加两个UI模块
- (void)viewDidLoad
{
[self begin1];
[self begin2];
[super viewDidLoad];
// Do any additional setup after loading the view from its nib.
}
-(void)begin1{
UIActivityIndicatorView *viView = [[UIActivityIndicatorView alloc] initWithActivityIndicatorStyle:UIActivityIndicatorViewStyleWhiteLarge];
viView.center = CGPointMake(160, 300);
viView.color = [UIColor blueColor];
viView.hidesWhenStopped = NO;
[viView startAnimating];
[viView stopAnimating];
[self.view addSubview:viView];
}
-(void)begin2{
UISwitch *mySwitch = [[UISwitch alloc] initWithFrame:CGRectMake(100, 100, 200, 50)];
mySwitch.onTintColor = [UIColor redColor];
mySwitch.on = NO;
[mySwitch setOn:YES animated:YES];
[mySwitch addTarget:self action:@selector(onButton:) forControlEvents:UIControlEventValueChanged];
[self.view addSubview:mySwitch];
}
-(void)onButton:(id)sender{
NSLog(@":::%@", sender);
UISwitch *sw = sender;
if(sw.on) NSLog(@"ON");
else NSLog(@"OFF");
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
@end
{
[self begin1];
[self begin2];
[super viewDidLoad];
// Do any additional setup after loading the view from its nib.
}
-(void)begin1{
UIActivityIndicatorView *viView = [[UIActivityIndicatorView alloc] initWithActivityIndicatorStyle:UIActivityIndicatorViewStyleWhiteLarge];
viView.center = CGPointMake(160, 300);
viView.color = [UIColor blueColor];
viView.hidesWhenStopped = NO;
[viView startAnimating];
[viView stopAnimating];
[self.view addSubview:viView];
}
-(void)begin2{
UISwitch *mySwitch = [[UISwitch alloc] initWithFrame:CGRectMake(100, 100, 200, 50)];
mySwitch.onTintColor = [UIColor redColor];
mySwitch.on = NO;
[mySwitch setOn:YES animated:YES];
[mySwitch addTarget:self action:@selector(onButton:) forControlEvents:UIControlEventValueChanged];
[self.view addSubview:mySwitch];
}
-(void)onButton:(id)sender{
NSLog(@":::%@", sender);
UISwitch *sw = sender;
if(sw.on) NSLog(@"ON");
else NSLog(@"OFF");
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
@end
TextField 限制密码数目:
- (BOOL)textField:(UITextField *)textField shouldChangeCharactersInRange:(NSRange)range replacementString:(NSString *)string{
if(textField.text.length >= 6 && !range.length)
return NO;
return YES;
}
if(textField.text.length >= 6 && !range.length)
return NO;
return YES;
}
有多个TextField 则限制所指定的数目:
- (BOOL)textField:(UITextField *)textField shouldChangeCharactersInRange:(NSRange)range replacementString:(NSString *)string{
NSLog(@"%d-%d-%d",range.length, range.location, textField.text.length);
if (textField == self.nameTextField) {
if(textField.text.length >= 6 && !range.length)
return NO;
return YES;
}
if (textField == self.passwordTextField){
if(textField.text.length >= 4 && !range.length)
return NO;
return YES;
}
return YES;
}
NSLog(@"%d-%d-%d",range.length, range.location, textField.text.length);
if (textField == self.nameTextField) {
if(textField.text.length >= 6 && !range.length)
return NO;
return YES;
}
if (textField == self.passwordTextField){
if(textField.text.length >= 4 && !range.length)
return NO;
return YES;
}
return YES;
}
手势:
添加手势:
UITapGestureRecognizer *tapGR;//添加单击手势
tapGR=[[UITapGestureRecognizer alloc]initWithTarget:self action:@selector(onTap:)];
[self.view addGestureRecognizer:tapGR];
[self.view removeGestureRecognizer:tapGR];
-(void)onTap:(id)sender{
//将输入框取消第一响应者,键盘就睡自动收回
[self.nameTextField resignFirstResponder];
[self.passwordTextField resignFirstResponder];
}
//将输入框取消第一响应者,键盘就睡自动收回
[self.nameTextField resignFirstResponder];
[self.passwordTextField resignFirstResponder];
}
-(void)dealloc{
//在销毁的时候将自己在通知中心中移除
[[NSNotificationCenter defaultCenter]removeObserver:self];
}
//在销毁的时候将自己在通知中心中移除
[[NSNotificationCenter defaultCenter]removeObserver:self];
}
- (BOOL)textFieldShouldReturn:(UITextField *)textField{
