AbstractList
1 类图
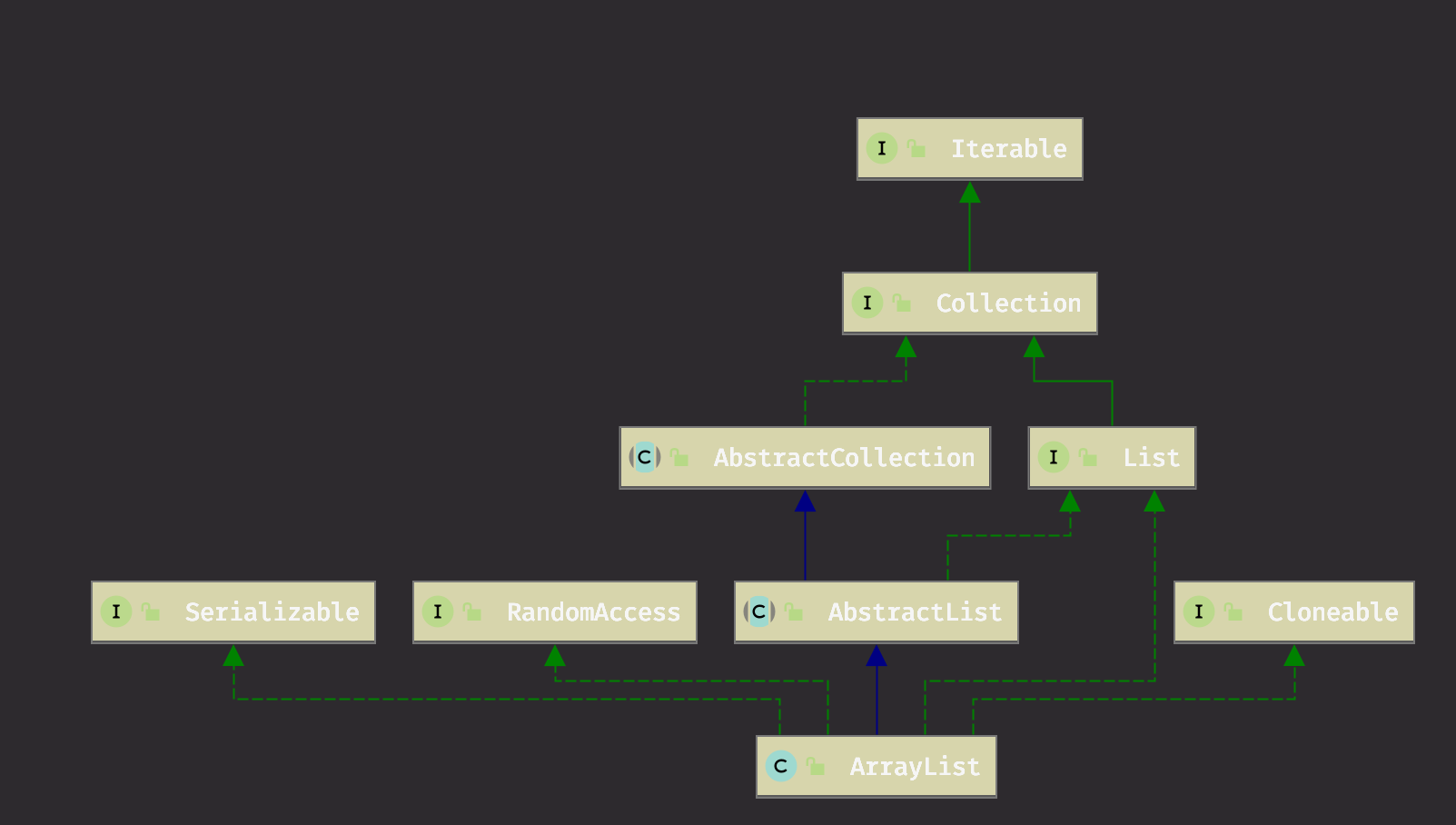
2 字段
// 默认容量
private static final int DEFAULT_CAPACITY = 10;
// 共享的空数组
private static final Object[] EMPTY_ELEMENTDATA = {};
// 共享个空数组,和EMPTY_ELEMENTDATA空数组相比是用于了解当添加第一个元素时数组应该扩容多少
private static final Object[] DEFAULTCAPACITY_EMPTY_ELEMENTDATA = {};
/**
* ArrayList存储元素的buffer, ArrayList的容量就是这个数组buffer的长度
* 任何拥有DEFAULTCAPACITY_EMPTY_ELEMENTDATA空数组的ArrayList在第一次
* 添加元素的时候都会扩容到DEFAULT_CAPACITY = 10
*/
transient Object[] elementData; // non-private to simplify nested class access
// 持有元素的个数
private int size;
3 构造器
/**
* 无参数的ArrayList的元素数组是DEFAULTCAPACITY_EMPTY_ELEMENTDATA
*/
public ArrayList() {
this.elementData = DEFAULTCAPACITY_EMPTY_ELEMENTDATA;
}
/**
* 初始容量为0的ArrayList的元素数组是EMPTY_ELEMENTDAT
*/
public ArrayList(int initialCapacity) {
if (initialCapacity > 0) {
this.elementData = new Object[initialCapacity];
} else if (initialCapacity == 0) {
this.elementData = EMPTY_ELEMENTDATA;
} else {
throw new IllegalArgumentException("Illegal Capacity: "+
initialCapacity);
}
}
/**
* 根据collection的迭代器返回的数据顺序构造一个ArrayList
*/
public ArrayList(Collection<? extends E> c) {
elementData = c.toArray();
if ((size = elementData.length) != 0) {
if (elementData.getClass() != Object[].class)
elementData = Arrays.copyOf(elementData, size, Object[].class);
} else {
this.elementData = EMPTY_ELEMENTDATA;
}
}
4 增
/**
* 往列表末尾增加元素
*/
public boolean add(E e) {
