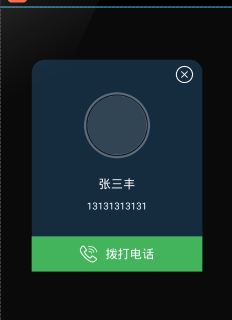
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<solid android:color="@color/home_00" />
<corners
android:bottomLeftRadius="20dp"
android:bottomRightRadius="20dp"
android:topLeftRadius="20dp"
android:topRightRadius="20dp" />
<stroke
android:width="2dp"
android:color="@color/home_00" />
</shape>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true">
<RelativeLayout
android:layout_width="280dp"
android:layout_height="wrap_content"
android:background="@drawable/pop_bg"
android:orientation="vertical"
android:id="@+id/pop_student"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true">
<ImageView
android:id="@+id/img_pop_close"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/student_close"
android:layout_alignParentRight="true"
android:layout_marginTop="10dp"
android:layout_marginRight="15dp"
android:layout_alignParentTop="true" />
<LinearLayout xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/layout_head"
android:background="@drawable/student_head_bg"
android:layout_below="@id/img_pop_close"
android:layout_marginTop="15dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:orientation="horizontal"
android:layout_centerHorizontal="true">
<com.jtx.iintroduce.widget.CircleImageView
android:id="@+id/img_pop_head"
android:layout_width="100dp"
android:layout_height="100dp"
android:src="@drawable/home_head"
app:border_width="1dp" />
</LinearLayout>
<TextView
android:id="@+id/txt_pop_name"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/layout_head"
android:textColor="@color/white"
android:layout_centerHorizontal="true"
android:layout_marginTop="30dp"
android:textSize="20sp"
android:text="张三丰" />
<TextView
android:id="@+id/txt_pop_phone"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/txt_pop_name"
android:textColor="@color/white"
android:layout_centerHorizontal="true"
android:layout_marginTop="15dp"
android:textSize="16sp"
android:text="13131313131" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/txt_pop_phone"
android:gravity="center"
android:background="@drawable/pop_bg_button"
android:paddingTop="5dp"
android:paddingBottom="5dp"
android:layout_marginTop="40dp">
<Button
android:id="@+id/btn_pop_call"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@color/transparent"
android:textColor="@color/white"
android:textSize="20sp"
android:text="拨打电话"
android:drawablePadding="13dp"
android:drawableLeft="@drawable/student_phone2" />
</LinearLayout>
</RelativeLayout>
</RelativeLayout>
1 public class StudentPopWindow extends PopupWindow implements View.OnClickListener {
2
3 private View popView = null;
4 private BaseActivity act = null;
5 private CircleImageView img_pop_head = null;
6 private TextView txt_pop_name = null;
7 private TextView txt_pop_phone = null;
8 private String strPhone = null;
9
11 public StudentPopWindow(BaseActivity act, String head, String name, String phone) {
12 super(act);
13 LayoutInflater inflater = (LayoutInflater) act
14 .getSystemService(Context.LAYOUT_INFLATER_SERVICE);
15 popView = inflater.inflate(R.layout.pop_student, null);
16
17 this.act = act;
18 strPhone = phone;
19 popView.findViewById(R.id.btn_pop_call).setOnClickListener(this);
20 popView.findViewById(R.id.img_pop_close).setOnClickListener(this);
21
22 img_pop_head = (CircleImageView) popView.findViewById(R.id.img_pop_head);
23 txt_pop_name = (TextView) popView.findViewById(R.id.txt_pop_name);
24 txt_pop_phone = (TextView) popView.findViewById(R.id.txt_pop_phone);
25 act.mToolBitmap.display(img_pop_head, head);
26 txt_pop_name.setText(name);
27 txt_pop_phone.setText(phone);
28
29
30 //设置SelectPicPopupWindow的View
31 this.setContentView(popView);
32 //设置SelectPicPopupWindow弹出窗体的宽
33 this.setWidth(LayoutParams.MATCH_PARENT);
34 //设置SelectPicPopupWindow弹出窗体的高
35 this.setHeight(LayoutParams.MATCH_PARENT);
36 //设置SelectPicPopupWindow弹出窗体可点击
37 this.setFocusable(true);
38
39 //设置SelectPicPopupWindow弹出窗体动画效果
40 //this.setAnimationStyle(R.style.AnimBottom);
41 //实例化一个ColorDrawable颜色为半透明
42 ColorDrawable dw = new ColorDrawable(act.getResources().getColor(R.color.b_translucent));
43 //设置SelectPicPopupWindow弹出窗体的背景
44 this.setBackgroundDrawable(dw);
45
46 //mMenuView添加OnTouchListener监听判断获取触屏位置如果在选择框外面则销毁弹出框
47 /*popView.setOnTouchListener(new OnTouchListener() {
49 public boolean onTouch(View v, MotionEvent event) {
51 int height = popView.findViewById(R.id.pop_student).getTop();
52 int y=(int) event.getY();
53 if (event.getAction() == MotionEvent.ACTION_UP) {
54 if (y < height) {
55 dismissPop();
56 }
57 }
58 return true;
59 }
60 });*/
61
62 }
63
65 @Override
66 public void onClick(View v) {
67 switch (v.getId()) {
68 case R.id.img_pop_close:
69 dismissPop();
70 break;
71 case R.id.btn_pop_call:
72 Intent intent = new Intent(Intent.ACTION_CALL, Uri.parse("tel://" + strPhone));
73 act.startActivity(intent);
74 break;
75 }
76 }
77
79 private void dismissPop() {
80 popView = null;
81 act = null;
82 img_pop_head = null;
83 txt_pop_name = null;
84 txt_pop_phone = null;
85 strPhone = null;
86 dismiss();
87 }
88 }
String strHead = listData.get(position).photo;
String strName = listData.get(position).name;
String strPhone = listData.get(position).phone;
popWindow = new StudentPopWindow(mActivity, strHead, strName, strPhone);
popWindow.setHeight(ViewGroup.LayoutParams.MATCH_PARENT);
popWindow.setWidth(ViewGroup.LayoutParams.MATCH_PARENT);
//显示窗口
//设置layout在PopupWindow中显示的位置
popWindow.showAtLocation(view, Gravity.CENTER, 0, 0);