NSLog(@"textFiled 键盘的return键被点击");
[textField resignFirstResponder];
NSLog(@"开始搜索!!!");
return YES;
}
NSLog(@"textFiled 键盘的return键被点击");
[textField resignFirstResponder];
NSLog(@"开始搜索!!!");
return YES;
}
自己做一个小调色板(红色、蓝色、绿色、透明度):如图
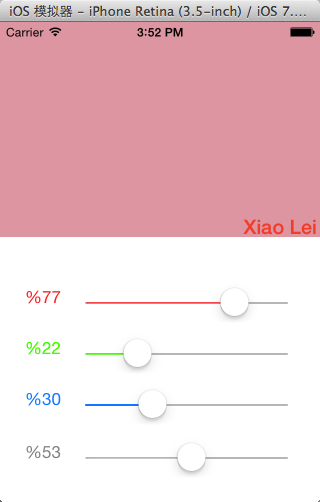
代码:
@interface ViewController ()
@property (strong, nonatomic) IBOutlet UIView *subView;
@property (nonatomic, assign)float red;
@property (nonatomic, assign)float blue;
@property (nonatomic, assign)float green;
@property (nonatomic, assign)float alpha;
@property (weak, nonatomic) IBOutlet UILabel *redLabel;
@property (weak, nonatomic) IBOutlet UILabel *greenLabel;
@property (weak, nonatomic) IBOutlet UILabel *blueLabel;
@property (weak, nonatomic) IBOutlet UILabel *alphaLabel;
@end
@implementation ViewController
- (void)viewDidLoad
{
[super viewDidLoad];
_redLabel.text = [NSString stringWithFormat:@"%%50"];
_blueLabel.text = [NSString stringWithFormat:@"%%50"];
_greenLabel.text = [NSString stringWithFormat:@"%%50"];
_alphaLabel.text = [NSString stringWithFormat:@"%%50"];
_red = _green = _blue = _alpha = 0.5;
self.subView.backgroundColor = [UIColor colorWithRed:_red green:_green blue:_blue alpha:_alpha];
// Do any additional setup after loading the view, typically from a nib.
UISlider *slide = [[UISlider alloc] initWithFrame:CGRectMake(10, 300, 300, 60)];
slide.minimumValue = 0;
slide.maximumValue = 1;
[slide setValue:0.5 animated:YES];
[slide addTarget:self action:@selector(onSliderChange:) forControlEvents:UIControlEventValueChanged];
//[self.view addSubview:slide];
}
-(void)onSliderChange:(id)sender{
NSLog(@"slider:%@", sender);
UISlider *slider = sender;
self.subView.alpha = slider.value;
}
- (IBAction)blueSlider:(id)sender {
UISlider *slider = sender;
_blue = slider.value;
_blueLabel.text = [NSString stringWithFormat:@"%%%.0lf", slider.value*100];
self.subView.backgroundColor = [UIColor colorWithRed:_red green:_green blue:_blue alpha:_alpha];
}
- (IBAction)greenSlider:(id)sender {
UISlider *slider = sender;
_green = slider.value;
_greenLabel.text = [NSString stringWithFormat:@"%%%.0lf", slider.value*100];
self.subView.backgroundColor = [UIColor colorWithRed:_red green:_green blue:_blue alpha:_alpha];
}
- (IBAction)redSlider:(id)sender {
UISlider *slider = sender;
_red = slider.value;
_redLabel.text = [NSString stringWithFormat:@"%%%.0lf", slider.value*100];
self.subView.backgroundColor = [UIColor colorWithRed:_red green:_green blue:_blue alpha:_alpha];
}
- (IBAction)alphaSlider:(id)sender {
UISlider *slider = sender;
_alpha = slider.value;
_alphaLabel.text = [NSString stringWithFormat:@"%%%.0lf", slider.value*100];
self.subView.backgroundColor = [UIColor colorWithRed:_red green:_green blue:_blue alpha:_alpha];
}
@property (strong, nonatomic) IBOutlet UIView *subView;
@property (nonatomic, assign)float red;
@property (nonatomic, assign)float blue;
@property (nonatomic, assign)float green;
@property (nonatomic, assign)float alpha;
@property (weak, nonatomic) IBOutlet UILabel *redLabel;
@property (weak, nonatomic) IBOutlet UILabel *greenLabel;
@property (weak, nonatomic) IBOutlet UILabel *blueLabel;
@property (weak, nonatomic) IBOutlet UILabel *alphaLabel;
@end
@implementation ViewController
- (void)viewDidLoad
{
[super viewDidLoad];
_redLabel.text = [NSString stringWithFormat:@"%%50"];
_blueLabel.text = [NSString stringWithFormat:@"%%50"];
_greenLabel.text = [NSString stringWithFormat:@"%%50"];
_alphaLabel.text = [NSString stringWithFormat:@"%%50"];
_red = _green = _blue = _alpha = 0.5;
self.subView.backgroundColor = [UIColor colorWithRed:_red green:_green blue:_blue alpha:_alpha];
// Do any additional setup after loading the view, typically from a nib.
