基本思想:
Step 1:选取列表的第一个元素pivotkey,将列表分为一小一大的两个子列表a、b。其中a 列表元素都比pivotkey 小, b 列表元素都比pivotkey 大。
Step 2:对子列表 a 应用 Step1;
Step 3:对子列表 b 应用 Step1;
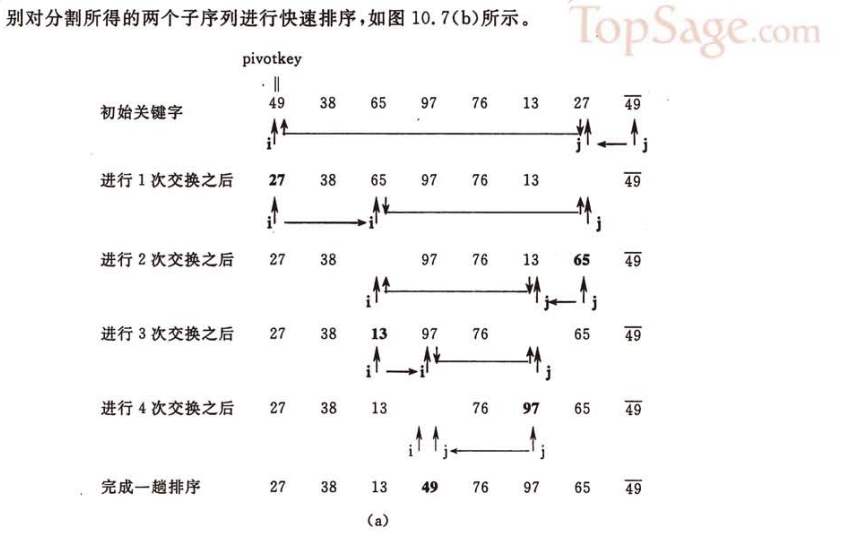
代码清单1.1。
public class QuickSort_a {
public static int[] quickSort(int[] unSortList, int leftSide, int rightSide) {
// 递归划分子列表
if (leftSide < rightSide) {
int pivotPosition = partition(unSortList, leftSide, rightSide);
quickSort(unSortList, leftSide, pivotPosition - 1);
quickSort(unSortList, pivotPosition + 1, rightSide);
}
return unSortList;
}
private static int partition(int[] list, int low, int high) {
// 以第一个元素作为pivot,划分为2个子列表
int pivot = list[low];
int leftPointer = low;
int rightPointer = high+1; // 后面while循环中 list[--rightPointer] 正好指向最后一个列表元素
while (true) {
while (list[++leftPointer] <= pivot) {
if (leftPointer == high)
break;
}
while (list[--rightPointer] >= pivot) { // 对应与前面 high+1,使得起始点是最后一个元素
if (rightPointer == low)
break;
}
if (leftPointer >= rightPointer)
break;
exchange(list, leftPointer, rightPointer);
}
exchange(list, low, rightPointer);
return rightPointer;
}
private static void exchange(int[] list, int i, int j) {
// 将列表中指定位置的元素互换,其中: list[i] > pivot,list[j]< pivot
int tmp = list[i];
list[i] = list[j];
list[j] = tmp;
}
}
代码清单1.2 (如果将上面代码中方法 partition,其余不变)
private static int partition(int[] list, int low, int high) { ...
