今日任务:对电影详情界面进行优化,主要完成了“星级评分”模块,使界面可以根据电影评分的不同而产生不同的星数。
星级评分模块主要代码:
<div class="score">
<p class="star">豆瓣评分:<font size="5" face="arial" color="black">9.7</font></p>
<canvas width="250" height="20" id="D_myCanvas"></canvas>
<p class="star">腾讯视频评分:<font size="5" face="arial" color="black">8.5</font></p>
<canvas width="250" height="20" id="T_myCanvas"></canvas>
<p class="star">爱奇艺评分:<font size="5" face="arial" color="black">6.0</font></p>
<canvas width="250" height="20" id="A_myCanvas"></canvas>
<p class="star">IMDB评分:<font size="5" face="arial" color="black">7.5</font></p>
<canvas width="250" height="20" id="I_myCanvas"></canvas>
</div>
<!--为每一个电影模块定义一个画布对象,实现方法一致,只需改变画布对象名称-->
<!--豆瓣评分-->
<script>
/**
* showRatingStars 显示评分星级
* @param {Object} myCanvas 画布对象
* @param {Number} rating 评分
* @param {Number} counts star个数
* @param {Number} size star大小
* @param {Object} style star样式
* Example: style = {
* borderColor:"#21DEEF",
* fillColor:"#21DEEF",
* spaceColor:"#FFFFFF"
* }
* @return none
*/
function showRatingStars(D_myCanvas, rating, counts, size, style) {
// 检测rating与star数目是否合适
if (rating > 2*counts) {
alert("Please set suitable rating and counts!");
return;
}
// 检测大小设置是否合适
if (D_myCanvas.offsetWidth < size * counts || D_myCanvas.offsetHeight < size) {
alert("Please set suitable size and D_myCanvas' size!");
return;
}
var context = D_myCanvas.getContext('2d');
var xStart = rating * size;
var yStart = 0;
var xEnd = (Math.ceil(rating) + 1) * size;
var yEnd = 0;
var radius = size / 2;
// 线性渐变,由左至右
var linear = context.createLinearGradient(xStart, yStart, xEnd, yEnd);
linear.addColorStop(0, style.fillColor);
linear.addColorStop(0.01, style.spaceColor);
linear.addColorStop(1, style.spaceColor);
context.fillStyle = linear;
// star边框颜色设置
context.strokeStyle = style.borderColor;
context.lineWidth = 1;
// 绘制star的顶点坐标
var x = radius,
y = 0;
for (var i = 0; i < counts; i++) {
// star绘制
context.beginPath();
var x1 = size * Math.sin(Math.PI / 10);
var h1 = size * Math.cos(Math.PI / 10);
var x2 = radius;
var h2 = radius * Math.tan(Math.PI / 5);
context.lineTo(x + x1, y + h1);
context.lineTo(x - radius, y + h2);
context.lineTo(x + radius, y + h2);
context.lineTo(x - x1, y + h1);
context.lineTo(x - x1, y + h1);
context.lineTo(x, y);
context.closePath();
context.stroke();
context.fill();
x = (i + 1.5) * size;
y = 0;
context.moveTo(x, y);
}
}
// 参数设置与函数调用
var size = 20;
var rating = 9.7;
var counts = 5;
var style = {
borderColor: "#FFFFFF",
fillColor: "#FFD700",
spaceColor: "#D5D5D5"
};
var D_myCanvas = document.getElementById("D_myCanvas");
showRatingStars(D_myCanvas, rating/2.0, counts, size, style);
</script>
<!--腾讯视频评分-->
<script>
/**
* showRatingStars 显示评分星级
* @param {Object} myCanvas 画布对象
* @param {Number} rating 评分
* @param {Number} counts star个数
* @param {Number} size star大小
* @param {Object} style star样式
