异常
概念
程序运行时,发生的不被期望的事件,它阻止了程序按照程序员的预期正常执行,这就是异常。
类型
- Error错误
- Exception异常
- 异常类的结构
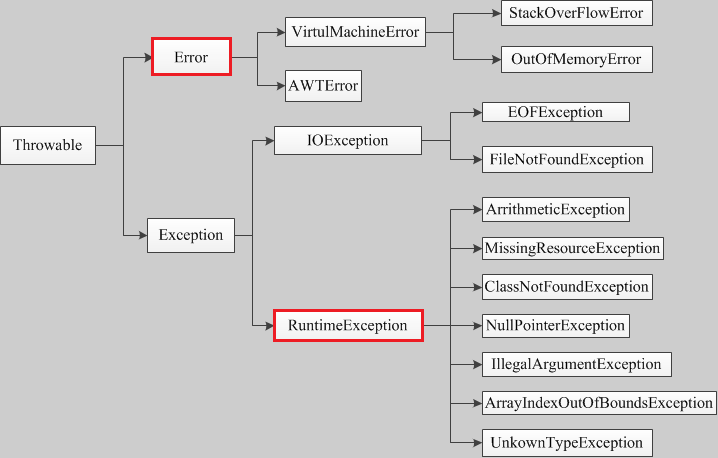
Throwable类是Java异常类型的顶层父类,一个对象只有是 Throwable 类的(直接或者间接)实例,他才是一个异常对象,才能被异常处理机制识别。JDK中内建了一些常用的异常类,我们也可以自定义异常
异常处理的方法
try catch finally throw throws
try-catch-finally
//异常就是程序运行时,发生了不被期望的事件,它阻碍了程序的正常运行。
public class Demo01 {
public static void main(String[] args) {
int a = 1;
int b = 0;
//快捷键:选中,ctr+alt+t
/**
* try:监控范围
* catch:捕捉异常
* finally:等try_catch结束后的后续操作
*/
try {
System.out.println(a/b);
}catch (ArithmeticException e) {
System.out.println("被除数不能为零!");
}catch (Exception e) {
System.out.println("Exception");
}catch (Throwable throwable){ //可以捕获多个异常,必须从小到大!
System.out.println("Throwable");
}finally {
System.out.println("finally");
}
}
}
throw
public class Demo02 {
public static void main(String[] args) {
int a = 1;
int b = 0;
//throw可以抛出异常
if (b==0){
throw new ArithmeticException();
}
System.out.println(a/b);
}
}
throws
public class Demo03 {
//throws方法异常向上抛出
//抛出后,在调用处用try-catch捕获异常
public void test (int a,int b)throws Exception{//向上抛出异常
System.out.println(a/b);
}
public static void main(String[] args) {
//调用时,捕获异常
try {
new Demo03().test(1,0);
} catch (Exception e) {
e.printStackTrace();
}
}
}
自定义异常
public class MyException extends Exception {
//自定义异常
private int detail;
public MyException(int a){
this.detail = a;
}
//打印异常信息
@Override
public String toString() {
return "MyException{" +
"detail=" + detail +
'}';
}
}
调用异常
public class Application {
//2.继续向上抛出
public void test(int a) throws MyException {
System.out.println("输入的参数为:"+a);
//1.如果大于10,抛出异常
if(a>10){
throw new MyException(a);
}else{
System.out.println("OK");
}
}
public static void main(String[] args) {
//3.调用时,try-catch捕获异常
try {
new Application().test(11);
} catch (MyException e) {
System.out.println("MyException==>"+e);
}
}
}