One day Alex was creating a contest about his friends, but accidentally deleted it. Fortunately, all the problems were saved, but now he needs to find them among other problems.
But there are too many problems, to do it manually. Alex asks you to write a program, which will determine if a problem is from this contest by its name.
It is known, that problem is from this contest if and only if its name contains one of Alex's friends' name exactly once. His friends' names are "Danil", "Olya", "Slava", "Ann" and "Nikita".
Names are case sensitive.
The only line contains string from lowercase and uppercase letters and "_" symbols of length, not more than 100 — the name of the problem.
Print "YES", if problem is from this contest, and "NO" otherwise.
Alex_and_broken_contest
NO
NikitaAndString
YES
Danil_and_Olya
NO
思路:
直接用string带的find函数查就行了
实现代码:
#include<bits/stdc++.h> using namespace std; int main() { string s; cin>>s; int flag = 0; int len = s.size(); if(s.find("Danil")!=s.npos){ if(s.find("Danil",s.find("Danil")+1)==s.npos) flag ++; else flag += 2; } if(s.find("Olya")!=s.npos){ if(s.find("Olya",s.find("Olya")+1)==s.npos) flag ++; else flag += 2; } if(s.find("Slava")!=s.npos){ if(s.find("Slava",s.find("Slava")+1)==s.npos) flag ++; else flag += 2; } if(s.find("Ann")!=s.npos){ if(s.find("Ann",s.find("Ann")+1)==s.npos) flag ++; else flag += 2; } if(s.find("Nikita")!=s.npos){ if(s.find("Nikita",s.find("Nikita")+1)==s.npos) flag ++; else flag += 2; } if(flag == 1) cout<<"YES"<<endl; else cout<<"NO"<<endl; }
One day Nikita found the string containing letters "a" and "b" only.
Nikita thinks that string is beautiful if it can be cut into 3 strings (possibly empty) without changing the order of the letters, where the 1-st and the 3-rd one contain only letters "a" and the 2-nd contains only letters "b".
Nikita wants to make the string beautiful by removing some (possibly none) of its characters, but without changing their order. What is the maximum length of the string he can get?
The first line contains a non-empty string of length not greater than 5 000 containing only lowercase English letters "a" and "b".
Print a single integer — the maximum possible size of beautiful string Nikita can get.
abba
4
bab
2
It the first sample the string is already beautiful.
In the second sample he needs to delete one of "b" to make it beautiful.
思路:
dp,
dp[0][i] 代表为当前字符串类型为a的情况,dp[1][i]代表当前字符串类型为ab的情况,dp2[[i]代表当前字符串类型为aba的情况
状态转移方程可以表示为:
dp[0][i+1] = dp[0][i] + (s[i] == 'a'); a状态只能由a+a得到的,当si = a时,dp[0][i]++即可; dp[1][i+1] = max(dp[1][i],dp[0][i]) + (s[i] == 'b'); ab状态可由a+b或ab+b得到当si = b时2取两种情况下最大的即可, dp[2][i+1] = max(max(dp[1][i],dp[0][i]),dp[2][i]) + (s[i] == 'a'); aba情况包括了前两中状态同时也可以由自身aba+a得到,所有当s==a时取三种状态中最大的
最后取三种情况下最大的即可
实现代码:
#include<bits/stdc++.h> using namespace std; int dp[4][10000]; int main() { string s; cin>>s; int len = s.size(); dp[0][0] = 0; //代表情况a dp[1][0] = 0; //代表情况ab dp[2][0] = 0; //代表情况aba for(int i = 0;i < len;i ++){ dp[0][i+1] = dp[0][i] + (s[i] == 'a'); dp[1][i+1] = max(dp[1][i],dp[0][i]) + (s[i] == 'b'); dp[2][i+1] = max(max(dp[1][i],dp[0][i]),dp[2][i]) + (s[i] == 'a'); } cout<<max(max(dp[0][len],dp[1][len]),dp[2][len])<<endl; }
Slava plays his favorite game "Peace Lightning". Now he is flying a bomber on a very specific map.
Formally, map is a checkered field of size 1 × n, the cells of which are numbered from 1 to n, in each cell there can be one or several tanks. Slava doesn't know the number of tanks and their positions, because he flies very high, but he can drop a bomb in any cell. All tanks in this cell will be damaged.
If a tank takes damage for the first time, it instantly moves to one of the neighboring cells (a tank in the cell n can only move to the cell n - 1, a tank in the cell 1 can only move to the cell 2). If a tank takes damage for the second time, it's counted as destroyed and never moves again. The tanks move only when they are damaged for the first time, they do not move by themselves.
Help Slava to destroy all tanks using as few bombs as possible.
The first line contains a single integer n (2 ≤ n ≤ 100 000) — the size of the map.
