给出一个数组,数组中的元素各不相同,找到该集合的所有子集(包括空集和本身)
举例说明:
int []nums={1,2,3}
返回结果如下:
[ [3], [1], [2], [1,2,3], [1,3], [2,3], [1,2], [] ]
使用回溯法解决上述问题。
相当于对一棵树的深度遍历操作。
上述的输出结果还是有些规律的,也就是按照子集的元素个数来进行分解,元素个数为0,元素个数为1,元素个数为2,元素个数为3.
list.add(new ArrayList<>(tempList)); for(int i = startLen ; i < len ; i++){ tempList.add(nums[i]); getSubset(list,tempList,i+1,nums,len); tempList.remove(tempList.size()-1); }
关键代码段如上所示。为了以后遇到这样的题目更顺手,把这道题举一个比较详细的计算过程
private static void getSubset(List<List<Integer>> list, List<Integer> tempList, int startLen, int[] nums, int len)
上面为函数体。
其中第一个参数类型为List<List<Integer>> list用来保存所有的子集,作为最终的输出结果。
第二个参数为List<Integer> tempList用来记录某一个子集,
第三个参数为int startLen 用来标记开始的长度
第四个参数为int[] nums也就是最原始的集合
第五个参数为int len 表示数组nums的长度,可以避免在循环体中不断对数组nums进行求取长度。
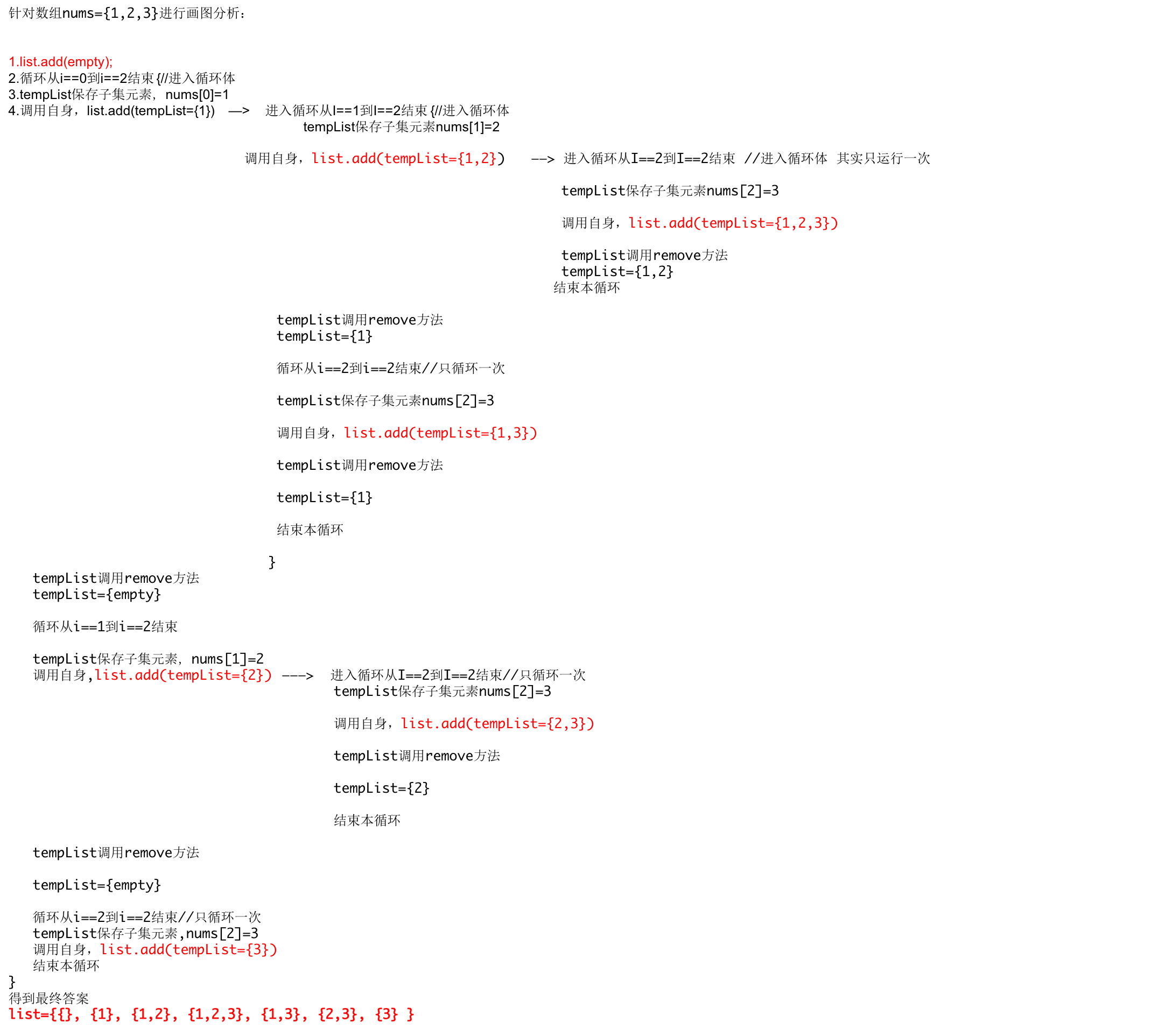
参考代码
package leetcode_100; import java.util.ArrayList; import java.util.Arrays; import java.util.List; /*** * * @author pengfei_zheng * 求集合的所有子集 */ public class Solution78 { public static List<List<Integer>> subsets(int[] nums) { List<List<Integer>> list = new ArrayList<>();//record the final answer List<Integer> tempList = new ArrayList<>();//record one of the subSet Arrays.sort(nums); int len = nums.length;//prevent calculating the length in the function getSubset(list, tempList, 0, nums, len);//calling the backtrack function return list; } private static void getSubset(List<List<Integer>> list, List<Integer> tempList, int startLen, int[] nums, int len) { list.add(new ArrayList<>(tempList));//by calling itself to add tempList to the list for(int i = startLen ; i < len ; i++){ tempList.add(nums[i]);// add element to tempList getSubset(list,tempList,i+1,nums,len);//calling itself tempList.remove(tempList.size()-1);//backtrack and remove the top element in tempList } } public static void main(String[]args){ int []nums = {0,1,2,3}; List<List<Integer>> list = subsets(nums); int len = list.size(); for(int i = 0 ; i < len; i++){ System.out.println(list.get(i)); } } }