ensureCapacityInternal(size + 1); // Increments modCount!!
elementData[size++] = e;
return true;
}
- 先内部确认容量
- 数组buffer末尾添加元素
- 返回true
// 最小容量是当前size+1
private void ensureCapacityInternal(int minCapacity) {
// 如果当前的元素数组是DEFAULTCAPACITY_EMPTY_ELEMENTDATA
// 那么最小容量取min(DEFAULT_CAPACITY, size+1)
// 否则直接取size+1
// 可以认为如果指定了容量,那么对容量的预期越低吗?
if (elementData == DEFAULTCAPACITY_EMPTY_ELEMENTDATA) {
minCapacity = Math.max(DEFAULT_CAPACITY, minCapacity);
}
ensureExplicitCapacity(minCapacity);
}
// 确认容量的核心方法,然后扩容
private void ensureExplicitCapacity(int minCapacity) {
// 修改次数++
modCount++;
// 如果最小容量大于当前数组buffer的长度,那么需要扩容的
if (minCapacity - elementData.length > 0)
grow(minCapacity);
}
/**
* 最大数组长度
*/
private static final int MAX_ARRAY_SIZE = Integer.MAX_VALUE - 8;
/**
* 扩容,最起码可以容纳minCapacity个元素
*/
private void grow(int minCapacity) {
int oldCapacity = elementData.length;
// 新容量是老容量1.5倍
int newCapacity = oldCapacity + (oldCapacity >> 1);
// 取minCapacity和newCapacity的更小值
if (newCapacity - minCapacity < 0)
newCapacity = minCapacity;
// 如果大于最大数组长度,再处理
if (newCapacity - MAX_ARRAY_SIZE > 0)
newCapacity = hugeCapacity(minCapacity);
// minCapacity is usually close to size, so this is a win:
elementData = Arrays.copyOf(elementData, newCapacity);
}
// 溢出则抛出错误
// 如果大于MAX_ARRAY_SIZE,取Integer最大值,否则取MAX_ARRAY_SIZE
private static int hugeCapacity(int minCapacity) {
if (minCapacity < 0) // overflow
throw new OutOfMemoryError();
return (minCapacity > MAX_ARRAY_SIZE) ?
