Description
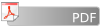
You are given a string consisting of parentheses () and []. A string of this type is said to be correct:
- (a)
- if it is the empty string
- (b)
- if A and B are correct, AB is correct,
- (c)
- if A is correct, (A ) and [A ] is correct.
Write a program that takes a sequence of strings of this type and check their correctness. Your program can assume that the maximum string length is 128.
Input
The file contains a positive integer n and a sequence of n strings of parentheses () and [], one string a line.Output
A sequence of Yes or No on the output file.Sample Input
3 ([]) (([()]))) ([()[]()])()
Sample Output
Yes No Yes
解题思路:此题应该利用栈的特点来解,输入当碰到')'或']'时进行判断,看前面是不是与之配对的'('及'[',如果是就去掉栈顶元素,若不是就要将其放进去并且退出循环,注意每进行一次判断之前都要判断栈是否为空。如果用gets(); 对数组进行输入,那么在输入数字之后要用getchar();这是因为gets();对空格也吸收。还要注意题目要求,空格也输出,所以做题要小心。
程序代码:
#include<cstdio>
#include<stack>
using namespace std;
int main()
{
int n,i;
scanf("%d",&n);
getchar();
while(n--)
{
stack<char>s;
char a[200];
gets(a);
int t=strlen(a);
if(t==0) {printf("Yes
");continue;} //用break;会使程序跳出去截止,就不会进行下一组了。
char ch;
for(i=0;i<t;i++)
{
if(a[i]==')')
{
if(s.empty())
{
s.push(a[i]);
break;
}
ch=s.top();
if(ch=='[')
break;
s.pop();
}
else if(a[i]==']')
{
if(s.empty())
{
s.push(a[i]);
break;
}
ch=s.top();
if(ch=='(')
break;
s.pop();
}
else
s.push(a[i]);
}
if(s.empty()) printf("Yes
");
else printf("No
");
}
return 0;
}