前面介绍了较为常用的几种Java的常用类:字符串相关类、日期相关类和比较器相关类,本篇博客将介绍其他的几种常用类,虽不如前面几种使用频繁,但还是需要了解和熟悉的。
目录:
☍ System类
System类代表系统相关的类,系统级的很多属性和控制方法都放置在该类的内部。该类位于java.lang包。
由于System类的特殊性,该类的构造器是private的,所以无法在外部实例化该类,但其内部的成员变量和成员方法都是static的,这就方便了使用者们的使用。
成员变量
☄ System类内部包含in、out和err三个成员变量,分别代表标准输入流(键盘输入),标准输出流(显示器)和标准错误输出流(显示器)。如我们最常用的控制台输出语句:System.out.println()
成员方法
☄ native long currentTimeMillis():
该方法的作用是返回当前的计算机时间,时间的表达格式为当前计算机时间和GMT时间(格林威治时间)1970年1月1号0时0分0秒所差的毫秒数,相当于new Date().getTime()。
☄ void exit(int status):
该方法的作用是退出程序。其中status的值为0代表正常退出,非零代表异常退出。使用该方法可以在图形界面编程中实现程序的退出功能等。
☄ void gc():
该方法的作用是请求系统进行垃圾回收。至于系统是否立刻回收,则取决于系统中垃圾回收算法的实现以及系统执行时的情况。
☄ String getProperty(String key):
该方法的作用是获得系统中属性名为key的属性对应的值。系统中常见的属性名以及属性的作用如下表所示:
属性名 | 说明 |
---|---|
java.version | Java运行时环境版本 |
java.home | Jdk安装目录 |
os.name | 操作系统名称 |
os.version | 操作系统版本 |
user.name | 系统用户名 |
user.home | 系统用户主目录 |
user.dir | 当前项目工作目录 |
代码:
public void testSystem(){
String javaVersion = System.getProperty("java.version");
System.out.println("Java运行时环境版本javaVersion:" + javaVersion);
String javaHome = System.getProperty("java.home");
System.out.println("Jdk安装目录javaHome:" + javaHome);
String osName = System.getProperty("os.name");
System.out.println("操作系统名称osName:" + osName);
String osVersion = System.getProperty("os.version");
System.out.println("操作系统版本osVersion:" + osVersion);
String userName = System.getProperty("user.name");
System.out.println("系统用户名userName:" + userName);
String userHome = System.getProperty("user.home");
System.out.println("系统用户主目录userHome:" + userHome);
String userDir = System.getProperty("user.dir");
System.out.println("当前项目工作目录userDir:" + userDir);
}
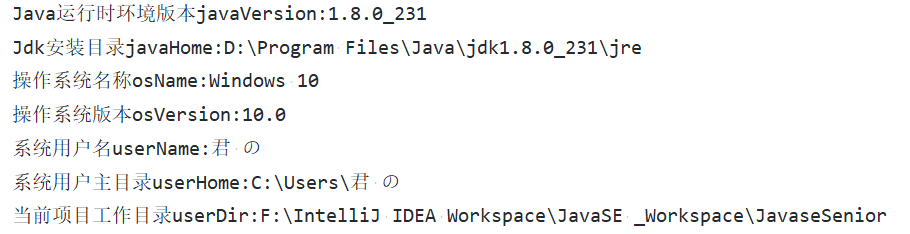
☍ Math类
java.lang.Math提供了一系列静态static方法用于数学计算。其方法的参数和返回为值类型一般为double
函数 | 说明 |
---|---|
static double abs(double a) | 绝对值 |
static double sin(double a):acos,asin,atan,cos,sin,tan | 三角函数 |
static double sqrt(double a) | 平方根 |
static double pow(double a, double b) | a 的b 次幂 |
static double log(double a) | log 自然对数 |
static double exp(double a) | e 为底a为指数 |
static double max(double a, double b) | 求两个数的最大值 |
static double min(double a, double b) | 求两个数的最小值 |
static double random() | 0.0 到1.0 的随机数 |
static long round(double a) | 四舍五入double➝long |
static double ceil(double a) | 向上取整 |
static double floor(double a) | 向下取整 |
toDegrees(double angrad) | 弧度—> 角度 |
toRadians(double angdeg) | 角度—> 弧度 |
代码:
public void testMath(){
//abs 绝对值
System.out.println("-4.2的绝对值:" + Math.abs(-4.2)); //输出:4.2
//acos,asin,atan,cos,sin,tan 三角函数
System.out.println("sin30的绝对值:" + Math.sin((1.0/6)*Math.PI)); //输出:0.49999999999999994
System.out.println("arcsin(1/2)的绝对值:" + Math.asin(0.5)); //输出:0.5235987755982989
//sqrt 平方根
System.out.println("144的平方根:"+Math.sqrt(144)); //输出:12.0
//pow(double a,doble b) a 的b 次幂
System.out.println("2的3次幂:"+Math.pow(2,3)); //输出:8.0
//log 自然对数
System.out.println("log2.718:"+Math.log(2.718281828459045)); //输出:1.0
