题目描述
输入一个字符串,按字典序打印出该字符串中字符的所有排列。例如输入字符串abc,则按字典序打印出由字符a,b,c所能排列出来的所有字符串abc,acb,bac,bca,cab和cba。
输入描述:
输入一个字符串,长度不超过9(可能有字符重复),字符只包括大小写字母。
示例1
输入
"ab"
返回值
["ab","ba"]
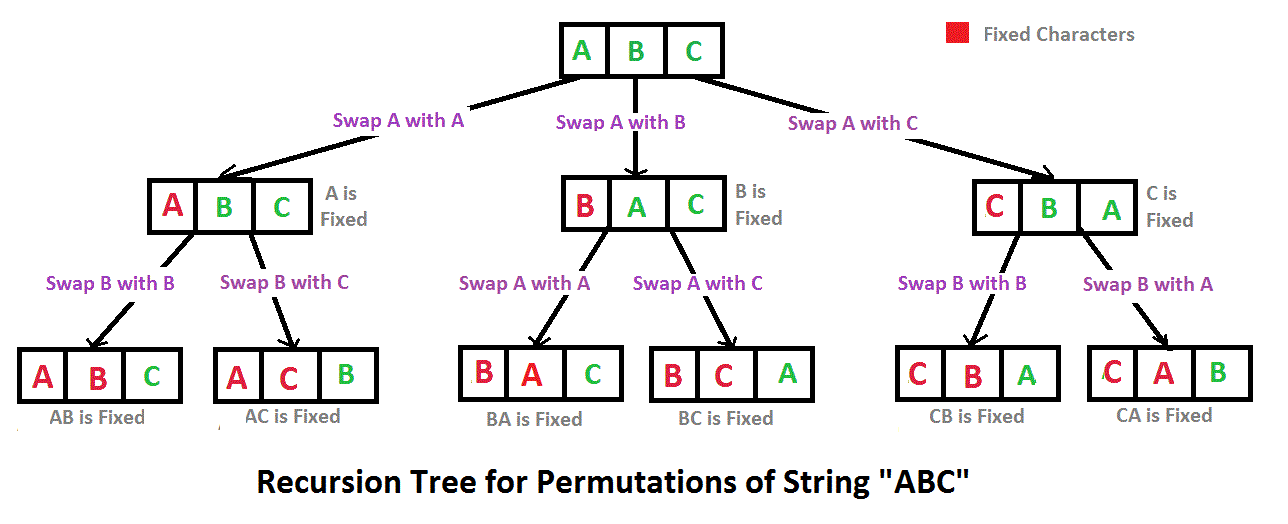
使用set完成去重和排序,使用assign完成将set集合中的数据拷贝到vector中。
class Solution { public: vector<string> Permutation(string str) { vector<string> result; if(str.length() == 0||str == "") return result; set<string> tempResult; rePermutation(0,tempResult,str); result.assign(tempResult.begin(), tempResult.end()); return result; } void rePermutation(int fixedWei,set<string> &result,string str){ if(fixedWei == str.length()-1){ result.insert(str); return; } char temp; for(int i=fixedWei;i<str.length();i++){ temp = str[fixedWei]; str[fixedWei] = str[i]; str[i] = temp; rePermutation(fixedWei+1,result,str); } } };
使用vector:
class Solution { public: vector<string> permutation(string s) { vector<string> result; if(s.length() == 0||s == "") return result; rePermutation(0,result,s); return result; } void rePermutation(int fixedWei,vector<string> &result,string str){ if(fixedWei == str.length()-1){ result.push_back(str); return; } char temp; set<char> charSet; for(int i=fixedWei;i<str.length();i++){ //判断该字符是否已经处理过,防止重复 if(i==fixedWei||charSet.find(str[i])==charSet.end()){ charSet.insert(str[i]); //交换 temp = str[fixedWei]; str[fixedWei] = str[i]; str[i] = temp; //递归 rePermutation(fixedWei+1,result,str); //恢复,防止影响下一次递归,因为从递归返回的是已经交换过的字符串 temp = str[fixedWei]; str[fixedWei] = str[i]; str[i] = temp; } } } };