Description: Given the root
of a complete binary tree, return the number of the nodes in the tree.
According to Wikipedia, every level, except possibly the last, is completely filled in a complete binary tree, and all nodes in the last level are as far left as possible. It can have between 1
and 2h
nodes inclusive at the last level h
.
Link: 222. Count Complete Tree Nodes
Examples:
Example 1:
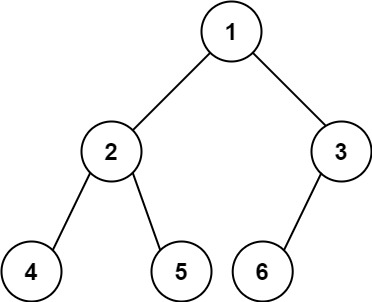
Input: root = [1,2,3,4,5,6] Output: 6 Example 2: Input: root = [] Output: 0 Example 3: Input: root = [1] Output: 1
思路: 从头遍历一次,O(n). 怎样才能更快呢?
class Solution(object):
def countNodes(self, root):
"""
:type root: TreeNode
:rtype: int
"""
if not root: return 0
return 1 + self.countNodes(root.left) + self.countNodes(root.right)
class Solution(object): def countNodes(self, root): """ :type root: TreeNode :rtype: int """ self.res = 0 if not root: return 0 self.res += 1 if root.left: self.res += self.countNodes(root.left) if root.right: self.res += self.countNodes(root.right) return self.res
深度为 h 的满二叉树,节点个数为 2 ^ h - 1. 完全二叉树的最后一层节点不一定满,从左到右填充。
首先计算左子树的深度l. 右子树的深度r; 如果 l == r,说明左子树是满二叉树,右子树是完全二叉树,总节点数 = 2 ** l -1 + 1 (root node) + countNodes(root.right)如果l > r,说明右子树是满二叉树,左子树是完全二叉树, 总节点数 = 2 ** r -1 + 1 (root node) + countNodes(root.left)。计算深度时,因为是完全二叉树,从左边排起,只要一直向下数做节点就好。
class Solution(object): def countNodes(self, root): """ :type root: TreeNode :rtype: int """ if not root: return 0 leftdepth = self.getdepth(root.left) rightdepth = self.getdepth(root.right) if leftdepth == rightdepth: return pow(2,leftdepth) + self.countNodes(root.right) else: return pow(2,rightdepth) + self.countNodes(root.left) def getdepth(self, root): depth = 0 while root: depth += 1 root = root.left return depth # def getdepth(self, root): # if not root: # return 0 # return 1 + self.getdepth(root.left)
Reference: https://blog.csdn.net/fuxuemingzhu/article/details/80781666
日期: 2021-04-15 生活总不会让人太难