}
替换为:
private static int partition(int[] list, int low, int high) {
int pivot = list[low];
int leftPointer = low;
int rightPointer = high; // Seeded Fault
while (true) {
while (list[++leftPointer] < pivot) {
if (leftPointer == high)
break;
}
while (list[--rightPointer] > pivot) {
if (rightPointer == low)
break;
}
if (leftPointer >= rightPointer)
break;
exchange(list, leftPointer, rightPointer);
}
exchange(list, low, rightPointer);
return rightPointer;
}
运行30个测试用例,如下:
public class TestQuickSort_a {
@Test
public void test1() {
int[] A = {};
QuickSort_a.quickSort(A, 0, 0);
int[] result = {};
assertArrayEquals(result,A);
}
@Test
public void test2() {
int[] A = { 1 };
QuickSort_a.quickSort(A, 0, 0);
int[] result = { 1 };
assertArrayEquals(result,A);
}
@Test
public void test3() {
int A[] = { 1, 2, 3, 4 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 2, 3, 4 };
assertArrayEquals(result,A);
}
@Test
public void test4() {
int A[] = { 2, 5, 1, 3, 6, 1 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
@Test
public void test5() {
int A[] = { 2, 5, 1, 1, 3, 6 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
@Test
public void test6() {
int A[] = { 2, 5, 1, 1, 6, 3 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
@Test
public void test7() {
int A[] = { 2, 5, 1, 6, 1, 3 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
@Test
public void test8() {
int A[] = { 2, 5, 1, 6, 3, 1 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
@Test
public void test9() {
int A[] = { 2, 5, 1, 1, 3, 6 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
@Test
public void test10() {
int A[] = { 2, 1, 4, 3 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 2, 3, 4 };
assertArrayEquals(result,A);
}
@Test
public void test11() {
int A[] = { 2, 3, 1, 4 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 2, 3, 4 };
assertArrayEquals(result,A);
}
@Test
public void test12() {
int A[] = { 2, 3, 4, 1 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 2, 3, 4 };
assertArrayEquals(result,A);
}
@Test
public void test13() {
int A[] = { 2, 4, 3, 1 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 2, 3, 4 };
assertArrayEquals(result,A);
}
@Test
public void test14() {
int A[] = { 2, 1, 5, 1, 6, 3 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
@Test
public void test15() {
int A[] = { 2, 1, 5, 6, 1, 3 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
@Test
public void test16() {
int A[] = { 2, 1, 3, 1, 5, 6 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
@Test
public void test17() {
int A[] = { 3, 1, 2, 4 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 2, 3, 4 };
assertArrayEquals(result,A);
}
@Test
public void test18() {
int A[] = { 3, 1, 4, 2 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 2, 3, 4 };
assertArrayEquals(result,A);
}
@Test
public void test19() {
int A[] = { 3, 4, 1, 2 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 2, 3, 4 };
assertArrayEquals(result,A);
}
@Test
public void test20() {
int A[] = { 3, 4, 2, 1 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 2, 3, 4 };
assertArrayEquals(result,A);
}
@Test
public void test21() {
int A[] = { 4, 2, 3, 1 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 2, 3, 4 };
assertArrayEquals(result,A);
}
@Test
public void test22() {
int A[] = { 4, 2, 1, 3 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 2, 3, 4 };
assertArrayEquals(result,A);
}
@Test
public void test23() {
int A[] = { 2, 1, 6, 1, 5, 3 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
@Test
public void test24() {
int A[] = { 2, 1, 6, 5, 3, 1 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
@Test
public void test25() {
int A[] = { 2, 1, 6, 3, 1, 5 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
@Test
public void test26() {
int A[] = { 2, 1, 6, 3, 5, 1 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
@Test
public void test27() {
int A[] = { 2, 1, 5, 3, 1, 6 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
@Test
public void test28() {
int A[] = { 2, 1, 5, 3, 6, 1 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
@Test
public void test29() {
int A[] = { 2, 1, 5, 6, 3, 1 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
@Test
public void test30() {
int A[] = { 2, 1, 1, 5, 3, 6 };
QuickSort_a.quickSort(A, 0, A.length - 1);
int[] result = { 1, 1, 2, 3, 5, 6 };
assertArrayEquals(result,A);
}
}
运行结果,Passed 9, Failed 21
依据上述测试结果,分别采用 Ochia、Jaccard、Tarantula、Naish2 算法对 清单1.2 进行错误定位,并标示可疑代码,如下图:

可见,错误定位指向的可疑代码,并不是真正的错误代码(浅蓝色阴影语句)