原创文章,转载请注明来自钢铁侠Mac博客http://www.cnblogs.com/gangtiexia
折半插入排序(Binary Insertion Sort)的基本思想是将新记录插入到已经排好序的有序表中,初始有序表只有无序表的第一个数据,依次对无序表每个数据进行折半插入排序,从而得到了有序表,具体步骤为
- 先将记录存在L.r[0]中,low=有序表低位下标,high=有序表高位下标
- 若low<=high,就将L.r[0]与mid=(low+high)/2位的数据比较,如果L.r[0]>L.r[mid],则low=mid+1,如果L.r[0]<L.r[mid],则high=mid-1
- 循环第2步,则high+1就是要插入的位置
- 重复对每个新纪录执行第1、2和3步,就得到了有序表
演示实例:
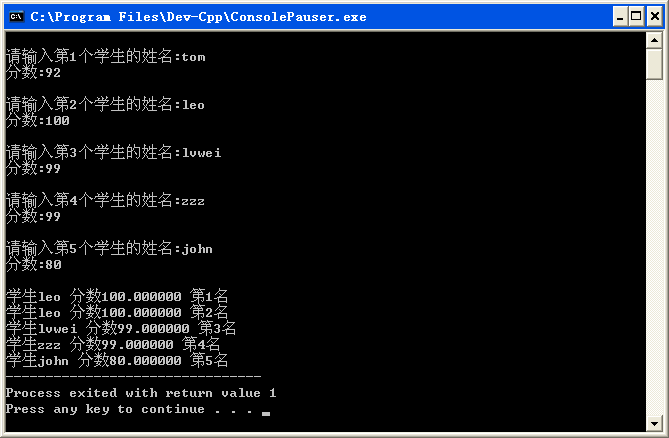
C语言实现(编译器Dev-c++5.4.0,源代码后缀.cpp)
1 #include <stdio.h> 2 #define LEN 6 3 4 typedef float keyType; 5 6 typedef struct{ 7 keyType score; 8 char name[20]; 9 }student; 10 11 typedef struct{ 12 int length=LEN; 13 student stu[LEN]; 14 }sqList; 15 16 void BinaryIS(sqList &L){ 17 int low,mid,high; 18 for(int i=2;i<L.length;i++){ 19 L.stu[0]=L.stu[i]; 20 low=1,high=i-1; 21 while(low<=high){ 22 mid=(low+high)/2; 23 if(L.stu[0].score>L.stu[mid].score) 24 high=mid-1; 25 else 26 low=mid+1; 27 } 28 L.stu[high+1]=L.stu[0]; 29 } 30 } 31 32 int main(){ 33 sqList L; 34 35 for(int i=1;i<L.length;i++){ 36 printf(" 请输入第%d个学生的姓名:",i); 37 gets(L.stu[i].name); 38 printf("分数:"); 39 scanf("%f",&(L.stu[i].score)); 40 getchar(); 41 } 42 43 BinaryIS(L); 44 45 for(int i=1;i<L.length;i++){ 46 printf(" 学生%s 分数%f 第%d名",L.stu[i].name,L.stu[i].score,i); 47 } 48 return 1; 49 }