"""
nms输入的数据为box的左上角x1,y1与右下角x2,y2+confidence,rows=batch_size,line=[x1,y1,x2,y2,confidence];
首先将batch_size按照已给的confidence从大到小排序,将最大confidence的box保存,而后与其它batch_size-1个boxes进行iou,
得到新的batch_size-1个iou(confidence),然后与阈值(threshold)相比较,保留满足阈值的boxes,
而后将保留后的boxes按照求解的confidence进行从大到小排序,保留最大box,以此类推,最终保留下来的为需要的boxes,
包含对应原来的confidence
其具体做法如下code。
"""
import numpy as np
boxes = np.array([
[204, 102, 358, 250, 0.5],
[257, 118, 380, 250, 0.7],
[280, 135, 400, 250, 0.6],
[255, 118, 360, 235, 0.7]])
box_threshold = 0.7
def NMS(BOXES, box_threshold):
"""Pure Python NMS baseline."""
x1 = BOXES[:, 0] # xmin
y1 = BOXES[:, 1] # ymin
x2 = BOXES[:, 2] # xmax
y2 = BOXES[:, 3] # ymax
confidence = BOXES[:, 4] # confidence
boxes_areas = (x2 - x1 + 1) * (y2 - y1 + 1) # the size of bbox
confidence_index = confidence.argsort()[::-1] # sort bounding boxes by decreasing order, returning array([3, 1, 2, 0])
save_boxes_end_index = [] # store the final bounding boxes of index
while confidence_index.size > 0: # 此行代码表示存在有置信度的box,才会运行。若只有一个box则下面将会和自己比较
i = confidence_index[0] # 永远取置信率最高的框作为物体的最佳框
save_boxes_end_index.append(i) # 第一次运行保存置信度最大box的索引值
# 获取两个框相交矩形的坐标
# 左上角坐标取二者最大;右下角取二者最小
xx1 = np.maximum(x1[i], x1[confidence_index[1:]]) # array([ 257., 280., 255.]) 保存相交区域左上角x
yy1 = np.maximum(y1[i], y1[confidence_index[1:]]) # array([ 118., 135., 118.]) 保存相交区域左上角y
xx2 = np.minimum(x2[i], x2[confidence_index[1:]]) # array([ 360., 360., 358.]) 保存相交区域右下角x
yy2 = np.minimum(y2[i], y2[confidence_index[1:]]) # array([ 235., 235., 235.]) 保存相交区域右下角y
w = np.maximum(0.0, xx2 - xx1 + 1) # array([ 104., 81., 104.])
h = np.maximum(0.0, yy2 - yy1 + 1) # array([ 118., 101., 118.])
# 计算相交矩形面积
boxes_cross_areas = w * h # array([ 12272., 8181., 12272.])
# 计算IOU
# Cross Area / (bbox + particular area - Cross Area)
boxes_iou = boxes_cross_areas / (boxes_areas[i] + boxes_areas[confidence_index[1:]] - boxes_cross_areas)
# reserve all the bounding box whose ovr less than thresh
confidence_process_index = np.where(boxes_iou <= box_threshold)[0] # 返回boxes_iou中小于box_threshold的索引值
# 与置信度最大的box比较,分别求解出其它boxes的iou值(是下次其它boxes置信度的值),而后只保留比例小于阙值的框,然后继续处理
confidence_index = confidence_index[confidence_process_index + 1] # 因为boxes_iou根据其它boxes与最大box得到一维矩阵,实际少了第一个box,因此confidence_process_index需要+1,而后得到新的confidence——index
boxes_end = []
for i in range(len(save_boxes_end_index)):
boxes_end.append(BOXES[save_boxes_end_index[i]])
boxes_end=np.array(boxes_end)
return boxes_end
boxes_end = NMS(boxes, box_threshold)
print(boxes_end)
其运行结果如下:
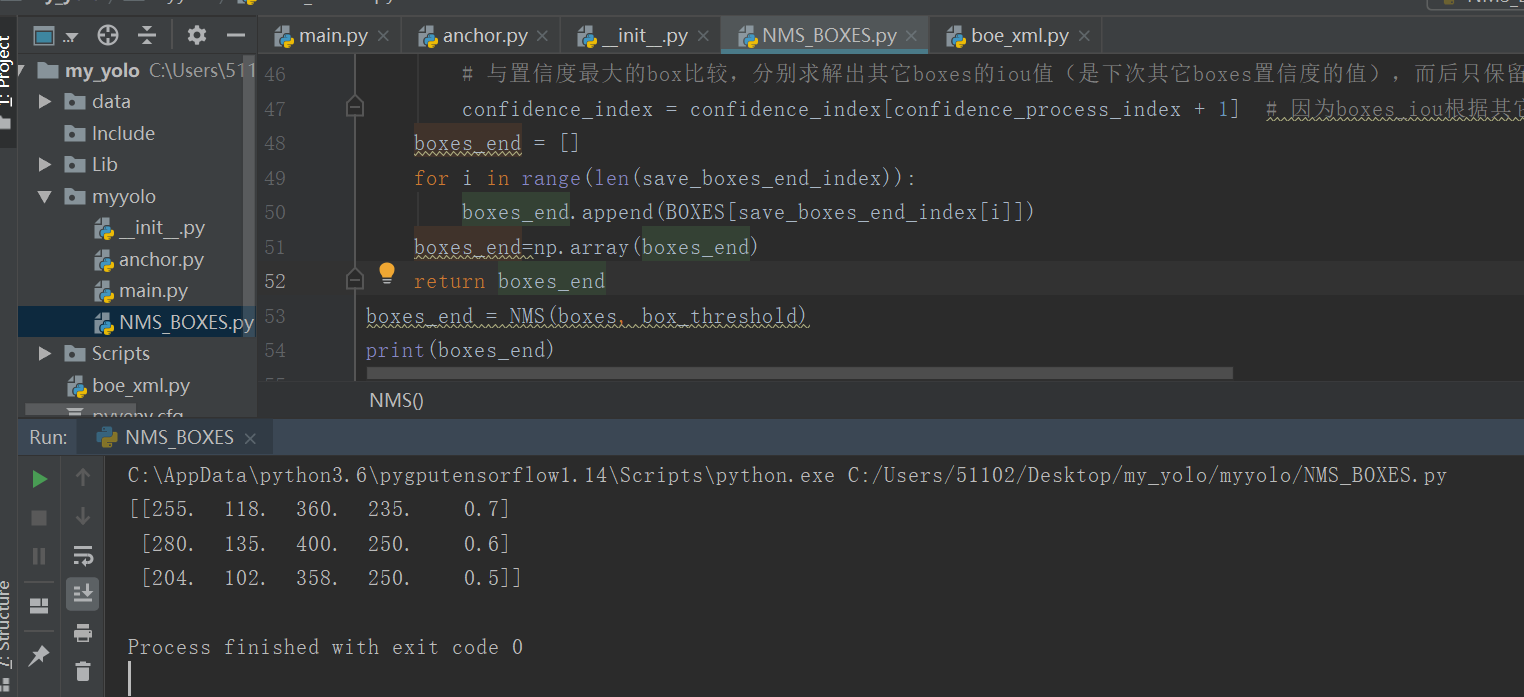