ES 服务器正常启动后,可以通过 Java API 客户端对象对 ES 索引进行操作
1 创建索引
package com.atguigu.es.test; import org.apache.http.HttpHost; import org.elasticsearch.client.RequestOptions; import org.elasticsearch.client.RestClient; import org.elasticsearch.client.RestHighLevelClient; import org.elasticsearch.client.indices.CreateIndexRequest; import org.elasticsearch.client.indices.CreateIndexResponse; public class ESTest_Index_Create { public static void main(String[] args) throws Exception { RestHighLevelClient esClient = new RestHighLevelClient( RestClient.builder(new HttpHost("localhost", 9200, "http")) ); // 创建索引 CreateIndexRequest request = new CreateIndexRequest("user"); CreateIndexResponse createIndexResponse = esClient.indices().create(request, RequestOptions.DEFAULT); // 响应状态 boolean acknowledged = createIndexResponse.isAcknowledged(); System.out.println("索引操作 :" + acknowledged); esClient.close(); } }
操作结果:
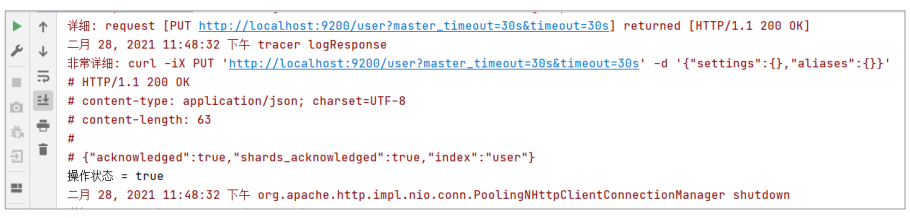
2 查看索引
// 查询索引 - 请求对象 GetIndexRequest request = new GetIndexRequest("user"); // 发送请求,获取响应 GetIndexResponse response = client.indices().get(request, RequestOptions.DEFAULT); System.out.println("aliases:"+response.getAliases()); System.out.println("mappings:"+response.getMappings()); System.out.println("settings:"+response.getSettings());
操作结果:
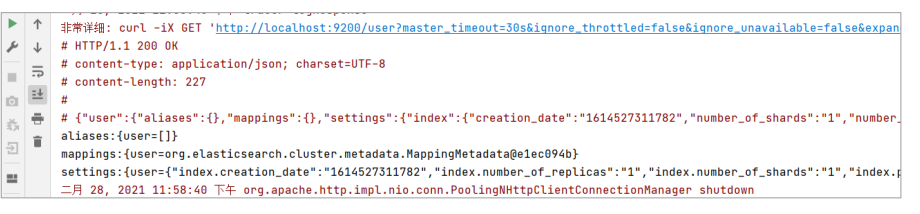
package com.atguigu.es.test; import org.apache.http.HttpHost; import org.elasticsearch.action.admin.indices.delete.DeleteIndexRequest; import org.elasticsearch.action.support.master.AcknowledgedResponse; import org.elasticsearch.client.RequestOptions; import org.elasticsearch.client.RestClient; import org.elasticsearch.client.RestHighLevelClient; import org.elasticsearch.client.indices.GetIndexRequest; import org.elasticsearch.client.indices.GetIndexResponse; public class ESTest_Index_Delete { public static void main(String[] args) throws Exception { RestHighLevelClient esClient = new RestHighLevelClient( RestClient.builder(new HttpHost("localhost", 9200, "http")) ); // 查询索引 DeleteIndexRequest request = new DeleteIndexRequest("user"); AcknowledgedResponse response = esClient.indices().delete(request, RequestOptions.DEFAULT); // 响应状态 System.out.println(response.isAcknowledged()); esClient.close(); } }
3 删除索引
package com.atguigu.es.test; import org.apache.http.HttpHost; import org.elasticsearch.action.admin.indices.delete.DeleteIndexRequest; import org.elasticsearch.action.support.master.AcknowledgedResponse; import org.elasticsearch.client.RequestOptions; import org.elasticsearch.client.RestClient; import org.elasticsearch.client.RestHighLevelClient; import org.elasticsearch.client.indices.GetIndexRequest; import org.elasticsearch.client.indices.GetIndexResponse; public class ESTest_Index_Delete { public static void main(String[] args) throws Exception { RestHighLevelClient esClient = new RestHighLevelClient( RestClient.builder(new HttpHost("localhost", 9200, "http")) ); // 查询索引 DeleteIndexRequest request = new DeleteIndexRequest("user"); AcknowledgedResponse response = esClient.indices().delete(request, RequestOptions.DEFAULT); // 响应状态 System.out.println(response.isAcknowledged()); esClient.close(); } }