UISlider *slide = [[UISlider alloc] initWithFrame:CGRectMake(10, 300, 300, 60)];
slide.minimumValue = 0;
slide.maximumValue = 1;
[slide setValue:0.5 animated:YES];
[slide addTarget:self action:@selector(onSliderChange:) forControlEvents:UIControlEventValueChanged];
//[self.view addSubview:slide];
}
-(void)onSliderChange:(id)sender{
NSLog(@"slider:%@", sender);
UISlider *slider = sender;
self.subView.alpha = slider.value;
}
- (IBAction)blueSlider:(id)sender {
UISlider *slider = sender;
_blue = slider.value;
_blueLabel.text = [NSString stringWithFormat:@"%%%.0lf", slider.value*100];
self.subView.backgroundColor = [UIColor colorWithRed:_red green:_green blue:_blue alpha:_alpha];
}
- (IBAction)greenSlider:(id)sender {
UISlider *slider = sender;
_green = slider.value;
_greenLabel.text = [NSString stringWithFormat:@"%%%.0lf", slider.value*100];
self.subView.backgroundColor = [UIColor colorWithRed:_red green:_green blue:_blue alpha:_alpha];
}
- (IBAction)redSlider:(id)sender {
UISlider *slider = sender;
_red = slider.value;
_redLabel.text = [NSString stringWithFormat:@"%%%.0lf", slider.value*100];
self.subView.backgroundColor = [UIColor colorWithRed:_red green:_green blue:_blue alpha:_alpha];
}
- (IBAction)alphaSlider:(id)sender {
UISlider *slider = sender;
_alpha = slider.value;
_alphaLabel.text = [NSString stringWithFormat:@"%%%.0lf", slider.value*100];
self.subView.backgroundColor = [UIColor colorWithRed:_red green:_green blue:_blue alpha:_alpha];
}
按钮开关相应事件:

- (void)viewDidLoad
{
[self begin1];
[self begin2];
[super viewDidLoad];
// Do any additional setup after loading the view from its nib.
}
-(void)begin1{
UIActivityIndicatorView *viView = [[UIActivityIndicatorView alloc] initWithActivityIndicatorStyle:UIActivityIndicatorViewStyleWhiteLarge];
viView.center = CGPointMake(160, 300);
viView.color = [UIColor blueColor];
viView.hidesWhenStopped = NO;
[viView startAnimating];
[viView stopAnimating];
[self.view addSubview:viView];
}
-(void)begin2{
UISwitch *mySwitch = [[UISwitch alloc] initWithFrame:CGRectMake(100, 100, 200, 50)];
mySwitch.onTintColor = [UIColor redColor];
mySwitch.on = NO;
[mySwitch setOn:YES animated:YES];
[mySwitch addTarget:self action:@selector(onButton:) forControlEvents:UIControlEventValueChanged];
[self.view addSubview:mySwitch];
}
-(void)onButton:(id)sender{
NSLog(@":::%@", sender);
UISwitch *sw = sender;
if(sw.on) NSLog(@"ON");
else NSLog(@"OFF");
}
{
[self begin1];
[self begin2];
[super viewDidLoad];
// Do any additional setup after loading the view from its nib.
