linux 系统用vim编辑python不方便,我们在windows安装 pycharm 软件(百度找安装方法)
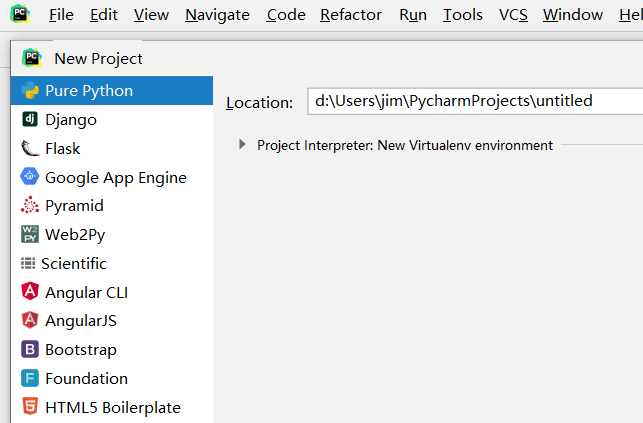
基础语法:
import getpass
import sys,os
#os.system("df -h") #调用linux系统命令
#os.system("dir") #调用windows系统命令
#os.system(''.join(sys.argv[1:])) #把用户的输入的参数当作一条命令交给os.system来执行
_name = "xj"
_passwd = "123456"
name = input("你的名字是:" )
age = int(input("年龄:"))
print(type(age))
#passwd = getpass.getpass("请输入密码:")
passwd = input("请输入用户密码:")
info = '''
name = %s
age = %d
''' % (name,age)
print(name,age)
print(info)
print(passwd)
if_else 语句:
_name = "xj"
_passwd = "123456"
if _name == name and _passwd == passwd:
print("正确 {yonghu} ".format(yonghu=name))
else:
print("错误")
while 语句:
age_of_xj = 29
count = 0
while count < 3:
input_age = int((input("猜一个年龄:")))
if age_of_xj == input_age:
print("恭喜猜对了")
break
elif age_of_xj > input_age:
print("猜小了")
else:
print("猜大了")
count +=1
else:
print(" 糟糕,猜错三次了,没有机会了")
for 语句:
age_of_xj = 29
for n in (range(0,3)): #range(0,3,2) 每隔一个输出一个数字
print(n)
input_age = int((input("猜一个年龄:")))
if age_of_xj == input_age:
print("恭喜猜对了")
break
elif age_of_xj > input_age:
print("猜小了")
else:
print("猜大了")
else:
print(" 糟糕,猜错三次了,没有机会了")
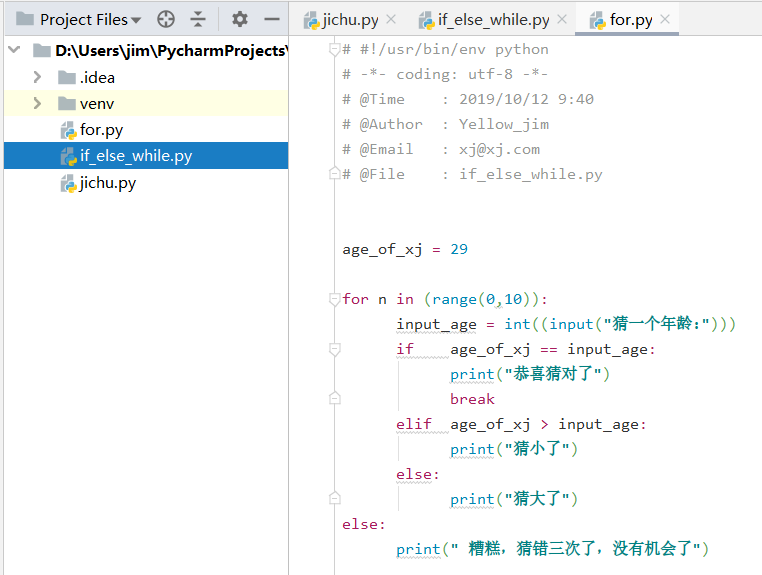
pass 语句:
Python pass 是空语句,是为了保持程序结构的完整性。
pass 不做任何事情,一般用做占位语句。
# 输出 Python 的每个字母
for letter in 'Python':
if letter == 'h':
pass
print '这是 pass 块'
print ('当前字母 :', letter)
continue break 语句:
Python continue 语句跳出本次循环,而break跳出整个循环。
continue 语句用来告诉Python跳过当前循环的剩余语句,然后继续进行下一轮循环。
for letter in 'Python':
if letter == 'h':
continue
print '当前字母 :', letter