160. Intersection of Two Linked Lists
Easy
Write a program to find the node at which the intersection of two singly linked lists begins.
For example, the following two linked lists:
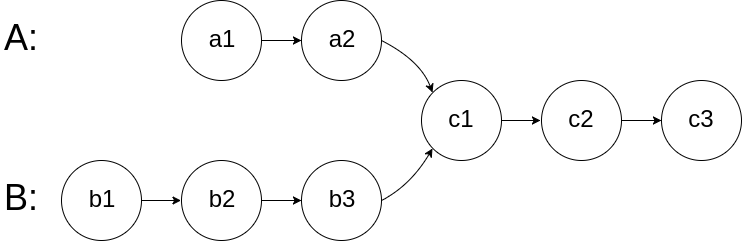
begin to intersect at node c1.
Example 1:
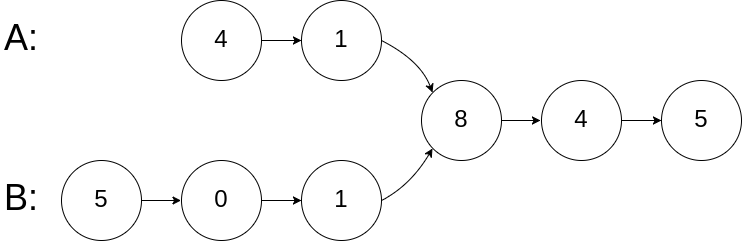
Input: intersectVal = 8, listA = [4,1,8,4,5], listB = [5,0,1,8,4,5], skipA = 2, skipB = 3 Output: Reference of the node with value = 8 Input Explanation: The intersected node's value is 8 (note that this must not be 0 if the two lists intersect). From the head of A, it reads as [4,1,8,4,5]. From the head of B, it reads as [5,0,1,8,4,5]. There are 2 nodes before the intersected node in A; There are 3 nodes before the intersected node in B.
Example 2:
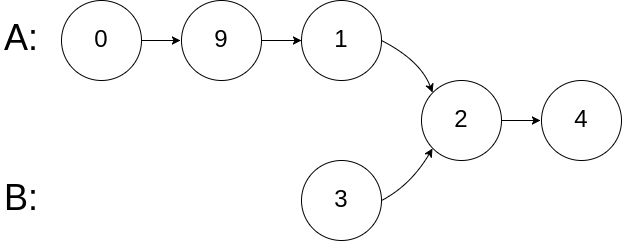
Input: intersectVal = 2, listA = [0,9,1,2,4], listB = [3,2,4], skipA = 3, skipB = 1 Output: Reference of the node with value = 2 Input Explanation: The intersected node's value is 2 (note that this must not be 0 if the two lists intersect). From the head of A, it reads as [0,9,1,2,4]. From the head of B, it reads as [3,2,4]. There are 3 nodes before the intersected node in A; There are 1 node before the intersected node in B.
Example 3:
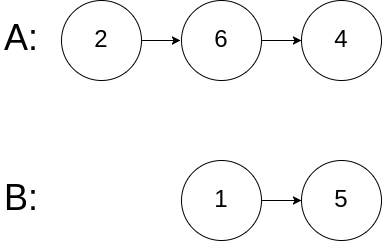
Input: intersectVal = 0, listA = [2,6,4], listB = [1,5], skipA = 3, skipB = 2 Output: null Input Explanation: From the head of A, it reads as [2,6,4]. From the head of B, it reads as [1,5]. Since the two lists do not intersect, intersectVal must be 0, while skipA and skipB can be arbitrary values. Explanation: The two lists do not intersect, so return null.
Notes:
- If the two linked lists have no intersection at all, return
null
. - The linked lists must retain their original structure after the function returns.
- You may assume there are no cycles anywhere in the entire linked structure.
- Your code should preferably run in O(n) time and use only O(1) memory.
package leetcode.easy; /** * Definition for singly-linked list. public class ListNode { int val; ListNode * next; ListNode(int x) { val = x; next = null; } } */ public class IntersectionOfTwoLinkedLists { public ListNode getIntersectionNode(ListNode headA, ListNode headB) { if (null == headA || null == headB) { return null; } else { ListNode pA = headA; ListNode pB = headB; while (pA != pB) { if (pA != null) { pA = pA.next; } else { pA = headB; } if (pB != null) { pB = pB.next; } else { pB = headA; } } return pA; } } @org.junit.Test public void test0() { ListNode a1 = new ListNode(1); ListNode a2 = new ListNode(2); ListNode b1 = new ListNode(1); ListNode b2 = new ListNode(2); ListNode b3 = new ListNode(3); ListNode c1 = new ListNode(1); ListNode c2 = new ListNode(2); ListNode c3 = new ListNode(3); a1.next = a2; b1.next = b2; b2.next = b3; a2.next = c1; b3.next = c1; c1.next = c2; c2.next = c3; c3.next = null; System.out.println(getIntersectionNode(a1, b1).val); } @org.junit.Test public void test1() { ListNode a1 = new ListNode(4); ListNode a2 = new ListNode(1); ListNode b1 = new ListNode(5); ListNode b2 = new ListNode(0); ListNode b3 = new ListNode(1); ListNode c1 = new ListNode(8); ListNode c2 = new ListNode(4); ListNode c3 = new ListNode(5); a1.next = a2; b1.next = b2; b2.next = b3; a2.next = c1; b3.next = c1; c1.next = c2; c2.next = c3; c3.next = null; System.out.println(getIntersectionNode(a1, b1).val); } @org.junit.Test public void test2() { ListNode a1 = new ListNode(0); ListNode a2 = new ListNode(9); ListNode a3 = new ListNode(1); ListNode b1 = new ListNode(3); ListNode c1 = new ListNode(2); ListNode c2 = new ListNode(4); a1.next = a2; a2.next = a3; a3.next = c1; b1.next = c1; c1.next = c2; c2.next = null; System.out.println(getIntersectionNode(a1, b1).val); } @org.junit.Test public void test3() { ListNode a1 = new ListNode(2); ListNode a2 = new ListNode(6); ListNode a3 = new ListNode(4); ListNode b1 = new ListNode(1); ListNode b2 = new ListNode(5); a1.next = a2; a2.next = a3; a3.next = null; b1.next = b2; b2.next = null; System.out.println(getIntersectionNode(a1, b1)); } }