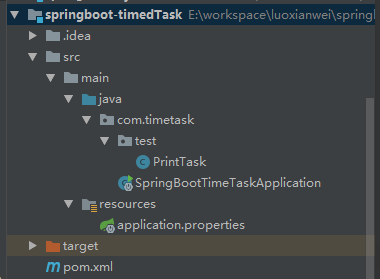
3.1 pom.xml
1 <?xml version="1.0" encoding="UTF-8"?>
2 <project xmlns="http://maven.apache.org/POM/4.0.0"
3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
4 xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
5 <modelVersion>4.0.0</modelVersion>
6
7 <groupId>com.timetask</groupId>
8 <artifactId>springboot-timedTask</artifactId>
9 <version>1.0-SNAPSHOT</version>
10
11 <!-- Spring boot 父引用-->
12 <parent>
13 <groupId>org.springframework.boot</groupId>
14 <artifactId>spring-boot-starter-parent</artifactId>
15 <version>1.4.1.RELEASE</version>
16 </parent>
17
18 <dependencies>
19 <!-- Spring boot 核心web-->
20 <dependency>
21 <groupId>org.springframework.boot</groupId>
22 <artifactId>spring-boot-starter-web</artifactId>
23 </dependency>
24 </dependencies>
25
26 <build>
27 <plugins>
28 <plugin>
29 <groupId>org.springframework.boot</groupId>
30 <artifactId>spring-boot-maven-plugin</artifactId>
31 <executions>
32 <execution>
33 <goals>
34 <goal>repackage</goal>
35 </goals>
36 </execution>
37 </executions>
38 <configuration>
39 <executable>true</executable>
40 </configuration>
41 </plugin>
42 </plugins>
43 </build>
44 </project>
3.2 PrintTask.class
1 package com.timetask.test;
2
3 import org.springframework.scheduling.annotation.Scheduled;
4 import org.springframework.stereotype.Component;
5 import java.text.SimpleDateFormat;
6 import java.util.Date;
7
8 /**
9 * @date 2018/6/2
10 */
11 @Component
12 public class PrintTask {
13
14 // 每分钟启动
15 //@Scheduled(cron = "0 0/1 * * * ?")
16 @Scheduled(cron = "0/5 * * * * ? ") // 间隔5秒执行
17 public void cron(){
18 System.out.println("执行测试时间:" + new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(new Date()));
19 }
20 }
3.3 application.properties
3.4 SpringBootTimeTaskApplication.class
1 package com.timetask;
2
3 import org.springframework.boot.SpringApplication;
4 import org.springframework.boot.autoconfigure.SpringBootApplication;
5 import org.springframework.scheduling.annotation.EnableScheduling;
6
7 @SpringBootApplication
8 @EnableScheduling //开启定时任务
9 public class SpringBootTimeTaskApplication {
10
11 public static void main(String[] args) {
12 SpringApplication.run(SpringBootTimeTaskApplication.class, args);
13 }
14
15 }
3.5 执行SpringBootTimeTaskApplication.class
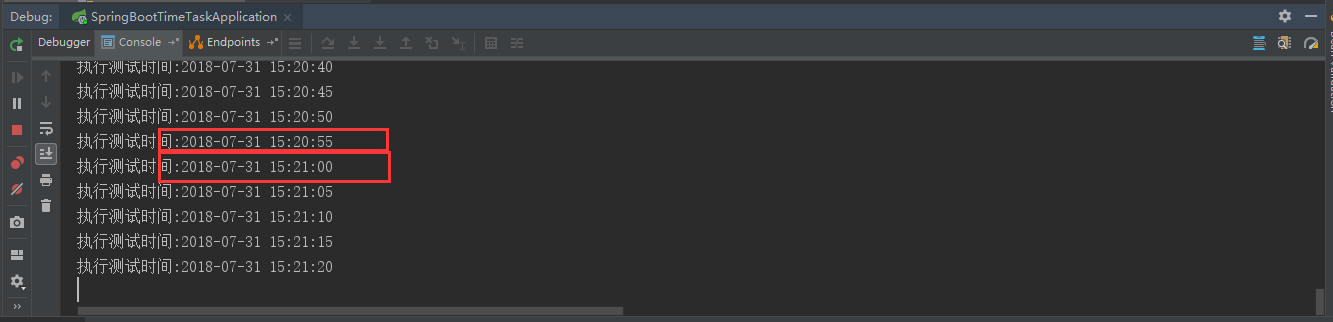