配合ASP.NET 2.0提供了FileUpload控件,很容易就可以完成文件上传功能。做了一个简单的上传逻辑,实现了:检查了文件是否存在、自动生成文件名、自动建立上传文件夹、对文件类型作校验等功能。
1
using System;
2
using System.Collections.Generic;
3
using System.Text;
4
using System.Web;
5
using System.IO;
6
using System.Web.Configuration;
7
using System.Configuration;
8
using System.Web.Security;
9
using System.Web.UI.WebControls;
10
namespace QmxMovieBLL
11
12
{
13
public class UploadFileBLL
14
{
15
string filePath = ConfigurationManager.AppSettings["UploadImages"].ToString();
16
private string GenerateRefUploadFileName(string ext)
17
{
18
string fileName;//初始文件名
19
string hashedFileName;//加密过的文件名
20
string refUploadDest;//最终上传地址(映射过的)
21
int count = 0;
22
do
23
{
24
if(count++>=32767) throw new Exception("生成文件名失败,请重新进行此操作!");//防止死循环
25
fileName = DateTime.Now.ToString() + DateTime.Now.Millisecond.ToString();
26
hashedFileName = FormsAuthentication.HashPasswordForStoringInConfigFile(fileName, "MD5");
27
refUploadDest =Path.Combine(filePath, hashedFileName);
28
} while (File.Exists(HttpContext.Current.Server.MapPath(refUploadDest)));
29
return refUploadDest+ext;
30
}
31
32
private bool CanUpload(string ext)
33
{
34
//TODO:是否合法的扩展名
35
//允许上传的图片格式为jpg/jpeg、png和gif类型
36
if (ext.ToLower() == ".jpg" || ext.ToLower() == ".jpeg" || ext.ToLower() == ".png"
37
|| ext.ToLower() == ".gif")
38
{
39
return true;
40
}
41
return false;
42
}
43
44
private bool FileTypeAllowed(FileUpload fu)
45
{
46
//是否合法的文件类型,通过FileUpload的ContentType属性来确定类型
47
string fileType = fu.PostedFile.ContentType.ToString().ToLower();
48
if (fileType == "image/pjpeg" || fileType == "image/x-png" || fileType == "image/gif") return true;
49
return false;
50
51
}
52
53
public string UploadFile(FileUpload fu)
54
{
55
if (fu.HasFile)
56
{
57
string fileName = fu.FileName;
58
//Response.Write(fu.PostedFile.ContentType.ToString().ToLower());
59
string ext = Path.GetExtension(fileName).ToLower();
60
//首先,验证是否可以上传该文件
61
if (!CanUpload(ext)||!(FileTypeAllowed(fu)))
62
{
63
throw new Exception("不是允许的上传文件类型!");
64
}
65
66
if (!Directory.Exists(HttpContext.Current.Server.MapPath(filePath)))
67
{
68
Directory.CreateDirectory(HttpContext.Current.Server.MapPath(filePath));
69
}
70
71
string refDest;
72
try
73
{
74
refDest = GenerateRefUploadFileName(ext);
75
}
76
catch (Exception ex)
77
{
78
throw ex;
79
}
80
string realDest = HttpContext.Current.Server.MapPath(refDest);
81
try
82
{
83
fu.SaveAs(realDest);
84
}
85
catch (Exception)
86
{
87
throw new Exception("文件保存出错。请检查上传文件夹是否存在。");
88
}
89
return refDest;
90
}
91
else
92
{
93
throw new Exception("请选择要上传的文件。");
94
}
95
}
96
97
}
98
}
/// <summary>
/// 检查文件的大小是否合法
/// </summary>
/// <param name="fu"></param>
/// <returns></returns>
private bool IsUploadSizeFit(FileUpload fu)
{
const int maxFileSize = 10;
if (fu.PostedFile.ContentLength > maxFileSize) return false;
return true;
}
//如果文件上传大小超过限制,则不允许上传
if (!IsUploadSizeFit(fu))
{
throw new Exception("文件大小超过允许范围!");
}
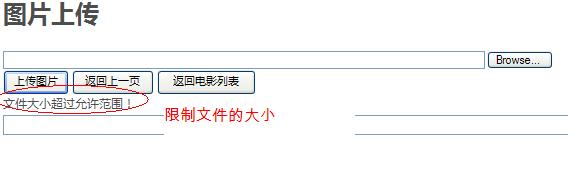
99

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

66

67

68

69

70

71

72

73

74

75

76

77

78

79

80

81

82

83

84

85

86

87

88

89

90

91

92

93

94

95

96

97

98

要实现这个可以用到FileUpload的ContentLength属性,此属性返回要上传文件的字节数。例如,可以写一个方法,设置测试大小限制为10字节:











在上文第65行位置插入如下代码:





完成的功能如下:
99
