/*
生产者,消费者。
多生产者,多消费者的问题。
if判断标记,只有一次,会导致不该运行的线程运行了。出现了数据错误的情况。
while判断标记,解决了线程获取执行权后,是否要运行!
notify:只能唤醒一个线程,如果本方唤醒了本方,没有意义。而且while判断标记+notify会导致死锁。
notifyAll解决了本方线程一定会唤醒对方线程的问题。
*/
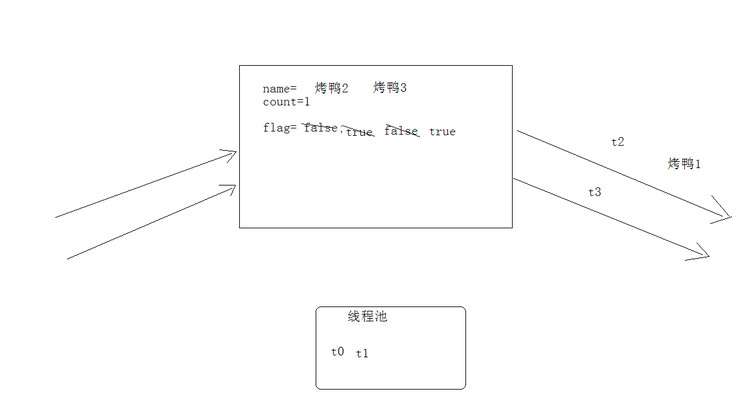
Object obj = new Object(); void show() { synchronized(obj) //同步代码块,对于锁的操作是隐式的。 { code...... } } Lock lock = new ReentrantLock(); //JDK1.5 以后将同步和锁封装成对象; //并将操作锁的隐式方式定义到对象中,将 //隐式动作变成显示动作。 void show() { 随着灵活性的增加,也带来了更多的责任。不使用块结构锁就失去了使用 synchronized 方法和语句时会出现的锁自动释放功能。在大多数情况下,应该使用以下语句: Lock l = ...; l.lock(); try { // access the resource protected by this lock } finally { l.unlock(); } }
Example1
class Resource { private String name; private int count = 1; private boolean flag = false; public synchronized void set(String name)// { while(flag) try{this.wait();}catch(InterruptedException e){}// t1 t0 this.name = name + count;//烤鸭1 烤鸭2 烤鸭3 count++;//2 3 4 System.out.println(Thread.currentThread().getName()+"...生产者..."+this.name);//生产烤鸭1 生产烤鸭2 生产烤鸭3 flag = true; notifyAll(); } public synchronized void out()// t3 { while(!flag) try{this.wait();}catch(InterruptedException e){} //t2 t3 System.out.println(Thread.currentThread().getName()+"...消费者........"+this.name);//消费烤鸭1 flag = false; notifyAll(); } } class Producer implements Runnable { private Resource r; Producer(Resource r) { this.r = r; } public void run() { while(true) { r.set("烤鸭"); } } } class Consumer implements Runnable { private Resource r; Consumer(Resource r) { this.r = r; } public void run() { while(true) { r.out(); } } } class ProducerConsumerDemo { public static void main(String[] args) { Resource r = new Resource(); Producer pro = new Producer(r); Consumer con = new Consumer(r); Thread t0 = new Thread(pro); Thread t1 = new Thread(pro); Thread t2 = new Thread(con); Thread t3 = new Thread(con); t0.start(); t1.start(); t2.start(); t3.start(); } }
Output:
Thread-3...消费者........烤鸭55643
Thread-1...生产者...烤鸭55644
Thread-2...消费者........烤鸭55644
Thread-0...生产者...烤鸭55645
Thread-3...消费者........烤鸭55645
Thread-1...生产者...烤鸭55646
Thread-2...消费者........烤鸭55646
Thread-0...生产者...烤鸭55647
Thread-3...消费者........烤鸭55647
Thread-1...生产者...烤鸭55648
Thread-2...消费者........烤鸭55648
Thread-0...生产者...烤鸭55649
Thread-3...消费者........烤鸭55649
Thread-1...生产者...烤鸭55650