Largest Rectangle in a Histogram
Time Limit : 2000/1000ms (Java/Other) Memory Limit : 65536/32768K (Java/Other)
Total Submission(s) : 16 Accepted Submission(s) : 6
Problem Description
A histogram is a polygon composed of a sequence of rectangles aligned at a common base line. The rectangles have equal widths but may have different heights. For example, the figure on the left shows the histogram that consists of rectangles with the heights 2, 1, 4, 5, 1, 3, 3, measured in units where 1 is the width of the rectangles:
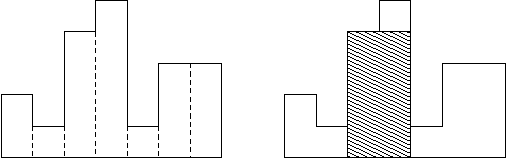
Usually, histograms are used to represent discrete distributions, e.g., the frequencies of characters in texts. Note that the order of the rectangles, i.e., their heights, is important. Calculate the area of the largest rectangle in a histogram that is aligned at the common base line, too. The figure on the right shows the largest aligned rectangle for the depicted histogram.
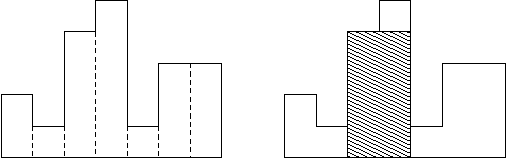
Usually, histograms are used to represent discrete distributions, e.g., the frequencies of characters in texts. Note that the order of the rectangles, i.e., their heights, is important. Calculate the area of the largest rectangle in a histogram that is aligned at the common base line, too. The figure on the right shows the largest aligned rectangle for the depicted histogram.
Input
The input contains several test cases. Each test case describes a histogram and starts with an integer n, denoting the number of rectangles it is composed of. You may assume that 1 <= n <= 100000. Then follow n integers h1, ..., hn, where 0 <= hi <= 1000000000. These numbers denote the heights of the rectangles of the histogram in left-to-right order. The width of each rectangle is 1. A zero follows the input for the last test case.
Output
For each test case output on a single line the area of the largest rectangle in the specified histogram. Remember that this rectangle must be aligned at the common base line.
Sample Input
7 2 1 4 5 1 3 3
4 1000 1000 1000 1000
0
4 1000 1000 1000 1000
0
Sample Output
8
4000
4000
一道用DP来做的题目:
对于条形图的每一条列,如果比前面的小,那么把他们放在一起肯定比单独算面积要大,右边如此!!
对于直方图的每一个右边界,穷举所有的左边界。将面积最大的那个值记录下来。时间复杂度为O(n^2). 单纯的穷举在LeetCode上面过大集合时会超时。可以通过选择合适的右边界,做一个剪枝(Pruning)。观察发现当height[k] >= height[k - 1]时,无论左边界是什么值,选择height[k]总会比选择height[k - 1]所形成的面积大。因此,在选择右边界的时候,首先找到一个height[k] < height[k - 1]的k,然后取k - 1作为右边界,穷举所有左边界,找最大面积。
1 #include<iostream> 2 #include<algorithm> 3 #include<string.h> 4 #include<stdio.h> 5 using namespace std; 6 int b[100005],c[100005]; 7 __int64 a[100005]; 8 int main() 9 { 10 int n,m,i; 11 __int64 max; 12 while(scanf("%I64d",&n)!=EOF&&n) 13 { 14 for(i=1;i<=n;i++) 15 scanf("%d",&a[i]); 16 for(i=1;i<=n;i++) 17 { 18 b[i]=i; 19 c[i]=i; 20 } 21 for(i=2;i<=n;i++) 22 { 23 m=i; 24 while(a[m-1]>=a[i]) 25 { 26 b[i]=b[m-1]; 27 m=b[m-1]; 28 if(m==1) 29 break; 30 } 31 } 32 for(i=n-1;i>=1;i--) 33 { 34 m=i; 35 while(a[m+1]>=a[i]) 36 { 37 c[i]=c[m+1]; 38 m=c[m+1]; 39 if(m==n) 40 break; 41 } 42 } 43 max=a[1]*(c[1]-b[1]+1); 44 for(i=2;i<=n;i++) 45 { 46 if(a[i]*(c[i]-b[i]+1)>=max) 47 max=a[i]*(c[i]-b[i]+1); 48 } 49 printf("%I64d ",max); 50 } 51 return 0; 52 }