}
-(void)begin1{
UIActivityIndicatorView *viView = [[UIActivityIndicatorView alloc] initWithActivityIndicatorStyle:UIActivityIndicatorViewStyleWhiteLarge];
viView.center = CGPointMake(160, 300);
viView.color = [UIColor blueColor];
viView.hidesWhenStopped = NO;
[viView startAnimating];
[viView stopAnimating];
[self.view addSubview:viView];
}
-(void)begin2{
UISwitch *mySwitch = [[UISwitch alloc] initWithFrame:CGRectMake(100, 100, 200, 50)];
mySwitch.onTintColor = [UIColor redColor];
mySwitch.on = NO;
[mySwitch setOn:YES animated:YES];
[mySwitch addTarget:self action:@selector(onButton:) forControlEvents:UIControlEventValueChanged];
[self.view addSubview:mySwitch];
}
-(void)onButton:(id)sender{
NSLog(@":::%@", sender);
UISwitch *sw = sender;
if(sw.on) NSLog(@"ON");
else NSLog(@"OFF");
}
触摸方法:
@implementation QFViewController{
UIView *redView;
CGPoint beginPoint;
BOOL canMove;
BOOL mark;
}
- (void)viewDidLoad
{
[super viewDidLoad];
redView=[[UIView alloc]initWithFrame:CGRectMake(-320, 0, 320, 480)];
redView.backgroundColor=[UIColor redColor];
[self.view addSubview:redView];
mark = 0;
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
#pragma mark--
#pragma mark touch事件
-(void)touchesBegan:(NSSet *)touches withEvent:(UIEvent *)event{
//任意取出一个touch对象
UITouch *touch = touches.anyObject;
beginPoint = [touch locationInView:self.view];
NSLog(@"mark = %d", mark);
if(mark == 0){
if (beginPoint.x<20) {
canMove=YES;
}else{
canMove=NO;
}
}
if(mark){
if (beginPoint.x]]]]>300) {
canMove=YES;
}else{
canMove=NO;
}
}
NSLog(@"触摸开始 %f,%f---",beginPoint.x,beginPoint.y);
}
-(void)touchesMoved:(NSSet *)touches withEvent:(UIEvent *)event{
UITouch *touch = touches.anyObject;
CGPoint movePoint = [touch locationInView:self.view];
NSLog(@"触摸滑动 %f",movePoint.x-beginPoint.x);
if (canMove && mark == 0) {
redView.frame=CGRectMake(-320+movePoint.x-beginPoint.x, 0, 320, 480);
}
if( canMove && mark == 1){
redView.frame=CGRectMake(movePoint.x - 320 , 0, 320, 480);
}
}
-(void)touchesEnded:(NSSet *)touches withEvent:(UIEvent *)event{
NSLog(@"触摸结束");
UITouch *touch = touches.anyObject;
CGPoint endPoint = [touch locationInView:self.view];
if(mark == 0){
if ((endPoint.x-beginPoint.x)>200) {
[UIView animateWithDuration:0.25 animations:^{
redView.frame=CGRectMake(0, 0, 320, 480);
}];
mark = 1;
}else{
[UIView animateWithDuration:0.25 animations:^{
redView.frame=CGRectMake(-320, 0, 320, 480);
}];
}
}
else if(mark == 1){
NSLog(@"duanyulei");
if ((320 - endPoint.x)<20) {
[UIView animateWithDuration:0.25 animations:^{
redView.frame=CGRectMake(-320, 0, 320, 480);
}];
mark = 0;
}else{
[UIView animateWithDuration:0.25 animations:^{
redView.frame=CGRectMake(0, 0, 320, 480);
}];
}
}
}
-(void)touchesCancelled:(NSSet *)touches withEvent:(UIEvent *)event{
NSLog(@"触摸取消");
}
UIView *redView;
CGPoint beginPoint;
BOOL canMove;
BOOL mark;
}
- (void)viewDidLoad
{
[super viewDidLoad];
redView=[[UIView alloc]initWithFrame:CGRectMake(-320, 0, 320, 480)];
redView.backgroundColor=[UIColor redColor];
