1、创建django项目
$ django-admin startproject DailyFresh
2、进入项目目录
$ cd DailyFresh
3、启动项目
$ python manage.py runserver Watching for file changes with StatReloader [28/Mar/2020 17:34:35] "GET / HTTP/1.1" 200 16351 [28/Mar/2020 17:34:35] "GET /static/admin/css/fonts.css HTTP/1.1" 200 423 [28/Mar/2020 17:34:35] "GET /static/admin/fonts/Roboto-Bold-webfont.woff HTTP/1.1" 200 86184 [28/Mar/2020 17:34:35] "GET /static/admin/fonts/Roboto-Regular-webfont.woff HTTP/1.1" 200 85876 [28/Mar/2020 17:34:35] "GET /static/admin/fonts/Roboto-Light-webfont.woff HTTP/1.1" 200 85692 Not Found: /favicon.ico [28/Mar/2020 17:34:39] "GET /favicon.ico HTTP/1.1" 404 1976 Performing system checks...
4、创建cart、goods、order、user4个应用
$ python manage.py startapp cart Administrator@PC-20171101OWRR MINGW64 /d/wordspace/python_project/DailyFresh $ python manage.py startapp goods Administrator@PC-20171101OWRR MINGW64 /d/wordspace/python_project/DailyFresh $ python manage.py startapp order Administrator@PC-20171101OWRR MINGW64 /d/wordspace/python_project/DailyFresh $ python manage.py startapp user
5、在settings中设置INSTALLED_APPS,和DATABASES(前提是现在数据库中建立daily_fresh数据库)
INSTALLED_APPS = [ 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'goods', 'order', 'cart', 'user', ]
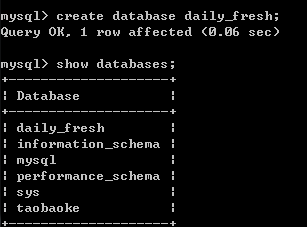
然后在settings中设置DATABASES;
DATABASES = { 'default': { 'ENGINE': 'django.db.backends.mysql', 'NAME':'daily_fresh', 'USER':'root',//数据库用户名 'PASSWORD':'123456',//数据库密码 'HOST':'localhost', 'PORT':'3306', } }
6、用python manage.py migrate创建数据库表
$ python manage.py migrate Operations to perform: Apply all migrations: admin, auth, contenttypes, sessions Running migrations: Applying contenttypes.0001_initial... OK Applying auth.0001_initial... OK Applying admin.0001_initial... OK Applying admin.0002_logentry_remove_auto_add... OK Applying admin.0003_logentry_add_action_flag_choices... OK Applying contenttypes.0002_remove_content_type_name... OK Applying auth.0002_alter_permission_name_max_length... OK Applying auth.0003_alter_user_email_max_length... OK Applying auth.0004_alter_user_username_opts... OK Applying auth.0005_alter_user_last_login_null... OK Applying auth.0006_require_contenttypes_0002... OK Applying auth.0007_alter_validators_add_error_messages... OK Applying auth.0008_alter_user_username_max_length... OK Applying auth.0009_alter_user_last_name_max_length... OK Applying auth.0010_alter_group_name_max_length... OK Applying auth.0011_update_proxy_permissions... OK Applying sessions.0001_initial... OK
至此项目架构已经搭好。
7、之后自己开始在每一个模块内建立数据模型。以下是在user应用的model.py中建立的模型。该模型对系统自带的user模型进行了扩展,继承了AbstractUser,
# Create your models here. class User(AbstractUser,BaseModel): """用户模型""" def generate_active_token(self): """生成用户签名字符串""" serializer = Serializer(settings.SECRET_KEY, 3600) info = {'confirm':self.id} token = serializer.dump(info) return token.decode() class Meta: db_table = 'user' verbose_name = '用户' verbose_name_plural = verbose_name
8、下一步想让数据模型写入数据库内,但在执行 python manage.py makemigrations时提示错误
$ python manage.py makemigrations SystemCheckError: System check identified some issues: ERRORS: auth.User.groups: (fields.E304) Reverse accessor for 'User.groups' clashes with reverse accessor for 'User.groups'. HINT: Add or change a related_name argument to the definition for 'User.groups' or 'User.groups'. auth.User.user_permissions: (fields.E304) Reverse accessor for 'User.user_permissions' clashes with reverse accessor for 'User.user_permissions'. HINT: Add or change a related_name argument to the definition for 'User.user_permissions' or 'User.user_permissions'. user.User.groups: (fields.E304) Reverse accessor for 'User.groups' clashes with reverse accessor for 'User.groups'. HINT: Add or change a related_name argument to the definition for 'User.groups' or 'User.groups'. user.User.user_permissions: (fields.E304) Reverse accessor for 'User.user_permissions' clashes with reverse accessor for 'User.user_permissions'. HINT: Add or change a related_name argument to the definition for 'User.user_permissions' or 'User.user_permissions'.