* Example: style = {
* borderColor:"#21DEEF",
* fillColor:"#21DEEF",
* spaceColor:"#FFFFFF"
* }
* @return none
*/
function showRatingStars(T_myCanvas, rating, counts, size, style) {
// 检测rating与star数目是否合适
if (rating > 2*counts) {
alert("Please set suitable rating and counts!");
return;
}
// 检测大小设置是否合适
if (T_myCanvas.offsetWidth < size * counts || T_myCanvas.offsetHeight < size) {
alert("Please set suitable size and T_myCanvas' size!");
return;
}
var context = T_myCanvas.getContext('2d');
var xStart = rating * size;
var yStart = 0;
var xEnd = (Math.ceil(rating) + 1) * size;
var yEnd = 0;
var radius = size / 2;
// 线性渐变,由左至右
var linear = context.createLinearGradient(xStart, yStart, xEnd, yEnd);
linear.addColorStop(0, style.fillColor);
linear.addColorStop(0.01, style.spaceColor);
linear.addColorStop(1, style.spaceColor);
context.fillStyle = linear;
// star边框颜色设置
context.strokeStyle = style.borderColor;
context.lineWidth = 1;
// 绘制star的顶点坐标
var x = radius,
y = 0;
for (var i = 0; i < counts; i++) {
// star绘制
context.beginPath();
var x1 = size * Math.sin(Math.PI / 10);
var h1 = size * Math.cos(Math.PI / 10);
var x2 = radius;
var h2 = radius * Math.tan(Math.PI / 5);
context.lineTo(x + x1, y + h1);
context.lineTo(x - radius, y + h2);
context.lineTo(x + radius, y + h2);
context.lineTo(x - x1, y + h1);
context.lineTo(x - x1, y + h1);
context.lineTo(x, y);
context.closePath();
context.stroke();
context.fill();
x = (i + 1.5) * size;
y = 0;
context.moveTo(x, y);
}
}
// 参数设置与函数调用
var size = 20;
var rating = 8.5;
var counts = 5;
var style = {
borderColor: "#FFFFFF",
fillColor: "#FFD700",
spaceColor: "#D5D5D5"
};
var T_myCanvas = document.getElementById("T_myCanvas");
showRatingStars(T_myCanvas, rating/2.0, counts, size, style);
</script>
<!--爱奇艺评分-->
<script>
/**
* showRatingStars 显示评分星级
* @param {Object} myCanvas 画布对象
* @param {Number} rating 评分
* @param {Number} counts star个数
* @param {Number} size star大小
* @param {Object} style star样式
* Example: style = {
* borderColor:"#21DEEF",
* fillColor:"#21DEEF",
* spaceColor:"#FFFFFF"
* }
* @return none
*/
function showRatingStars(A_myCanvas, rating, counts, size, style) {
// 检测rating与star数目是否合适
if (rating > 2*counts) {
alert("Please set suitable rating and counts!");
return;
}
// 检测大小设置是否合适
if (A_myCanvas.offsetWidth < size * counts || A_myCanvas.offsetHeight < size) {
alert("Please set suitable size and A_myCanvas' size!");
return;
}
var context = A_myCanvas.getContext('2d');
var xStart = rating * size;
var yStart = 0;
var xEnd = (Math.ceil(rating) + 1) * size;
var yEnd = 0;
var radius = size / 2;
// 线性渐变,由左至右
var linear = context.createLinearGradient(xStart, yStart, xEnd, yEnd);
linear.addColorStop(0, style.fillColor);
linear.addColorStop(0.01, style.spaceColor);
linear.addColorStop(1, style.spaceColor);
context.fillStyle = linear;
// star边框颜色设置
context.strokeStyle = style.borderColor;
