数据类型
数字类型
int
1、用途
2、定义方式
age = 18 # age = int(18)
print(type(age))
>>>:
<class 'int'>
int数据类型转换:可以把纯数字组成的字符串转成整型
res = int(' 18 ') # 左右可以有空格,中间不能有空格
print(res,type(res))
>>>:
18 <class 'int'>
3、常用操作+内置方法
4、该类型总结
float
1、用途
2、定义方式
salsry = 3.1 # salsry = float(3.1)
print(type(salsry))
>>>:
<class 'float'>
3、常用操作+内置方法
4、该类型总结
补充
长整型 long(在python中(python3中没有长整型的概念)):
C:Users野>Python
Python 2.7.18 (v2.7.18:8d21aa21f2, Apr 20 2020, 13:25:05) [MSC v.1500 64 bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> x=1111111111111111111111111111111
>>> type(x)
<type 'long'>
>>> x
1111111111111111111111111111111L
进制转换
详情参见:https://blog.csdn.net/weixin_42167759/article/details/85604546
字符串类型
1、用途
2、定义方式
在'' "" """""" '''''' 内包含一串字符
1、上述引号定义出来的都是str类型,没有区别
2、三引号可以存放多行字符串
3、引号的嵌套:外层双引号,内层只能用单引号
数据类型转换:str可以把任意类型转换成字符串类型
res = str([1,2,3])
print(res,type(res))
>>>:
[1, 2, 3] <class 'str'>
补充:转义字符 和 r
在打开文件或正则表达式的时候,在字符串前加 r 和不加 r 是有区别的:
r 是防止字符转义的,如果字符串中出现
的话:
不加 r ,
就会被转义成换行符
加上 r ,
就能保留原有的样子,就说明后面的字符,就是普通的字符了。
print("abc
ddd")
>>>:
abc
ddd
print(r"abc
ddd")
>>>:
abc
ddd
print("abc\nddd")
>>>:
abc
ddd
*********************
file_path = 'D:
ewa.py'
print(file_path)
>>>:
D:
ew.py
file_path = r'D:
ewa.py'
print(file_path)
>>>:
D:
ewa.py
3、常用操作+内置方法
优先掌握的操作
1、按索引取值(正向取+反向取) :只能取
msg = 'hello world'
print(msg[0],type(msg[0])) # 正向取
>>>:
h <class 'str'>
print(msg[-1]) # 反向取
msg[0] = 'H' # 只能取不能改
2、切片(顾头不顾尾,步长)
字符串切片:从字符串中取出相应的元素,重新组成一个新的字符串
语法: 字符串[ 开始元素下标 : 结束元素下标 : 步长 ] # 字符串的每个元素都有正负两种下标
步长:切片间隔以及切片方向,默认值是1;实际意义为从开始取一个数据,跳过步长的长度,再取一个数据,一直到结束索引
步长为正值:开始索引默认为0, 结束索引默认为最后是len()+1,从开始索引从左往右走;
步长为负值:开始索引默认为-1, 结束索引默认为开始,不能认为是0,也不能认为是-1,从开始索引从右往左走;
msg = 'hello world'
res1 = msg[1:7]
res2 = msg[1:7:2]
print(res1)
print(res2)
print(msg)
>>>:
ello w
el
hello world
复制:
res = msg[:]
print(res)
>>>:
hello world
将字符串倒着写出来
res = msg[::-1]
print(res)
3、长度len
msg = 'hello world'
print(len(msg))
>>>:
11
4、成员运算in和not in
msg = 'hello world'
print('he' in msg)
print('he' not in msg) # 推荐
print(not 'he' in msg)
>>>:
True
False
False
5、移除空白strip
msg = ' hello '
print(msg.strip())
>>>:
msg = 'hello world'
msg = '*******hello*******'
print(msg.strip('*'))
>>>:
hello
msg = '+*-hello***%/*'
print(msg.strip('+*-/%'))
>>>:
hello
6、切分split
msg = 'egon:123:3000'
res = msg.split(':')
print(res[0])
>>>:
egon
res = msg.split(':',1)
print(res)
>>>:
['egon', '123:3000']
7、循环
msg = 'hello word'
for x in msg:
print(x)
需要掌握的操作
1、strip,lstrip,rstrip
msg = '*****hello*****'
print(msg.strip('*'))
print(msg.lstrip('*')) #去除左边指定字符
print(msg.rstrip('*')) #去除右边指定字符
>>>:
hello
hello*****
*****hello
2、lower,upper
msg = 'hello word'
print(msg.lower())
print(msg.upper())
>>>:
hello word
HELLO WORD
3、startswith,endswith
msg = 'egon is ok'
print(msg.startswith('egon'))
print(msg.startswith('eg'))
print(msg.endswith('ok'))
>>>:
True
True
True
4、format的三种玩法
msg = 'my name is %s ,my age is %s' %('egon',18)
msg = 'my name is {name} ,my age is {age}'.format(age = 18,name = 'egon')
msg = 'my name is {1} ,my age is {0}{0}'.format(18,'egon')
print(msg)
print(msg)
print(msg)
>>>:
my name is egon ,my age is 18
my name is egon ,my age is 18
my name is egon ,my age is 1818
补充:
msg = 'my name is {name} ,my age is {age}'.format(**{'age':18,'name':'egon'})
msg = 'my name is %(name)s ,my age is %(age)s' %{'age':18,'name':'egon'}
print(msg)
print(msg)
>>>:
my name is egon ,my age is 18
my name is egon ,my age is 18
name = 'egon'
age = 18
res = f'my name is {name} my age is {age}'
print(res)
>>>:
my name is egon my age is 18
5、split,rsplit
msg = 'egon:18:3000'
print(msg.split(":",1))
print(msg.rsplit(":",1))
>>>:
['egon', '18:3000']
['egon:18', '3000']
6、join
msg = 'egon:18:3000'
l = msg.split(":")
print(l)
res = ":".join(l)
print(res)
>>>:
['egon', '18', '3000']
egon:18:3000
7、replace
msg = 'egon xxx egon yyy egon'
res = msg.replace('egon','Egon',1)
res = msg.replace('egon','Egon',-1)
res = msg.replace('egon','Egon')
print(res)
>>>:
Egon xxx egon yyy egon
Egon xxx Egon yyy Egon
Egon xxx Egon yyy Egon
小案例:
msg = '**_+_***he llo***+_**'
res = msg.strip('*_+-').replace(" ",'')
print(res)
8、isdigit
num = input('>>>:')
if num.isdigit():
num = int(num)
print(num > 10)
else:
print('必须输入数字,小垃圾')
其他操作(了解即可)
1、find,rfind,index,rindex,count
msg = 'hello xxelx yyely abc'
res =msg.find('el')
res =msg.rfind('el')
print(res)
res1 = msg.index('el')
res2 = msg.find('nnn') # 找不到返回-1
res2 = msg.index('nnn') # 找不到则报错
print(res1)
print(res2)
2、center,ljust,rjust,zfill
print('hello'.center(20,'*')) # *******hello********
print('hello'.ljust(20,'*')) # hello***************
print('hello'.rjust(20,'*')) # ***************hello
print('hello'.zfill(20)) # 000000000000000hello
3、captalize,swapcase,title
print('hello'.capitalize()) # Hello
print('aAbB'.swapcase()) # AaBb
print('hello word'.title()) # Hello Word
4、is数字系列
在python3中
num1=b'4' #bytes
num2=u'4' #unicode,python中无需加u就是unicode
num3='四' # 中文数字
num4='Ⅳ' #罗马数字
bytes、unicode
print(num1.isdigit()) # True
print(num2.isdigit()) # True
print(num3.isdigit()) # False
print(num4.isdigit()) # False
unicode
print(num2.isdecimal()) # True
print(num3.isdecimal()) # False
print(num4.isdecimal()) # False
unicode、中文数字、罗马数字
print(num2.isnumeric()) # True
print(num3.isnumeric()) # True
print(num4.isnumeric()) # True
5、is其他
name = 'egon123'
print(name.isalpha()) # False 只能由字母组成
print(name.isalnum()) # True 由字母或数字组成
print(name.islower()) # 是否全是小写
print(name.isupper()) # 是否全是大写
name = ' '
print(name.isspace()) # True
name = 'Hell oword'
print(name.istitle()) # False
4、该类型总结
5、习题
写代码,有如下变量,请按照要求实现每个功能 (共6分,每小题各0.5分)
name = " aleX"
1) 移除 name 变量对应的值两边的空格,并输出处理结果
res = name.strip()
print(res) #aleX
2) 判断 name 变量对应的值是否以 "al" 开头,并输出结果
res1 = name.startswith('al')
res2 = name.strip().startswith('al')
print(res1) # False
print(res12) # True
3) 判断 name 变量对应的值是否以 "X" 结尾,并输出结果
res = name.endswith('X')
print(res) # True
4) 将 name 变量对应的值中的 “l” 替换为 “p”,并输出结果
res = name.replace('l','p')
print(res) # apeX
5) 将 name 变量对应的值根据 “l” 分割,并输出结果。
res = name.split('l')
print(res) # [' a', 'eX']
6) 将 name 变量对应的值变大写,并输出结果
res = name.upper()
print(res) # ALEX
7) 将 name 变量对应的值变小写,并输出结果
res = name.lower()
print(res) # alex
8) 请输出 name 变量对应的值的第 2 个字符?
res = name[1]
print(res) # a
9) 请输出 name 变量对应的值的前 3 个字符?
res = name[0:3]
print(res) # al
10) 请输出 name 变量对应的值的后 2 个字符?
res = name[-2::]
print(res) #eX
11) 请输出 name 变量对应的值中 “e” 所在索引位置?