解决方案:
需要在setting中重载AUTH_USER_MODEL
# 格式为 "<django_app名>.<model名>" AUTH_USER_MODEL = "user.User"
如下调整后,执行正常
$ python manage.py makemigrations Migrations for 'user': usermigrations 001_initial.py - Create model User - Create model Address
9、但在执行python manage.py migrate写入数据库时,又提示错误了
$ python manage.py migrate Traceback (most recent call last): File "manage.py", line 21, in <module> main() File "manage.py", line 17, in main execute_from_command_line(sys.argv) File "C:Python36libsite-packagesdjangocoremanagement\__init__.py", line 401, in execute_from_command_line utility.execute() File "C:Python36libsite-packagesdjangocoremanagement\__init__.py", line 395, in execute self.fetch_command(subcommand).run_from_argv(self.argv) File "C:Python36libsite-packagesdjangocoremanagementase.py", line 328, in run_from_argv self.execute(*args, **cmd_options) File "C:Python36libsite-packagesdjangocoremanagementase.py", line 369, in execute output = self.handle(*args, **options) File "C:Python36libsite-packagesdjangocoremanagementase.py", line 83, in wrapped res = handle_func(*args, **kwargs) File "C:Python36libsite-packagesdjangocoremanagementcommandsmigrate.py", line 89, in handle executor.loader.check_consistent_history(connection) File "C:Python36libsite-packagesdjangodbmigrationsloader.py", line 299, in check_consistent_history connection.alias, django.db.migrations.exceptions.InconsistentMigrationHistory: Migration admin.0001_initial is applied before its dependency user.0001_initial on database 'default'.
经查是因为在第6步已经执行过python manage.py migrate,现在再执行写不进去了。我的解决办法就是将之前生成的daily_fresh数据库全部删除,然后重新建立数据库,再执行python manage.py migrate,问题就解决了。这个方法主要参考了这个链接(https://stackoverflow.com/questions/42412409/inconsistentmigrationhistory-error-with-custom-django-user-model.)
1)删除数据库
2)重新建立数据库
3)再执行python manage.py migrate 。注意此处没有先执行python manage.py makemigrations,因为执行了也提示no changes detected
$ python manage.py migrate
Operations to perform:
Apply all migrations: admin, auth, contenttypes, sessions, user
Running migrations:
Applying contenttypes.0001_initial... OK
Applying contenttypes.0002_remove_content_type_name... OK
Applying auth.0001_initial... OK
Applying auth.0002_alter_permission_name_max_length... OK
Applying auth.0003_alter_user_email_max_length... OK
Applying auth.0004_alter_user_username_opts... OK
Applying auth.0005_alter_user_last_login_null... OK
Applying auth.0006_require_contenttypes_0002... OK
Applying auth.0007_alter_validators_add_error_messages... OK
Applying auth.0008_alter_user_username_max_length... OK
Applying auth.0009_alter_user_last_name_max_length... OK
Applying auth.0010_alter_group_name_max_length... OK
Applying auth.0011_update_proxy_permissions... OK
Applying user.0001_initial... OK
Applying admin.0001_initial... OK
Applying admin.0002_logentry_remove_auto_add... OK
Applying admin.0003_logentry_add_action_flag_choices... OK
Applying sessions.0001_initial... OK
$ django-admin startproject DailyFresh
|