Description
A number of rectangular posters, photographs and other pictures of the same shape are pasted on a wall. Their sides are all vertical or horizontal. Each rectangle can be partially or totally covered by the others. The length of the boundary of the union of all rectangles is called the perimeter.
Write a program to calculate the perimeter. An example with 7 rectangles is shown in Figure 1.
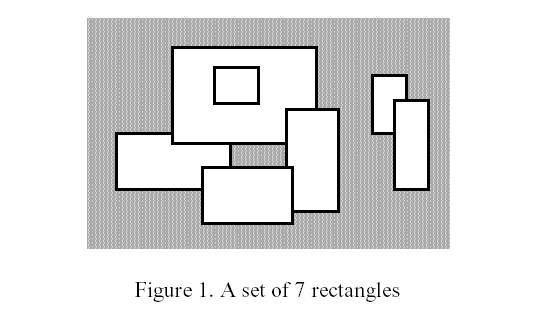
The corresponding boundary is the whole set of line segments drawn in Figure 2.
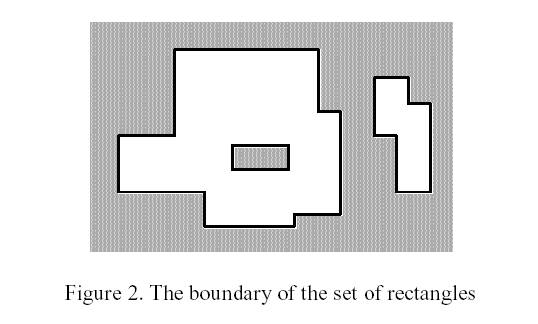
The vertices of all rectangles have integer coordinates.
Write a program to calculate the perimeter. An example with 7 rectangles is shown in Figure 1.
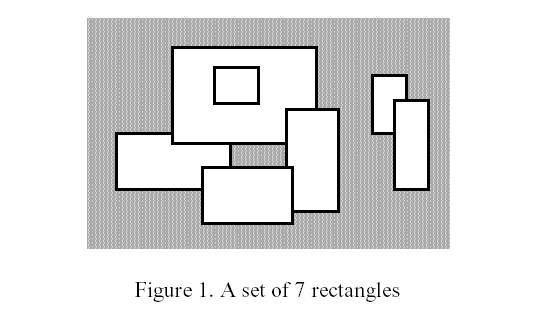
The corresponding boundary is the whole set of line segments drawn in Figure 2.
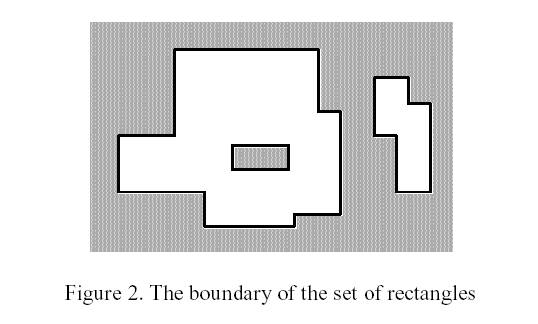
The vertices of all rectangles have integer coordinates.
Input
Your program is to read from standard input. The first line contains the number of rectangles pasted on the wall. In each of the subsequent lines, one can find the integer coordinates of the lower left vertex and the upper right vertex of each rectangle. The values of those coordinates are given as ordered pairs consisting of an x-coordinate followed by a y-coordinate.
0 <= number of rectangles < 5000
All coordinates are in the range [-10000,10000] and any existing rectangle has a positive area.
0 <= number of rectangles < 5000
All coordinates are in the range [-10000,10000] and any existing rectangle has a positive area.
Output
Your program is to write to standard output. The output must contain a single line with a non-negative integer which corresponds to the perimeter for the input rectangles.
