一、简单函数的结构
def greet_user():
'''问候用户'''
print(Hello,John!")
greet_user()
执行结果:
二、向函数传递信息
def greet_user(username):
'''问候用户'''
print(‘’Hello!"+username)
greet_user('jack')
执行结果:
三、多种参数的情况
1、位置实参:基于实参的顺序
eg :
def greet_user(username,city):
'''问候用户'''
print("Hello "+username)
print("He lived in "+city)
greet_user('jack','shanxi')
执行结果:
2、关键字实参
def greet_user(username,city):
'''问候用户'''
print("Hello "+username)
print("He lived in "+city)
greet_user(city='shanxi',username='zhangsan')
执行结果:
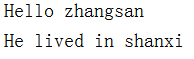
3、默认值
def greet_user(username,city='shanghai'):
'''问候用户'''
print("Hello "+username)
print("He lived in "+city)
greet_user('zhangsan')
执行结果:
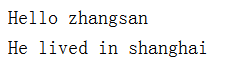
四、函数返回值
def greet_user(first_name,last_name):
'''问候用户'''
full_name = first_name+' '+last_name
return full_name
username = greet_user('zhang','san')
print(username)
注:调用返回值函数时,需要提供一个变量来存储返回的值。
执行结果:
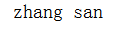
五、让实参变成可选
def greet_user(first_name,last_name,middle_name=''):
'''问候用户'''
if middle_name:
full_name = first_name + ' '+ middle_name +' '+ last_name
else:
full_name = first_name +' ' + last_name
return full_name
username = greet_user('zhang','san')
print(username)
username = greet_user('li','pang','san')
print(username)
注:实参可选时可以给它一个默认空值-空字符串,放在末尾。
执行结果:
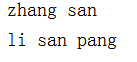
六、返回一个字典
def greet_user(first_name,last_name):
'''返回用户信息'''
person = {'first':first_name,'last':last_name}
return person
person_message = greet_user('zhang','san')
print(person_message)
执行结果:

七、返回的字典接受可选值
def greet_user(first_name,last_name,age=''):
'''返回用户信息'''
person = {'first':first_name,'last':last_name}
if age:
person['age'] = age
return person
person_message = greet_user('zhang','san',26)
print(person_message)
执行结果:

八、while循环和函数相结合
def greet_user(first_name,last_name):
'''返回用户信息'''
full_name = first_name + last_name
return full_name
while True:
f_name = input("请输入姓氏:按‘q’退出:")
if f_name == 'q':
break
l_name = input("请输入名字:按'q'退出:")
if l_name == 'q':
break
person_message = greet_user(f_name,l_name)
print("Hello, "+person_message)
执行结果:
九、向函数传递列表
def greet_user(username):
'''循环问候存在列表中的每一位用户'''
for user in username:
msg = "Hello,"+ user.title()
print(msg)
user_name = ['zhangsan','lili','lucy']
greet_user(user_name)
执行结果:
十、在函数中修改列表
def show_magicians(names):
"""显示魔术师列表信息"""
for magician in magicians:
print(magician)
def make_great(names):
"""修改魔术师列表,在每个名字前加The great """
length = len(magicians)
for i in range(1,length+1):
magicians[i-1]='the great '+magicians[i-1]
magicians = ['liuqian','zhangsan','lisi']
make_great(magicians)
show_magicians(magicians)
执行结果:
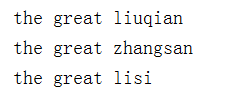
十一、传递任意数量的实参。函数名(括号里的形参加*)
def sandwich_toppings(*toppings):
"""显示顾客点的三明治的食材"""
print("Your sandwich with :")
for topping in toppings:
print(topping)
sandwich_toppings('hot dog')
sandwich_toppings('vegetable','fish','meal')
sandwich_toppings('onions','pepper chili')
执行结果:
十二、结合使用位置实参和任意数量实参(要让函数接受不同类型的实参,必须在函数定义中将接纳任意数量实参的形参放在最后。Python先匹配位置实参和关键字实参,再将余下的实参都收集到最后一个形参中)
def sandwich_toppings(size,*toppings):
"""显示顾客点的三明治的食材"""
print("Your " + str(size) + "-sandwich with :")
for topping in toppings:
print(topping)
sandwich_toppings(7,'hot dog')
sandwich_toppings(5,'vegetable','fish','meal')
sandwich_toppings(8,'onions','pepper chili')
执行结果:
十三、使用任意数量的关键字实参。(函数名括号里跟两个*号,将参数以键-值对的形式封装到字典中)
def build_profile(first_name,last_name,**info):
"""显示用户的一切信息"""
profile = {}
profile['first_name'] = first_name
profile['last_name'] = last_name
for key,value in info.items():
profile[key] = value
return profile
user_info = build_profile('jiang','yun',city='xian',sex='girl')
print(user_info)
执行结果:

十四、导入模块
1、导入整个模块
import modle_name
调用函数:modle_name.function_name()
2、调用特定的函数,多个函数时用逗号分隔
from modle_name import function_name1,function_name2
调用函数时无需使用句点加上模块名称:fuction_name()
3、使用as给函数指定别名
from modle_name import function_name as 别名
调用函数:别名()
4、使用as给模块指定别名
import modle_name as 别名
调用函数:别名.fuction_name()
5、导入模块中所有的函数
from modle_name import *
十五、函数的实参和形参编写规则
如果有默认值或关键字形参,注意等号两边不要有空格。