Description
Once upon a time there was a greedy King who ordered his chief Architect to build a wall around the King's castle. The King was so greedy, that he would not listen to his Architect's proposals to build a beautiful brick wall with a perfect shape and nice tall towers. Instead, he ordered to build the wall around the whole castle using the least amount of stone and labor, but demanded that the wall should not come closer to the castle than a certain distance. If the King finds that the Architect has used more resources to build the wall than it was absolutely necessary to satisfy those requirements, then the Architect will loose his head. Moreover, he demanded Architect to introduce at once a plan of the wall listing the exact amount of resources that are needed to build the wall.
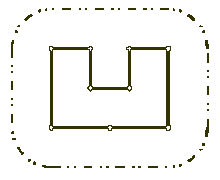
Your task is to help poor Architect to save his head, by writing a program that will find the minimum possible length of the wall that he could build around the castle to satisfy King's requirements.
The task is somewhat simplified by the fact, that the King's castle has a polygonal shape and is situated on a flat ground. The Architect has already established a Cartesian coordinate system and has precisely measured the coordinates of all castle's vertices in feet.
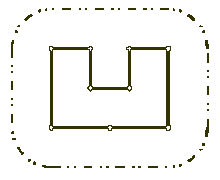
The task is somewhat simplified by the fact, that the King's castle has a polygonal shape and is situated on a flat ground. The Architect has already established a Cartesian coordinate system and has precisely measured the coordinates of all castle's vertices in feet.
Input
The first line of the input file contains two integer numbers N and L separated by a space. N (3 <= N <= 1000) is the number of vertices in the King's castle, and L (1 <= L <= 1000) is the minimal number of feet that King allows for the wall to come close to the castle.
Next N lines describe coordinates of castle's vertices in a clockwise order. Each line contains two integer numbers Xi and Yi separated by a space (-10000 <= Xi, Yi <= 10000) that represent the coordinates of ith vertex. All vertices are different and the sides of the castle do not intersect anywhere except for vertices.
Next N lines describe coordinates of castle's vertices in a clockwise order. Each line contains two integer numbers Xi and Yi separated by a space (-10000 <= Xi, Yi <= 10000) that represent the coordinates of ith vertex. All vertices are different and the sides of the castle do not intersect anywhere except for vertices.
Output
Write to the output file the single number that represents the minimal possible length of the wall in feet that could be built around the castle to satisfy King's requirements. You must present the integer number of feet to the King, because the floating numbers are not invented yet. However, you must round the result in such a way, that it is accurate to 8 inches (1 foot is equal to 12 inches), since the King will not tolerate larger error in the estimates.
Sample Input
9 100 200 400 300 400 300 300 400 300 400 400 500 400 500 200 350 200 200 200
Sample Output