//exp e 为底a为指数
System.out.println("e^1:"+Math.exp(1)); //输出:2.718281828459045
//max(double a,double b) 求两个数的最大值
System.out.println("14和3.2的最大值:"+Math.max(14,3.2)); //输出:14.0
//min(double a,double b) 求两个数的最小值
System.out.println("14和3.2的最小值:"+Math.min(14,3.2)); //输出:3.2
//random() 返回0.0 到1.0 的随机数
System.out.println("0-1的随机数:"+Math.random()); //输出:0.6852038863540545
//long round(double a) double 型数据a 转换为long型(四舍五入)
System.out.println("4.56四舍五入:" + Math.round(4.76)); //输出:5
//ceil(double a) 向上取整 double
System.out.println("3.12向上取整:" + Math.ceil(3.12)); //输出:4.0
//floor(double a) 向下取整 double
System.out.println("4.82向下取整:"+ Math.floor(4.82)); //输出:4.0
//toDegrees(double angrad) 弧度—> 角度
//toRadians(double angdeg) 角度—> 弧度
}
☍ BigInteger和BigDecimal
Integer类作为int的包装类,能存储的最大整型值为2 31 -1,Long类也是有限的,最大为2 63 -1。如果要表示再大的整数,不管是基本数据类型还是他们的包装类都无能为力,更无法进行数学计算。
▾ BigInteger
java.math包的BigInteger 可以表示不可变的任意精度的整数。BigInteger 提供所有 Java 的基本整数操作符的对应物,并提供 java.lang.Math 的所有相关方法(见上Math类)。
BigInteger还提供以下运算:模算术、GCD 计算、质数测试、素数生成、位操作以及一些其他操作
构造器
☄ BigInteger(String val):根据字符串构建BigInteger对象
常用方法
♩ public BigInteger abs():绝对值,返回绝对值的BigInteger
♪ BigInteger add(BigInteger val) :加法,返回值为 (this + val)
♪ BigInteger subtract(BigInteger val) :减法,返回值为 (this - val)
♬ BigInteger multiply(BigInteger val) :乘法,返回值为 (this * val)
♫ BigInteger divide(BigInteger val) :除法,返回值为 (this / val) 的 BigInteger。整数相除只保留整数部分。
♩ BigInteger remainder(BigInteger val):取余,返回值为 (this % val)
♫ BigInteger[] divideAndRemainder(BigInteger val):返回包含 (this / val) 后跟(this % val) 的两个 BigInteger 的数组。
♩ BigInteger pow(int exponent) :指数,幂值,返回其值为 (this ^exponent )
public void testBigInteger() throws ParseException {
//BigInteger构造器
BigInteger bigInteger = new BigInteger("-23523535335");
BigInteger bigInteger1 = new BigInteger("1234623623623");
BigInteger bigInteger2 = new BigInteger("4235");
//abs()绝对值
System.out.println("绝对值:" + bigInteger.abs());
//绝对值:23523535335
//add()加法
BigInteger add = bigInteger1.add(bigInteger2);
System.out.println("bigInteger1+bigInteger=" + add);
//bigInteger1+bigInteger=1234623627858
//add()减法
BigInteger sub = bigInteger1.subtract(bigInteger2);
System.out.println("bigInteger1-bigInteger2=" + sub);
//bigInteger1-bigInteger2=1234623619388
//multiply()乘法
BigInteger multiply = bigInteger1.multiply(bigInteger2);
System.out.println("bigInteger1*bigInteger2=" + multiply);
//bigInteger1*bigInteger2=5228631046043405
//divide()除法
BigInteger divide = bigInteger1.divide(bigInteger2);
System.out.println("bigInteger1/bigInteger2=" + divide);
//bigInteger1/bigInteger2=291528600
//remainder()取余
BigInteger remainder = bigInteger1.remainder(bigInteger2);
System.out.println("bigInteger1%bigInteger2=" + remainder);
//bigInteger1%bigInteger2=2623
//(this / val) 后跟(this % val) 的两个 BigInteger 的数组。
BigInteger[] divideAndRemainder = bigInteger1.divideAndRemainder(bigInteger2);
