题目:
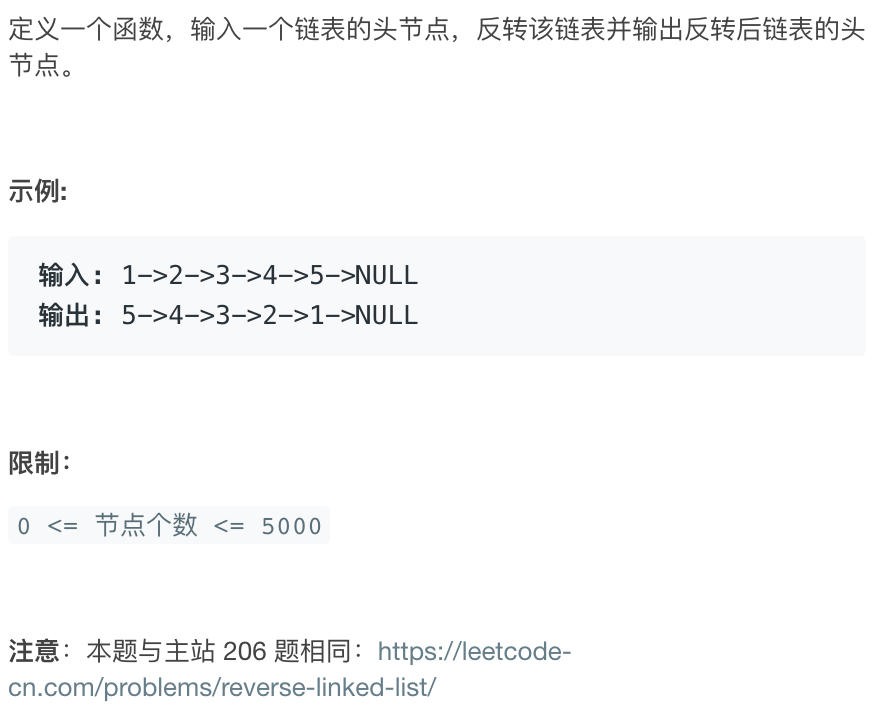
思路:
1、双指针。一个指向反转链表的头部,一个指向原链表的头部,每次从原链表头部摘下一个节点加入反转链表头部。两个指针同时移动
2、双指针。一个一直指向head也就是反转链表的尾部,head.next指向原链表头部,一个指向反转链表头部并移动
3、递归。head的反转可以转换成head.next子问题的反转
代码:
Python
# Definition for singly-linked list.
# class ListNode(object):
# def __init__(self, x):
# self.val = x
# self.next = None
class Solution(object):
def reverseList(self, head):
"""
:type head: ListNode
:rtype: ListNode
"""
# # 双指针移动
# cur = None
# pre = head
# while pre:
# tmp = pre.next
# pre.next = cur
# cur = pre
# pre = tmp
# return cur
# # head作为最后一个元素指针不移动
# if head is None:
# return None
# cur = head
# while head.next:
# tmp = head.next.next
# head.next.next = cur
# cur = head.next
# head.next = tmp
# return cur
# 递归(子问题, 子链表反转)
if head is None or head.next is None:
return head
tmp = self.reverseList(head.next)
head.next.next = head
head.next = None
return tmp