Sample Input
7 -15 0 5 10 -5 8 20 25 15 -4 24 14 0 -6 16 4 2 15 10 22 30 10 36 20 34 0 40 16
Sample Output
228
Source
题意:有多个矩形,矩形的两边平行于坐标轴,这些矩形之间可能存在相互覆盖,求周长。
思路:记录每个矩形的两条竖边(x1,y1,y2)和(x2,y1,y2),将所有的竖边按照x从小到大排序,然后一条一条竖边开始计算周长,那么以竖边所在的垂直于x轴的直线即是扫描线。每次移动到一条新的竖边的时候,我们需要计算所在竖边扫描线上有用边长(即当前竖边的有用部分,可能当前的竖边被覆盖部分),以及加上当前扫描线与上一条扫描线之前的横边长 * 横边条数,一直计算到最后一条竖边,即是完整周长了。
代码如下:
1 #include <iostream> 2 #include <algorithm> 3 #include <cstdio> 4 #include <cstring> 5 #include <vector> 6 using namespace std; 7 const int N=5005; 8 struct Line{ 9 int x,y1,y2; 10 int flag; 11 bool operator<(const Line s) 12 { 13 if(x==s.x) return flag>s.flag; 14 return x<s.x; 15 } 16 }line[N*2]; 17 18 struct Tree 19 { 20 int l,r; 21 bool lf,rf; ///左右边界点是否被覆盖; 22 int cover_len; 23 int cover_num; 24 int num; ///矩形数目; 25 }tr[N*10]; 26 27 vector<int>v; 28 29 void build(int l,int r,int i) 30 { 31 tr[i].l=l; tr[i].r=r; 32 tr[i].cover_len=0; 33 tr[i].cover_num=0; 34 tr[i].num=0; 35 tr[i].lf=tr[i].rf=false; 36 if(l+1==r) return ; 37 int mid=(l+r)>>1; 38 build(l,mid,i<<1); 39 build(mid,r,i<<1|1); 40 } 41 void process(int i) 42 { 43 if(tr[i].cover_num>0) 44 { 45 tr[i].cover_len=v[tr[i].r]-v[tr[i].l]; 46 tr[i].lf=tr[i].rf=true; 47 tr[i].num=1; 48 return ; 49 } 50 if(tr[i].l+1==tr[i].r) 51 { 52 tr[i].cover_len=0; 53 tr[i].num=0; 54 tr[i].lf=tr[i].rf=false; 55 return ; 56 } 57 int ls=(i<<1); 58 int rs=(i<<1|1); 59 tr[i].cover_len=tr[ls].cover_len+tr[rs].cover_len; 60 tr[i].num=tr[ls].num + tr[rs].num - (tr[ls].rf & tr[rs].lf); 61 tr[i].lf=tr[ls].lf; tr[i].rf=tr[rs].rf; 62 } 63 void update(int i,Line t) 64 { 65 if(t.y1<=v[tr[i].l] && v[tr[i].r]<=t.y2) 66 { 67 tr[i].cover_num+=t.flag; 68 process(i); 69 return ; 70 } 71 int mid=(tr[i].l+tr[i].r)>>1; 72 if(t.y1<v[mid]) update(i<<1,t); 73 if(t.y2>v[mid]) update(i<<1|1,t); 74 process(i); 75 } 76 int main() 77 { 78 int n; 79 while(scanf("%d",&n)!=EOF) 80 { 81 v.clear(); 82 for(int i=0;i<n;i++) 83 { 84 int x1,y1,x2,y2; 85 scanf("%d%d%d%d",&x1,&y1,&x2,&y2); 86 line[i*2].x=x1; line[i*2].y1=y1; line[i*2].y2=y2; line[i*2].flag=1; 87 line[i*2+1].x=x2; line[i*2+1].y1=y1; line[i*2+1].y2=y2; line[i*2+1].flag=-1; 88 v.push_back(y1); 89 v.push_back(y2); 90 } 91 sort(line,line+2*n); 92 sort(v.begin(),v.end()); 93 int num=unique(v.begin(),v.end())-v.begin(); 94 95 build(0,num-1,1); 96 int ans=0,len=0; 97 for(int i=0;i<2*n;i++) 98 { 99 if(i>0) ans+=tr[1].num*2*(line[i].x-line[i-1].x); 100 update(1,line[i]); 101 ans+=abs(tr[1].cover_len-len); 102 len=tr[1].cover_len; 103 } 104 printf("%d ",ans); 105 } 106 return 0; 107 }