一、父组件怎么覆盖子组件的样式呢
1./deep/(不建议这么做,以后angular会取消,因为这样写不利于组件的独立性)
在父组件的scss里面写:
:host{ 子组件名 /deep/ label{ color:red } }
这样就可以覆盖掉子组件label的color了
2.host和host-context
在子组件的scss里面写:
:host(.自身加的class){ label{ color:red; } }
或者
:host-context(父组件名){ label{ color:red; } }
网上查到的定义:
:host(selector) { ... }
forselector
to match attributes, classes, ... on the host element-
:host-context(selector) { ... }
forselector
to match elements, classes, ...on parent
二、Unit Test for Service
有两个方法,但是其实都是大同小异,都需要inject来生成实例,和mockBackend来模拟http返回data
方法一:注入要测试的service和MockBackend,并得到其实例
import { MockBackend } from '@angular/http/testing'; import { TestBed, inject } from '@angular/core/testing'; import { TestService } from './test.service'; import { Response, ResponseOptions } from '@angular/http'; describe("test.service", () => { let testService:any; let backend:any; beforeEach(() => { TestBed.configureTestingModule({ providers: [TestService, MockBackend] }); }) //inject注入要测试的service和mockbackend(模拟http返回数据),后面注入function拿到对应实例 beforeEach(inject([TestService, MockBackend], (testService, mockBackend) => { this.testService = testService; this.backend = mockBackend; })) it('test a service', () => { let MOCKDATA = { //一些模拟数据... } this.backend.connections.subscribe((connection) => { connection.mockRespond(new Response(new ResponseOptions({ body: JSON.stringify(MOCKDATA) }))); }); this.testService.getData('一些api需要的数据').subscribe((res) => { expect(res.length).toEqual(10); }); }) })
方法二:自己重写一个http,mockData传到自己重写的http上,前提是service调用了自己重写的http方法
describe("test.service", () => { let testService:any; beforeEach(() => { let mockData = new ResponseOptions({ body: JSON.stringify({ //MockData }) }); let cshttpServiceStub: any = { get: function () { return Observable.create(observer => { observer.next((new Response(mockData))); observer.complete(); }) } } TestBed.configureTestingModule({ providers: [ TestService, { provide: CshttpService, useValue: cshttpServiceStub } ] }); }) //还是需要inject,才能在不调用component请款下创建service实例 beforeEach(inject([TestService], (testService) => { this.testService = testService; })) it('test a service', () => { this.testService.getData('一些api需要的数据').subscribe((res) => { expect(res.length).toEqual(10); }); }) })
三、面对没有值的html属性,怎么办?
例如input的disabled,video和audio的autoplay、loop,ol的reversed.....
这些属性都是只要存在于标签上就会有所影响:
例如:
<video autoplay src="1.mp4"></video> <video autoplay="false" src="1.mp4"></video>
无论给autoplay什么值都好,甚至给它false都好,这个video还是会自动播放,这个时候Angular提供了比较方便的方法去实现动态判断是否留下这样属性的方法
大致有2个方法:
方法1:中括号输入法
<video [autoplay]="false" src="1.mp4"></video>
把autoplay用中括号包住,如果是false,Angular就会自动把它去除掉,编译出来就变成
<video src="1.mp4"></video>
方法2:中括号attr输入法
<video [attr.autoplay]="true" src="1.mp4"></video> <video [attr.autoplay]="null" src="1.mp4"></video>
attr.属性和中括号输入法差不多,不同的是false的时候不能输入false,因为attr.属性会把输入的值原封不动地输入去,编译成下面这样:
<video autoplay="false" src="1.mp4"></video>
而输入null则可以实现和括号输入法输入false时一样的效果。
四、常用的Angular内置的指令
指令 |
例子 |
ngIf | *ngIf="true" |
ngFor | *ngFor="let btn of btns" |
ngClass | [ngClass]="{'info':true}" |
ngModel | [(ngModel)] = "person.name" |
ngModelChange |
(ngModelChange)="onChange($event)"
|
ngSubmit | (ngSubmit) = "onSubmit($event)" |
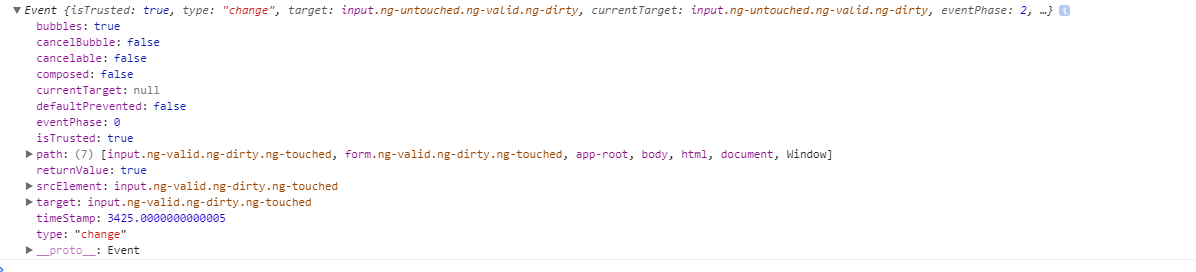