1628
Hint
结果四舍五入就可以了
解题思路:题意其实就是在一个凸包外建立一座围墙,要求围墙到凸包(城堡)的最小距离为L,求此围墙的最小总长度。公式:围墙的最小总长度=凸包周长+一个以L为半径的圆周长。证明:假设顺时针给出4个点A、B、C、D(都是凸包的顶点)组成一个凸四边形ABCD。不妨过A点作AE、AF分别垂直于AB、AD,过B点作BG、BH分别垂直于AB、BC......过A点以L为半径作一段弧连到AF,同理使GH成为一段弧。显然EG平行且等于AB......对其他顶点进行同样的操作后,可得出围墙的最小值=四边形的周长+四个顶点对应的弧长(半径都为L)之和。如图所示:
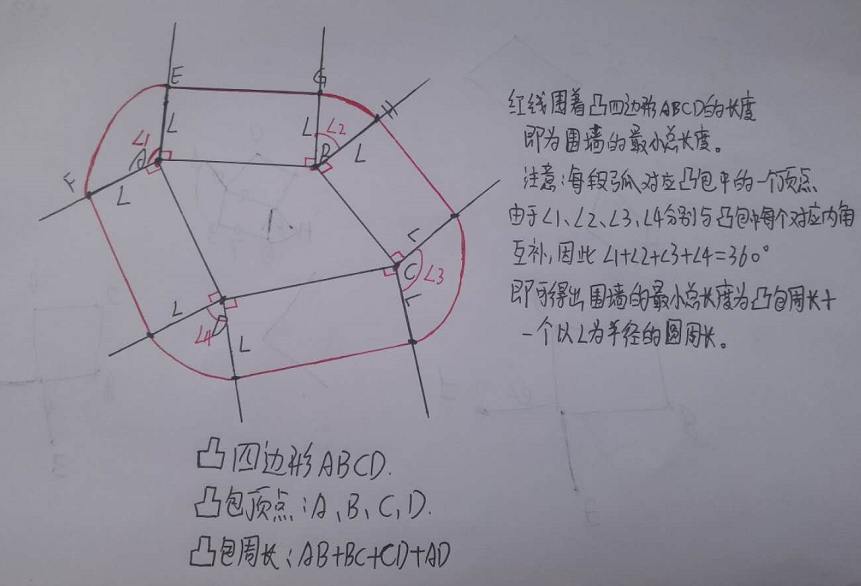
推广到任意凸多边形,每段圆弧都是以凸包上每个对应的顶点为圆心,给定的L为半径,与相邻两条边的切线之间的一段圆弧。每段圆弧的两条半径的夹角与凸包内对应的内角互补。设凸包上有n个顶点,则组成了n个圆周角,总角度数为360°*n=2*180°*n,凸包(凸多边形)的内角和为180°*(n-2),作了2*n条垂线,那么所有垂角之和为2*n*90°=180°*n,因此所有小圆弧对应的圆心角之和为2*180°*n-180°*(n-2)-180°*n=360°(为一个以L为半径的圆)。综上所述,围墙的最小总长度=凸包周长+一个以L为半径的圆周长。
AC代码(63ms):Graham-scan算法:时间复杂度为0(nlogn)。
1 #include<iostream> 2 #include<string.h> 3 #include<algorithm> 4 #include<cstdio> 5 #include<cmath> 6 using namespace std; 7 const int maxn=1005; 8 const double PI=acos(-1.0); 9 struct node{int x,y;}; 10 node vex[maxn]; 11 node stackk[maxn]; 12 bool cmp1(node a,node b){ 13 if(a.y==b.y)return a.x<b.x; 14 else return a.y<b.y; 15 } 16 bool cmp2(node a,node b){ 17 double A=atan2(a.y-stackk[0].y,a.x-stackk[0].x); 18 double B=atan2(b.y-stackk[0].y,b.x-stackk[0].x); 19 if(A!=B)return A<B; 20 else return a.x<b.x; 21 } 22 int cross(node p0,node p1,node p2){ 23 return (p1.x-p0.x)*(p2.y-p0.y)-(p2.x-p0.x)*(p1.y-p0.y); 24 } 25 double dis(node a,node b){ 26 return sqrt((a.x-b.x)*(a.x-b.x)*1.0+(a.y-b.y)*(a.y-b.y)); 27 } 28 int main(){ 29 int n,l; 30 while(~scanf("%d%d",&n,&l)){ 31 for(int i=0;i<n;++i)//输入t个点 32 scanf("%d%d",&vex[i].x,&vex[i].y); 33 memset(stackk,0,sizeof(stackk)); 34 sort(vex,vex+n,cmp1); 35 stackk[0]=vex[0]; 36 sort(vex+1,vex+n,cmp2); 37 stackk[1]=vex[1]; 38 int top=1; 39 for(int i=2;i<n;++i){ 40 while(top>0&&cross(stackk[top-1],stackk[top],vex[i])<=0)top--; 41 stackk[++top]=vex[i]; 42 } 43 double s=0; 44 for(int i=1;i<=top;++i) 45 s+=dis(stackk[i-1],stackk[i]); 46 s+=dis(stackk[top],vex[0]); 47 s+=2*PI*l;//加上圆的周长 48 printf("%d ",(int)(s+0.5));//四舍五入 49 } 50 return 0; 51 }
AC代码二(32ms):Andrew算法:时间复杂度为O(nlogn),但比Graham-scan算法还快!
1 #include<iostream> 2 #include<string.h> 3 #include<algorithm> 4 #include<cstdio> 5 #include<cmath> 6 using namespace std; 7 const int maxn=1005; 8 const double PI=acos(-1.0); 9 struct node{int x,y;}vex[maxn],stackk[maxn]; 10 bool cmp(node a,node b){//坐标排序 11 return ((a.y<b.y)||(a.y==b.y&&a.x<b.x)); 12 } 13 int cross(node p0,node p1,node p2){ 14 return (p1.x-p0.x)*(p2.y-p0.y)-(p2.x-p0.x)*(p1.y-p0.y); 15 } 16 double dis(node a,node b){ 17 return sqrt((a.x-b.x)*(a.x-b.x)*1.0+(a.y-b.y)*(a.y-b.y)); 18 } 19 int main(){ 20 int n,l; 21 while(~scanf("%d%d",&n,&l)){ 22 for(int i=0;i<n;++i) 23 scanf("%d%d",&vex[i].x,&vex[i].y); 24 memset(stackk,0,sizeof(stackk)); 25 sort(vex,vex+n,cmp); 26 int top=-1; 27 for(int i=0;i<n;++i){//构造凸包下侧 28 while(top>0&&cross(stackk[top-1],stackk[top],vex[i])<=0)top--; 29 stackk[++top]=vex[i]; 30 } 31 for(int i=n-2,k=top;i>=0;--i){//构造凸包上侧 32 while(top>k&&cross(stackk[top-1],stackk[top],vex[i])<=0)top--; 33 stackk[++top]=vex[i]; 34 } 35 double s=0; 36 for(int i=1;i<=top;++i)//计算凸包周长 37 s+=dis(stackk[i-1],stackk[i]); 38 s+=2*PI*l; 39 printf("%d ",(int)(s+0.5)); 40 } 41 return 0; 42 }