直接选择排序
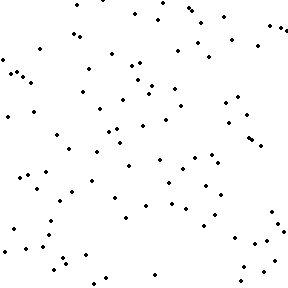
数据结构 |
数组 |
最差时间复杂度 |
O(n^2) |
最优时间复杂度 |
O(n^2) |
平均时间复杂度 |
O(n^2) |
最差空间复杂度 |
О(n) total, O(1) auxiliary |
1、算法思想
- 首先在未排序序列中找到最小(大)元素,存放到排序序列的起始位置。
- 然后,再从剩余未排序元素中继续寻找最小(大)元素,放到已排序序列的末尾。
- 以此类推,直到所有元素均排序完毕。
2、伪代码
repeat (numOfElements - 1) times
set the first unsorted element as the minimum
for each of the unsorted elements
if element < currentMinimum
set element as new minimum
swap minimum with first unsorted position
3、实现
void selection_sort(int arr[], int len) {
int i, j, min, temp;
for (i = 0; i < len - 1; i++) {
min = i;
for (j = i + 1; j < len; j++)
if (arr[min] > arr[j])
min = j;
temp = arr[min];
arr[min] = arr[i];
arr[i] = temp;
}
}