给定 n 个非负整数表示每个宽度为 1 的柱子的高度图,计算按此排列的柱子,下雨之后能接多少雨水。
上面是由数组 [0,1,0,2,1,0,1,3,2,1,2,1] 表示的高度图,在这种情况下,可以接 6 个单位的雨水(蓝色部分表示雨水)。 感谢 Marcos 贡献此图。
示例:
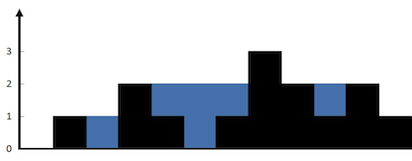
输入: [0,1,0,2,1,0,1,3,2,1,2,1]
输出: 6
输出: 6
思路:1.接完水后的数组最大值的左边不严格递增,最大值的右边不严格递减。
2.先找到最大值的下标
3.左边遍历到最大值下标,右边遍历到最大值的下标,发现左右两边遍历的时候分别用一个cur表示最大值索引,如果发现索引过程中小于最大值,则 res += cur - height[i] .
4.可以极限一点想,假设我们已经找到最大值下标,则左右两边只要高度不等于0则都能存水,存水量就是当前索引的最大值与下一个高度的差。
1 int trap(int* height, int heightSize) 2 { 3 int n = heightSize; 4 if(n == 0) 5 { 6 return 0; 7 } 8 int maxIndex = 0; 9 int max = height[0]; 10 for(int i = 1; i < n; i++) 11 { 12 if(height[i] > max) 13 { 14 maxIndex = i; 15 max = height[i]; 16 } 17 } 18 int res = 0,cur = height[0]; 19 for(int i = 1; i < maxIndex; i++) 20 { 21 if(height[i] < cur) 22 { 23 res += cur - height[i]; 24 } 25 else 26 { 27 cur = height[i]; 28 } 29 } 30 cur = height[n-1]; 31 for(int i = n-2; i > maxIndex; i--) 32 { 33 if(height[i] < cur) 34 { 35 res += cur-height[i]; 36 } 37 else 38 { 39 cur = height[i]; 40 } 41 } 42 return res; 43 }