[self.view addSubview:redView];
mark = 0;
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
#pragma mark--
#pragma mark touch事件
-(void)touchesBegan:(NSSet *)touches withEvent:(UIEvent *)event{
//任意取出一个touch对象
UITouch *touch = touches.anyObject;
beginPoint = [touch locationInView:self.view];
NSLog(@"mark = %d", mark);
if(mark == 0){
if (beginPoint.x<20) {
canMove=YES;
}else{
canMove=NO;
}
}
if(mark){
if (beginPoint.x]]]]>300) {
canMove=YES;
}else{
canMove=NO;
}
}
NSLog(@"触摸开始 %f,%f---",beginPoint.x,beginPoint.y);
}
-(void)touchesMoved:(NSSet *)touches withEvent:(UIEvent *)event{
UITouch *touch = touches.anyObject;
CGPoint movePoint = [touch locationInView:self.view];
NSLog(@"触摸滑动 %f",movePoint.x-beginPoint.x);
if (canMove && mark == 0) {
redView.frame=CGRectMake(-320+movePoint.x-beginPoint.x, 0, 320, 480);
}
if( canMove && mark == 1){
redView.frame=CGRectMake(movePoint.x - 320 , 0, 320, 480);
}
}
-(void)touchesEnded:(NSSet *)touches withEvent:(UIEvent *)event{
NSLog(@"触摸结束");
UITouch *touch = touches.anyObject;
CGPoint endPoint = [touch locationInView:self.view];
if(mark == 0){
if ((endPoint.x-beginPoint.x)>200) {
[UIView animateWithDuration:0.25 animations:^{
redView.frame=CGRectMake(0, 0, 320, 480);
}];
mark = 1;
}else{
[UIView animateWithDuration:0.25 animations:^{
redView.frame=CGRectMake(-320, 0, 320, 480);
}];
}
}
else if(mark == 1){
NSLog(@"duanyulei");
if ((320 - endPoint.x)<20) {
[UIView animateWithDuration:0.25 animations:^{
redView.frame=CGRectMake(-320, 0, 320, 480);
}];
mark = 0;
}else{
[UIView animateWithDuration:0.25 animations:^{
redView.frame=CGRectMake(0, 0, 320, 480);
}];
}
}
}
-(void)touchesCancelled:(NSSet *)touches withEvent:(UIEvent *)event{
NSLog(@"触摸取消");
}
警告和通知:
@interface QFViewController ()<UIAlertViewDelegate,UIActionSheetDelegate]]]]>
- (IBAction)showAlertView:(id)sender;
@property (weak, nonatomic) IBOutlet UIButton *showActionSheet;
- (IBAction)showActionSheet:(id)sender;
@end
@implementation QFViewController{
UIAlertView *alertView;
UIActionSheet *actionSheet;
}
- (void)viewDidLoad
{
[super viewDidLoad];
alertView=[[UIAlertView alloc]initWithTitle:@"通知" message:@"明天礼拜五了,大家晚上多多努力,争取在放假前把项目上架。" delegate:self cancelButtonTitle:@"取消" otherButtonTitles:@"确定",nil,nil];
actionSheet=[[UIActionSheet alloc]initWithTitle:@"你确定删除么?" delegate:self cancelButtonTitle:@"取消" destructiveButtonTitle:@"确定" otherButtonTitles:@"其他", nil];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (IBAction)showAlertView:(id)sender {
[alertView show];
}
- (IBAction)showActionSheet:(id)sender {
[actionSheet showInView:self.view];
}
#pragma mark--
#pragma mark UIAlertView代理方法
- (void)alertView:(UIAlertView *)alertView clickedButtonAtIndex:(NSInteger)buttonIndex{
NSLog(@"点击了第%d个按键",buttonIndex);
switch (buttonIndex) {
case 0:
break;
default:
break;
}
}
- (void)actionSheet:(UIActionSheet *)actionSheet clickedButtonAtIndex:(NSInteger)buttonIndex{
NSLog(@"点击了第%d个按键",buttonIndex);
switch (buttonIndex) {
case 0:
break;
default:
break;
}
}