In the first line print m — the minimum number of bombs Slava needs to destroy all tanks.
In the second line print m integers k1, k2, ..., km. The number ki means that the i-th bomb should be dropped at the cell ki.
If there are multiple answers, you can print any of them.
2
3
2 1 2
3
4
2 1 3 2
思路:
之前直接扔翻译看中文结果看成是轰炸n,会移到n-1,漏了n+1,搞得wa到怀疑人生,轰炸n会移到n-1和n+1,那么只要先炸一遍偶数区域再炸一遍奇数,再炸一遍偶数那么就可以保证最少的情况全部炸毁
为什么不先炸奇数,,因为当n为奇数的时候,先炸奇数会多出一步操作
实现代码:
#include<bits/stdc++.h> using namespace std; int dp[4][10000]; int main() { int n; cin>>n; cout<<n+n/2<<endl; for(int i = 2;i <= n;i +=2) cout<<i<<" "; for(int i = 1;i <= n;i +=2) cout<<i<<" "; for(int i = 2;i <= n;i +=2) cout<<i<<" "; }
Olya loves energy drinks. She loves them so much that her room is full of empty cans from energy drinks.
Formally, her room can be represented as a field of n × m cells, each cell of which is empty or littered with cans.
Olya drank a lot of energy drink, so now she can run k meters per second. Each second she chooses one of the four directions (up, down, left or right) and runs from 1 to k meters in this direction. Of course, she can only run through empty cells.
Now Olya needs to get from cell (x1, y1) to cell (x2, y2). How many seconds will it take her if she moves optimally?
It's guaranteed that cells (x1, y1) and (x2, y2) are empty. These cells can coincide.
The first line contains three integers n, m and k (1 ≤ n, m, k ≤ 1000) — the sizes of the room and Olya's speed.
Then n lines follow containing m characters each, the i-th of them contains on j-th position "#", if the cell (i, j) is littered with cans, and "." otherwise.
The last line contains four integers x1, y1, x2, y2 (1 ≤ x1, x2 ≤ n, 1 ≤ y1, y2 ≤ m) — the coordinates of the first and the last cells.
Print a single integer — the minimum time it will take Olya to get from (x1, y1) to (x2, y2).
If it's impossible to get from (x1, y1) to (x2, y2), print -1.
3 4 4
....
###.
....
1 1 3 1
3
3 4 1
....
###.
....
1 1 3 1
8
2 2 1
.#
#.
1 1 2 2
-1
In the first sample Olya should run 3 meters to the right in the first second, 2 meters down in the second second and 3 meters to the left in the third second.
In second sample Olya should run to the right for 3 seconds, then down for 2 seconds and then to the left for 3 seconds.
Olya does not recommend drinking energy drinks and generally believes that this is bad.
思路:
一开始想简单了以为是直接找最短的路径长度,题目说的是1s朝某个方向跑k米,那么我们就直接枚举每个方向每秒跑的1-k米之间的所有情况,直接用bfs
暴力搜就行了。因为bfs是分层次访问的,那么最先到达终点的肯定就是耗时最短的。
实现代码:
#include<bits/stdc++.h> using namespace std; const int M = 1009; struct node{ int x,y,time; }; int dx[4] = {0,0,1,-1}; int dy[4] = {1,-1,0,0}; int vis[M][M][4]; char mp[M][M]; int ex,ey,sx,sy,n,m,k; int bfs(){ node t,t1; queue<node>q; t.x = sx;t.y = sy;t.time = 0; vis[sx][sy][0] = 1;vis[sx][sy][1] = 1; vis[sx][sy][2] = 1;vis[sx][sy][3] = 1; q.push(t); while(!q.empty()){ t = q.front(); q.pop(); if(t.x == ex&&t.y == ey) return t.time; for(int i = 0;i < 4;i ++){ for(int j = 1;j <= k;j ++){ int nx = t.x + j*dx[i]; int ny = t.y + j*dy[i]; if(mp[nx][ny] == '.'&&nx >= 1&&nx <= n&&ny >= 1&&ny <= m&&!vis[nx][ny][i]){ vis[nx][ny][i] = 1; t1.x = nx; t1.y = ny; t1.time = t.time+1; q.push(t1); } else break; } } } return -1; } int main() { cin>>n>>m>>k; for(int i = 1;i <= n;i ++) for(int j = 1;j <= m;j ++) cin>>mp[i][j]; cin>>sx>>sy>>ex>>ey; cout<<bfs()<<endl; }
Danil decided to earn some money, so he had found a part-time job. The interview have went well, so now he is a light switcher.
Danil works in a rooted tree (undirected connected acyclic graph) with n vertices, vertex 1 is the root of the tree. There is a room in each vertex, light can be switched on or off in each room. Danil's duties include switching light in all rooms of the subtree of the vertex. It means that if light is switched on in some room of the subtree, he should switch it off. Otherwise, he should switch it on.