Integer.MAX_VALUE :
MAX_ARRAY_SIZE;
}
5 删除
/**
* 删除指定位置的元素,剩余右边元素都需要向左边移动一位
*/
public E remove(int index) {
rangeCheck(index);
// 修改次数+1
modCount++;
E oldValue = elementData(index);
int numMoved = size - index - 1;
if (numMoved > 0)
System.arraycopy(elementData, index+1, elementData, index, numMoved);
elementData[--size] = null;
return oldValue;
}
/**
* 索引边界检查
*/
private void rangeCheck(int index) {
if (index >= size)
throw new IndexOutOfBoundsException(outOfBoundsMsg(index));
}
7 更新
/**
* 替换指定位置上的元素
*/
public E set(int index, E element) {
rangeCheck(index);
E oldValue = elementData(index);
elementData[index] = element;
return oldValue;
}
8 查询
/**
* 返回指定位置元素
*/
public E get(int index) {
rangeCheck(index);
return elementData(index);
}
/**
* 返回指定元素第一次出现的索引,如果没有返回-1
*/
public int indexOf(Object o) {
if (o == null) {
for (int i = 0; i < size; i++)
if (elementData[i]==null)
return i;
} else {
for (int i = 0; i < size; i++)
if (o.equals(elementData[i]))
return i;
}
return -1;
}
9 遍历
/**
* 返回一个迭代器
*/
public Iterator<E> iterator() {
return new Itr();
}
private class Itr implements Iterator<E> {
int cursor; // 下一个返回的元素的索引
int lastRet = -1; // 上一个返回的元素的索引,如果没有则返回-1
int expectedModCount = modCount; // 期望的修改数目以及实际的修改数目
public boolean hasNext() {
return cursor != size;
}
// 如果实际修改次数和期望修改次数不相等则抛出ConcurrentModificationException
final void checkForComodification() {
if (modCount != expectedModCount)
throw new ConcurrentModificationException();
}
@SuppressWarnings("unchecked")
public E next() {
checkForComodification();
int i = cursor;
if (i >= size)
throw new NoSuchElementException();
Object[] elementData = ArrayList.this.elementData;
if (i >= elementData.length)
throw new ConcurrentModificationException();
// 往后遍历,游标+1
cursor = i + 1;
// 上一个返回的元素的索引是i
return (E) elementData[lastRet = i];
}
public void remove() {
if (lastRet < 0)
throw new IllegalStateException();
checkForComodification();
try {
// 调用ArrayList.remove,modCount++
ArrayList.this.remove(lastRet);
// 下一个游标还是当前元素,比如说上一个索引0,数字2
// 下一个索引1,数字3,当前游标是1
// 删除2后,游标变成0,还会返回3
// 那么上一个返回的元素就没有了,索引置-1
cursor = lastRet;
lastRet = -1;
// 这里把expectedModCount设置为删除后的modCount
expectedModCount = modCount;
} catch (IndexOutOfBoundsException ex) {
throw new ConcurrentModificationException();
}
}
@Override
@SuppressWarnings("unchecked")
public void forEachRemaining(Consumer<? super E> consumer) {
Objects.requireNonNull(consumer);
final int size = ArrayList.this.size;
int i = cursor;
if (i >= size) {
return;
}
final Object[] elementData = ArrayList.this.elementData;
if (i >= elementData.length) {
throw new ConcurrentModificationException();
}
while (i != size && modCount == expectedModCount) {
consumer.accept((E) elementData[i++]);
}
// update once at end of iteration to reduce heap write traffic
cursor = i;
lastRet = i - 1;
checkForComodification();
}
}
/**
* An optimized version of AbstractList.ListItr
*/
private class ListItr extends Itr implements ListIterator<E> {
ListItr(int index) {
super();
cursor = index;
}
public boolean hasPrevious() {
return cursor != 0;
}
public int nextIndex() {
return cursor;
}
public int previousIndex() {
return cursor - 1;
}
// 可以向前遍历
@SuppressWarnings("unchecked")
public E previous() {
checkForComodification();
int i = cursor - 1;
if (i < 0)
throw new NoSuchElementException();
Object[] elementData = ArrayList.this.elementData;
if (i >= elementData.length)
throw new ConcurrentModificationException();
cursor = i;
return (E) elementData[lastRet = i];
}
public void set(E e) {
if (lastRet < 0)
throw new IllegalStateException();
checkForComodification();
try {
ArrayList.this.set(lastRet, e);
} catch (IndexOutOfBoundsException ex) {
throw new ConcurrentModificationException();
}
}
public void add(E e) {
checkForComodification();
try {
int i = cursor;
ArrayList.this.add(i, e);
cursor = i + 1;
lastRet = -1;
expectedModCount = modCount;
} catch (IndexOutOfBoundsException ex) {
throw new ConcurrentModificationException();
}
}
}