一.多对一的映射关系
举例:根据员工编号查询员工所在部门的部门信息
第一步,需要在多的一方也就是员工实体类中持有一的一方部门实体类的引用
第二步,在dao接口中声明方法
第三步,在mapper中实现该方法
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.aaa.mybatis.dao.EmpDao"> <!---创建resultMap映射关联关系--> <!--type对应resultMap中的实体类的类型 ,需要写实体类的完全限定名--> <resultMap id="empMap" type="com.aaa.mybatis.entity.Emp"> <!--id标签用来映射实体类的主键 column对应sql语句返回值的别名 不区分大小写 property对应实体类中属性名 区分大小写 --> <id column="empno" property="empno"></id> <!--result映射普通的属性--> <result column="ename" property="ename"></result> <result column="sal" property="sal"></result> <!--配置多对一的关联映射 property对应实体类中持有的一的一方的属性名 javaType 对应持有的一的一方的属性的类型,即该属性对应类的完全限定名 --> <association property="dept" javaType="com.aaa.mybatis.entity.Dept"> <id column="deptno" property="deptno"></id> <result column="dname" property="dname"></result> <result column="loc" property="loc"></result> </association> </resultMap> <select id="findByEmpno" resultMap="empMap"> select e.empno as empno, e.ename as ename,e.sal as sal,d.deptno as deptno ,d.dname as dname ,d.loc as loc from emp e inner join dept d on e.deptno=d.deptno where e.empno=#{empno} </select> </mapper>
第四步,测试
public static void main(String[] args) { SqlSession session = SqlSessionFactoryUtil.getSession(); EmpDao empdao = session.getMapper(EmpDao.class); Emp emp = empdao.findByEmpno(7369L); System.out.println("员工编号:"+emp.getEmpno()); System.out.println("员工姓名:"+emp.getEname()); System.out.println("基本工资"+emp.getSal()); //获取员工的部门信息 Dept dept = emp.getDept(); System.out.println("部门对象:"+dept); System.out.println("部门编号:"+dept.getDeptno()); System.out.println("部门名称:"+dept.getDname()); System.out.println("部门地址:"+dept.getLoc()); System.out.println(); session.close(); }
使用在resultMap或者association中可以使用autoMapping属性,这个值设置成true,则列的别名和实体类属性名
一直的标签就可以省略不写了。
在DeptDaoMapper中一般都会有deptMap的声明:
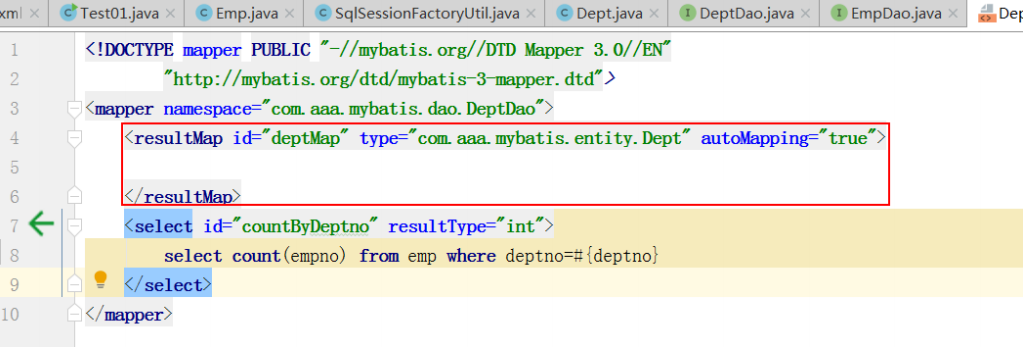
在EmpDaoMapper中配置empMap的时候,association的resultMap属性可以指向以上声明的deptMap,这样可以
达到复用map的目的。
二.一对多的映射关系
举例:根据部门编号查询某个部门下有哪些员工,打印这些员工的信息。
第一步,在一的一方持有多的一方的引用
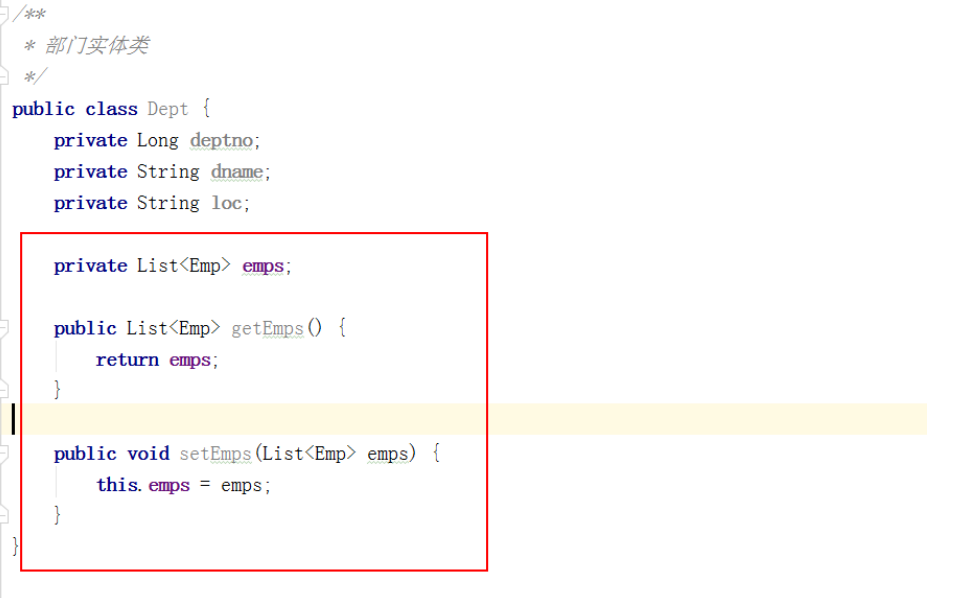
第二步,在dao接口中声明方法
第三步,在mapper中实现该方法
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.aaa.mybatis.dao.DeptDao"> <!--创建resultMap--> <resultMap id="deptMap" type="com.aaa.mybatis.entity.Dept"> <id column="deptno" property="deptno"></id> <result column="dname" property="dname"></result> <result column="loc" property="loc"></result> <!--配置一对多的映射关系 property 一的一方持有的多的一方的属性的名称 ofType 一的一方持有的多的一方的属性的集合类型中的实体类的完全限定名 --> <collection property="emps" ofType="com.aaa.mybatis.entity.Emp"> <id column="empno" property="empno"></id> <result column="ename" property="ename"></result> <result column="sal" property="sal"></result> </collection> </resultMap> <select id="findByDeptno" resultMap="deptMap"> select d.deptno,d.dname,d.loc,e.empno,e.ename,e.sal from dept d inner join emp e on d.deptno =e.deptno where d.deptno=#{deptno} </select> </mapper>
第四步,测试
/** * 配置一对多的关联 */ public class Test01 { public static void main(String[] args) { SqlSession session = SqlSessionFactoryUtil.getSession(); DeptDao deptDao = session.getMapper(DeptDao.class); Dept dept = deptDao.findByDeptno(10L); System.out.println("部门编号:"+dept.getDeptno()); System.out.println("部门名称:"+dept.getDname()); System.out.println("部门位置:"+dept.getLoc()); System.out.println("====="); List<Emp> emps = dept.getEmps(); for (Emp e : emps) { System.out.println("员工编号:"+e.getEmpno()); System.out.println("员工姓名:"+e.getEname()); System.out.println("员工基本工资:"+e.getSal()); System.out.println("---------"); } session.close(); } }