Crashing Robots
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 9859 | Accepted: 4209 |
Description
In a modernized warehouse, robots are used to fetch the goods. Careful planning is needed to ensure that the robots reach their destinations without crashing into each other. Of course, all warehouses are rectangular, and all robots occupy a circular floor space with a diameter of 1 meter. Assume there are N robots, numbered from 1 through N. You will get to know the position and orientation of each robot, and all the instructions, which are carefully (and mindlessly) followed by the robots. Instructions are processed in the order they come. No two robots move simultaneously; a robot always completes its move before the next one starts moving.
A robot crashes with a wall if it attempts to move outside the area of the warehouse, and two robots crash with each other if they ever try to occupy the same spot.
A robot crashes with a wall if it attempts to move outside the area of the warehouse, and two robots crash with each other if they ever try to occupy the same spot.
Input
The first line of input is K, the number of test cases. Each test case starts with one line consisting of two integers, 1 <= A, B <= 100, giving the size of the warehouse in meters. A is the length in the EW-direction, and B in the NS-direction.
The second line contains two integers, 1 <= N, M <= 100, denoting the numbers of robots and instructions respectively.
Then follow N lines with two integers, 1 <= Xi <= A, 1 <= Yi <= B and one letter (N, S, E or W), giving the starting position and direction of each robot, in order from 1 through N. No two robots start at the same position.
Figure 1: The starting positions of the robots in the sample warehouse
Finally there are M lines, giving the instructions in sequential order.
An instruction has the following format:
< robot #> < action> < repeat>
Where is one of
and 1 <= < repeat> <= 100 is the number of times the robot should perform this single move.
The second line contains two integers, 1 <= N, M <= 100, denoting the numbers of robots and instructions respectively.
Then follow N lines with two integers, 1 <= Xi <= A, 1 <= Yi <= B and one letter (N, S, E or W), giving the starting position and direction of each robot, in order from 1 through N. No two robots start at the same position.
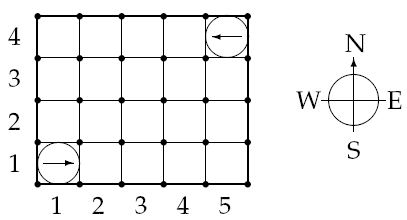
Figure 1: The starting positions of the robots in the sample warehouse
Finally there are M lines, giving the instructions in sequential order.
An instruction has the following format:
< robot #> < action> < repeat>
Where is one of
- L: turn left 90 degrees,
- R: turn right 90 degrees, or
- F: move forward one meter,
and 1 <= < repeat> <= 100 is the number of times the robot should perform this single move.
Output
Output one line for each test case:
Only the first crash is to be reported.
- Robot i crashes into the wall, if robot i crashes into a wall. (A robot crashes into a wall if Xi = 0, Xi = A + 1, Yi = 0 or Yi = B + 1.)
- Robot i crashes into robot j, if robots i and j crash, and i is the moving robot.
- OK, if no crashing occurs.
Only the first crash is to be reported.
Sample Input
4 5 4 2 2 1 1 E 5 4 W 1 F 7 2 F 7 5 4 2 4 1 1 E 5 4 W 1 F 3 2 F 1 1 L 1 1 F 3 5 4 2 2 1 1 E 5 4 W 1 L 96 1 F 2 5 4 2 3 1 1 E 5 4 W 1 F 4 1 L 1 1 F 20
Sample Output
Robot 1 crashes into the wall Robot 1 crashes into robot 2 OK Robot 1 crashes into robot 2
Source
题目大意如下:给定几个机器人在一个房间里的坐标和面对的方向(东南西北),再给定几个移动(转几次方向或沿原方向前进其次),判断这些机器人是否会撞到房间墙壁或撞到互相或平安无事。
题解:模拟即可。把方向数组设成按顺时针或逆时针的顺序可缩减代码量。转方向时,注意mod4来减少要转圈数。
15775948 | ksq2013 | 2632 | Accepted | 732K | 0MS | G++ | 1985B | 2016-07-21 18:05:33 |
代码稍长:
#include<cstdio> #include<cstring> #include<iostream> using namespace std;//横坐标x代表WE方向,纵坐标y代表NS方向; //const int mx[]={0,+1,-1,0,0};mov[1]->E,沿x轴向东/右移动+1;mov[2]->W,向西/左移动-1;mov[3]->N,沿x轴不移动;mov[4]->S,沿x轴不移动. //const int my[]={0,0,0,+1,-1};mov[1]->E,沿y轴不移动;mov[2]->W,沿y轴不移动;mov[3]->N,沿y轴向北/上移动+1;mov[4]->S,沿y轴向南/下移动-1. //顺序:东南西北;从0开始; const int mx[]={+1,0,-1,0}; const int my[]={0,-1,0,+1}; int n,m,p,q,mp[101][101]; struct robot{ int x,y,z; }rt[101]; struct req{ int k,rep; char pos; }rq[101]; void Init() { scanf("%d%d%d%d",&n,&m,&p,&q); for(int i=1;i<=p;i++){ char z; scanf("%d%d",&rt[i].x,&rt[i].y); getchar(); z=getchar(); mp[rt[i].x][rt[i].y]=i; switch(z){ case 'E':rt[i].z=0;break; case 'S':rt[i].z=1;break; case 'W':rt[i].z=2;break; case 'N':rt[i].z=3;break; } } for(int i=1;i<=q;i++){ scanf("%d",&rq[i].k); getchar(); rq[i].pos=getchar(); scanf("%d",&rq[i].rep); } } bool judge(int k) { if(rt[k].x<1||rt[k].x>n||rt[k].y<1||rt[k].y>m){ printf("Robot %d crashes into the wall ",k); return false; } if(mp[rt[k].x][rt[k].y]){ printf("Robot %d crashes into robot %d ",k,mp[rt[k].x][rt[k].y]); return false; } mp[rt[k].x][rt[k].y]=k;//修改移动后位置数据; return true; } bool solve() { for(int i=1;i<=q;i++){ int k=rq[i].k; switch(rq[i].pos){ case 'F': for(int j=1;j<=rq[i].rep;j++){ mp[rt[k].x][rt[k].y]=0;//清空原位置数据; rt[k].x+=mx[rt[k].z]; rt[k].y+=my[rt[k].z]; if(!judge(k))return false; } break; case 'L':rt[k].z=(rt[k].z-rq[i].rep%4+4)%4;break; case 'R':rt[k].z=(rt[k].z+rq[i].rep%4)%4;break; } } return true; } int main() { int T; scanf("%d",&T); while(T--){ memset(mp,0,sizeof(mp)); Init(); if(solve())puts("OK"); } return 0; }