System.out.println(divideAndRemainder[0] + "--" + divideAndRemainder[1]);
//291528600--2623
//pow()幂指函数
BigInteger pow = bigInteger2.pow(2);
System.out.println("bigInteger2 ^ 2=" + pow);
//bigInteger2 ^ 2=17935225
}
▾ BigDecimal
一般的Float类和Double类可完成大多数小数的表示和计算,但在某些商业、工业或科学计算中要求数字精度比较高,此时可选择使用java.math.BigDecimal类。
构造器
☄ public BigDecimal(double val)
☄ public BigDecimal(String val)
常用方法
♩ public BigDecimal add(BigDecimal augend):加法,返回值为 (this + augend)
♫ public BigDecimal subtract(BigDecimal subtrahend):减法,返回值为 (this - subtrahend)
♪ public BigDecimal multiply(BigDecimal multiplicand):乘法,返回值为 (this * multiplicand)
♬ public BigDecimal divide(BigDecimal divisor, int scale, int roundingMode):除法,返回值为 (this / divisor) 的 BigInteger。scale为保留的小数位数,roundingMode为保留小数的模式(四舍五入/向上取整/向下取整)
public void testBigDecimal() {
//BigDecimal构造器
BigDecimal bd1 = new BigDecimal("3.1415926"); //3.1415926
BigDecimal bd2 = new BigDecimal(2020.0123456789);
//2020.012345678899919221294112503528594970703125,传入double小数结果不会具体到该小数
BigDecimal bd3 = new BigDecimal(2020); //2020
System.out.println(bd1 + "
" + bd2 + "
" + bd3);
//add()加法
BigDecimal add = bd2.add(bd1);
System.out.println(add); //2023.153938278899919221294112503528594970703125
//subtract()减法
BigDecimal sub = bd2.subtract(bd3);
System.out.println(sub); //0.012345678899919221294112503528594970703125
//multiply()乘法
BigDecimal mul = bd3.multiply(bd1);
System.out.println(mul); //6346.0170520
//divide()除法
// BigDecimal div = bd2.divide(bd3); //报错,需要指定保留模式
BigDecimal div = bd2.divide(bd3,6,BigDecimal.ROUND_CEILING);
System.out.println(div); //1.000007
}
▾ 保留小数位数
☄ 使用String类的format()方法将数字格式化为指定格式达到保留小数的目的,默认四舍五入,%.nf
,n为要保留的小数位数,返回字符串
☄ 转换为BigDecimal对象然后使用setScale()方法设置保留位数,可指定保留模式(四舍五入、向上保留,向下保留等),返回BigDecimal
☄ 使用DecimalFormat类对象df,调用format(BigDecimal b)转换为指定位数,返回字符串
public void testScale() throws ParseException {
//调用String.format()格式化数字达到保留指定小数的目的,结果为String类型,默认四舍五入
double dNum = 6.64968;
System.out.println("String.format('%.2f',6.64968)="+String.format("%.2f",dNum)); //6.65
BigDecimal bigDecimal = new BigDecimal(51561561.6554144);
//向下取整小数点后指定位:bigDecimal.setScale(精确位,BigDecimal.ROUND_CEILING);
//四舍五入:BigDecimal.ROUND_HALF_UP BigDecimal.ROUND_HALF_DOWN
//BigDecimal.ROUND_HALF_EVEN
//向上保留:ROUND_CEILING
//向下保留:ROUND_FLOOR
System.out.println("-----------BigDecimal精确小数点----------");
BigDecimal bigDecimal1 = bigDecimal.setScale(2,BigDecimal.ROUND_CEILING);
System.out.println("51561561.6554144保留两位小数:" + bigDecimal);
//51561561.655414402484893798828125
System.out.println(bigDecimal1); //51561561.66
DecimalFormat df = new DecimalFormat("0.0000");
//格式化BigDecimal对象为字符串
String d1 = df.format(bigDecimal); //默认四舍五入
System.out.println("格式化51561561.6554144:" + d1); //51561561.6554
//字符串解析为Number对象,再调用xxxValue()方法转为对应基本数据类型
Number num1 = df.parse("614646.5565");
System.out.println("解析614646.5565:"+num1.doubleValue());
//类似SimpleDateFormat的用法
}
本博客与CSDN博客༺ཌ༈君☠纤༈ད༻同步发布