context.lineWidth = 1;
// 绘制star的顶点坐标
var x = radius,
y = 0;
for (var i = 0; i < counts; i++) {
// star绘制
context.beginPath();
var x1 = size * Math.sin(Math.PI / 10);
var h1 = size * Math.cos(Math.PI / 10);
var x2 = radius;
var h2 = radius * Math.tan(Math.PI / 5);
context.lineTo(x + x1, y + h1);
context.lineTo(x - radius, y + h2);
context.lineTo(x + radius, y + h2);
context.lineTo(x - x1, y + h1);
context.lineTo(x - x1, y + h1);
context.lineTo(x, y);
context.closePath();
context.stroke();
context.fill();
x = (i + 1.5) * size;
y = 0;
context.moveTo(x, y);
}
}
// 参数设置与函数调用
var size = 20;
var rating = 6;
var counts = 5;
var style = {
borderColor: "#FFFFFF",
fillColor: "#FFD700",
spaceColor: "#D5D5D5"
};
var A_myCanvas = document.getElementById("A_myCanvas");
showRatingStars(A_myCanvas, rating/2.0, counts, size, style);
</script>
<!--IMDB评分-->
<script>
/**
* showRatingStars 显示评分星级
* @param {Object} myCanvas 画布对象
* @param {Number} rating 评分
* @param {Number} counts star个数
* @param {Number} size star大小
* @param {Object} style star样式
* Example: style = {
* borderColor:"#21DEEF",
* fillColor:"#21DEEF",
* spaceColor:"#FFFFFF"
* }
* @return none
*/
function showRatingStars(I_myCanvas, rating, counts, size, style) {
// 检测rating与star数目是否合适
if (rating > 2*counts) {
alert("Please set suitable rating and counts!");
return;
}
// 检测大小设置是否合适
if (I_myCanvas.offsetWidth < size * counts || I_myCanvas.offsetHeight < size) {
alert("Please set suitable size and I_myCanvas' size!");
return;
}
var context = I_myCanvas.getContext('2d');
var xStart = rating * size;
var yStart = 0;
var xEnd = (Math.ceil(rating) + 1) * size;
var yEnd = 0;
var radius = size / 2;
// 线性渐变,由左至右
var linear = context.createLinearGradient(xStart, yStart, xEnd, yEnd);
linear.addColorStop(0, style.fillColor);
linear.addColorStop(0.01, style.spaceColor);
linear.addColorStop(1, style.spaceColor);
context.fillStyle = linear;
// star边框颜色设置
context.strokeStyle = style.borderColor;
context.lineWidth = 1;
// 绘制star的顶点坐标
var x = radius,
y = 0;
for (var i = 0; i < counts; i++) {
// star绘制
context.beginPath();
var x1 = size * Math.sin(Math.PI / 10);
var h1 = size * Math.cos(Math.PI / 10);
var x2 = radius;
var h2 = radius * Math.tan(Math.PI / 5);
context.lineTo(x + x1, y + h1);
context.lineTo(x - radius, y + h2);
context.lineTo(x + radius, y + h2);
context.lineTo(x - x1, y + h1);
context.lineTo(x - x1, y + h1);
context.lineTo(x, y);
context.closePath();
context.stroke();
context.fill();
x = (i + 1.5) * size;
y = 0;
context.moveTo(x, y);
}
}
// 参数设置与函数调用
var size = 20;
var rating = 7.5;
var counts = 5;
var style = {
borderColor: "#FFFFFF",
fillColor: "#FFD700",
spaceColor: "#D5D5D5"
};
var I_myCanvas = document.getElementById("I_myCanvas");
showRatingStars(I_myCanvas, rating/2.0, counts, size, style);
</script>
效果截图:
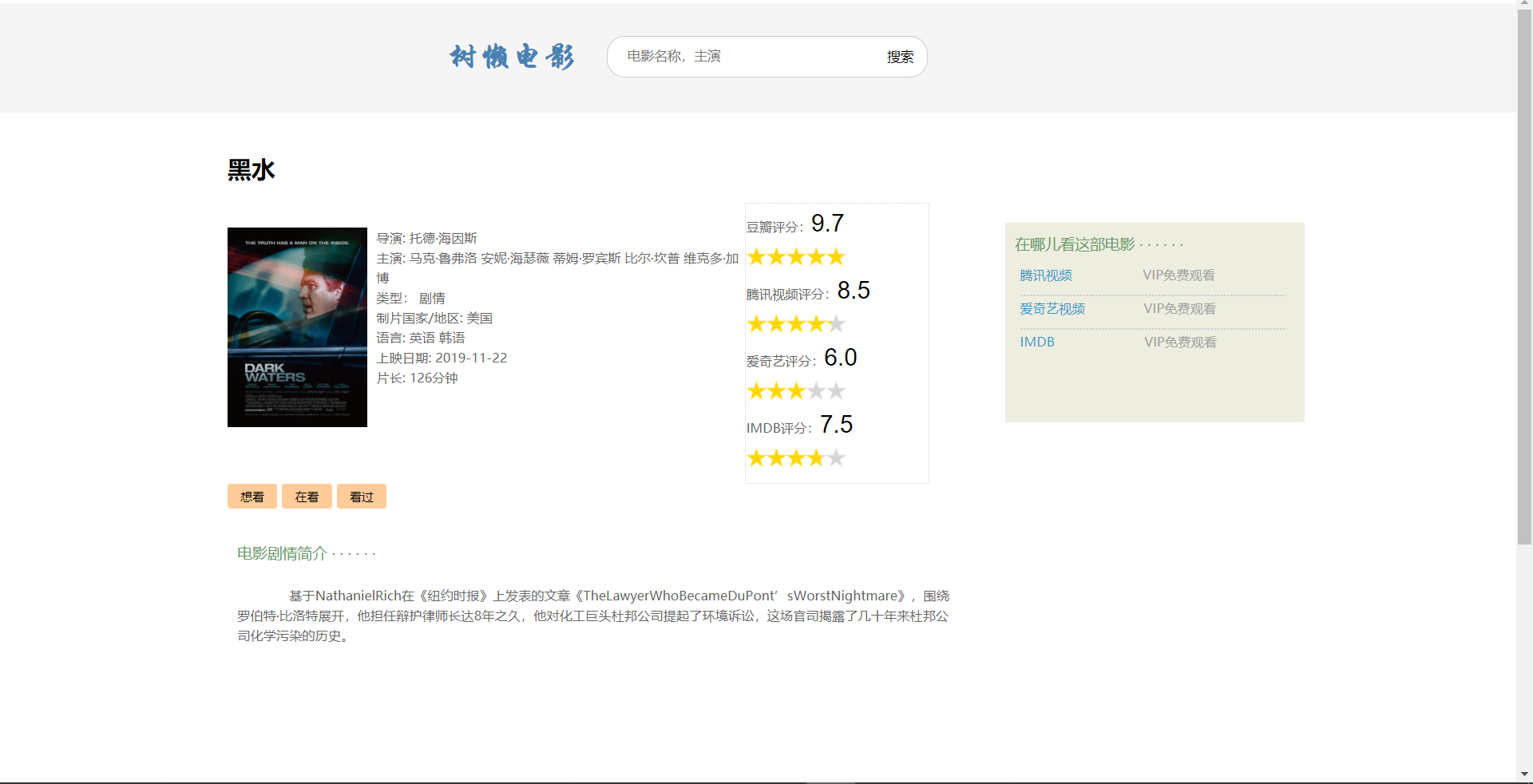