Unfortunately (or fortunately), Danil is very lazy. He knows that his boss is not going to personally check the work. Instead, he will send Danil tasks using Workforces personal messages.
There are two types of tasks:
- pow v describes a task to switch lights in the subtree of vertex v.
- get v describes a task to count the number of rooms in the subtree of v, in which the light is turned on. Danil should send the answer to his boss using Workforces messages.
A subtree of vertex v is a set of vertices for which the shortest path from them to the root passes through v. In particular, the vertex v is in the subtree of v.
Danil is not going to perform his duties. He asks you to write a program, which answers the boss instead of him.
The first line contains a single integer n (1 ≤ n ≤ 200 000) — the number of vertices in the tree.
The second line contains n - 1 space-separated integers p2, p3, ..., pn (1 ≤ pi < i), where pi is the ancestor of vertex i.
The third line contains n space-separated integers t1, t2, ..., tn (0 ≤ ti ≤ 1), where ti is 1, if the light is turned on in vertex i and 0 otherwise.
The fourth line contains a single integer q (1 ≤ q ≤ 200 000) — the number of tasks.
The next q lines are get v or pow v (1 ≤ v ≤ n) — the tasks described above.
For each task get v print the number of rooms in the subtree of v, in which the light is turned on.
4
1 1 1
1 0 0 1
9
get 1
get 2
get 3
get 4
pow 1
get 1
get 2
get 3
get 4
2
0
0
1
2
1
1
0
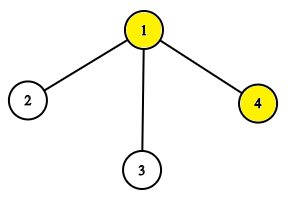
The tree after the task pow 1.
思路:
题意就是在一棵树上修改点然后查询子树,很裸的dfs序+线段树了,dfs序把树结构转化成线性结构,然后直接用线段树区间修改和查询就可以了。注意下dfs序转化的结点编号和之前的编号是不同的。
其中l[x]代表x结点转化后的编号,r[x]代表转化后x结点的子树中l[x]最大的那个,且由于dfs转化的规则,x结点的子树转换后必定为一段连续的区间,那么lx就是这段区间的左端点,rx是这段区间的右端点
所以 rx - lx + 1 等于x结点的子树所含的结点数(包括自己),这个自己看下dfs序应该就知道了。
实现代码:
#include<bits/stdc++.h> using namespace std; #define lson l,m,rt<<1 #define rson m+1,r,rt<<1|1 const int M = 2e5+20; vector<int>g[M]; int lazy[M<<2],sum[M<<2],a[M],id[M],l[M],r[M],tot; void dfs(int x){ l[x] = tot; for(int i = 0;i < g[x].size();i ++) tot++,dfs(g[x][i]); r[x] = tot; } void pushup(int rt){ sum[rt] = sum[rt<<1] + sum[rt<<1|1]; } void pushdown(int rt,int m){ if(lazy[rt]){ sum[rt<<1] = (m-(m>>1)) - sum[rt<<1]; sum[rt<<1|1] = (m>>1) - sum[rt<<1|1]; lazy[rt<<1] ^= 1; lazy[rt<<1|1] ^= 1; lazy[rt] = 0; } } void build(int l,int r,int rt){ if(l == r){ sum[rt] = a[id[l]]; return ; } int m = (l + r) >> 1; build(lson); build(rson); pushup(rt); } void update(int L,int R,int l,int r,int rt){ if(L <= l&&R >= r){ sum[rt] = r - l + 1 - sum[rt]; lazy[rt] ^= 1; return ; } pushdown(rt,r-l+1); int m = (l + r) >> 1; if(L <= m) update(L,R,lson); if(R > m) update(L,R,rson); pushup(rt); } int query(int L,int R,int l,int r,int rt){ if(L <= l&&R >= r){ return sum[rt]; } pushdown(rt,r-l+1); int ret = 0; int m = (l + r) >> 1; if(L <= m) ret += query(L,R,lson); if(R > m) ret += query(L,R,rson); return ret; } int main() { int x,n,m,k; string s; ios::sync_with_stdio(0); cin.tie(0); cout.tie(0); cin>>n; for(int i = 2;i <= n;i ++){ cin>>x; g[x].push_back(i); } for(int i = 1;i <= n;i ++) cin>>a[i]; tot = 1; dfs(1); for(int i = 1;i <= n;i ++) id[l[i]] = i; build(1,n,1); cin>>m; while(m--){ cin>>s>>k; if(s=="get") cout<<query(l[k],r[k],1,n,1)<<endl; else{ update(l[k],r[k],1,n,1); } } return 0; }