res = name.index('e')
print(res) # 3
12) 获取子序列,去掉最后一个字符。如: oldboy 则获取 oldbo。
str1 = 'oldboy'
res = str1[:-1]
print(res)
6、字符串格式化的三种方式
"""
方式一 最方便的
缺点:需要一个一个的格式化
print('hello %s and %s' %('egon','tom'))
方式二 最好用的
优点:不需要一个一个的格式化,可以利用字典的方式,缩短时间
print('hello %(first) and %(second)' %{'first':'egon','second':'tom'})
方式三 最先进的
优点:可续型强
print('hello {first} and {second}'.format(first='egon',second='tom'))
简写:
name = 'egon'
res = f'hello {name}'
"""
列表
1、用途
2、定义方式
在[ ]内用逗号分隔开多个任意类型的值
l = [111,2222,333,'xxx',[11,22,33]] # l = list(...)
print(type(l)) #<class 'list'>
list数据类型转换,把可迭代的类型转成列表,可以被for循环遍历的类型
res = list('hello')
print(res) # ['h', 'e', 'l', 'l', 'o']
res =list({'k1':111,'k2':222})
print(res) # ['k1', 'k2']
res = list(1000) #TypeError: 'int' object is not iterable
print(list(range(10))) # [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
3、常用操作+内置方法
优先掌握的操作
优先掌握的操作:
1、按索引存取值(正向存取+反向存取):即可存也可以取
l = [111,222,333]
print(id(l)) # 1555661667584
l[0] = 666
print(l) # [666, 222, 333]
print(id(l)) # 1555661667584
l = [111,222,333]
l[0] = 666
l[3] = 777 # 列表索引不能超出范围
2、切片(顾头不顾尾,步长)
切片: 变量的名字[start: end: step]
start的默认值是按照step方向上的第一个元素
end的默认值是按照step的方向上的最后一个元素
step的默认值是1
list = [111,222,333]
print(list[::-1]) # [333, 222, 111]
3、长度
l = [111,222,333]
print(len(l)) # 3
4、成员运算in和not in
l = [111,222,333]
print(111 in l) # True
print(111 not in l) # False
5、追加
l = [111,222,333]
l.append(444)
l.append(555)
print(l) # [111, 222, 333, 444, 555]
5.1插入
l = [111,222,333]
l.insert(1,666)
print(l) # [111, 666, 222, 333]
6、删除
l = [111,222,333]
del l[0] # 万能删除
print(l) # [222, 333]
l.remove(指定元素)
l.remove(222)
print(l) # [111, 333]
2、l.pop(指定索引)
res = l.pop(1)
print(res) # 222
print(l) # [111, 333]
7、循环
l = [111,222,333]
for x in l:
print(x)
>>>:
111
222
333
需要掌握的操作
l = [11,22,33,44,55]
# l.copy()
new_l = l.copy() # 浅拷贝 new_l = l[:]
# len()
print(l)
print(len(l)) # 5
# l.index()
print(l.index(33)) # 2
print(l.index(77)) # 找不到报错
# l.count()
print(l.count(33)) # 1
# l.clear()
l.clear()
print(l) # []
# l.extend()
l.extend('hello')
print(l) # [11, 22, 33, 44, 55, 'h', 'e', 'l', 'l', 'o']
l.append([1,2,3])
print(l) # [11, 22, 33, 44, 55, [1, 2, 3]]
# l.reverse()
l.reverse()
print(l) # [55, 44, 33, 22, 11]
# l.sort()
l=[11,-3,9,7,99,73]
l.sort()
print(l) # [-3, 7, 9, 11, 73, 99]
l.sort(reverse=True)
print(l) # [99, 73, 11, 9, 7, -3]
4、该类型的总结
5、深浅拷贝
详情参见:https://blog.csdn.net/bufengzj/article/details/90486991
https://www.cnblogs.com/ZhZhang12138/p/14177914.html#yiliebiao
1、浅拷贝
对于浅copy来说,第一层创建的是新的内存地址,而从第二层开始,指向的都是同一个内存地址,所以,对于第二层以及更深的层数来说,保持一致性。
2、深拷贝
1)需要导入 copy 模块。
Import copy
2)运用copy.deepcopy()
元组
1、用途
元组就相当于一种不可变的列表,所以说元组也是按照位置存放多个任意类型的元素
2、定义方式
在()内用逗号分割开多个任意类型的元素
t = (11,22,33,'xxx',[44,55]) #t = tuple(...)
print(t[-1][0]) # 44
print(type(t)) # <class 'tuple'>
数据类型转换
tuple(可迭代的类型)
注意:如果元组内只有一个元素,那么必须用逗号分隔
t = (11,)
print(type(t)) # <class 'tuple'>
3、常用操作+内置方法
优先掌握的操作
1、按索引取值(正向取+反向取):只能取
t = (11,22,33)
t[0] = 7777 # 报错,元组是不可变类型
2、切片(顾头不顾尾,步长)
t = (11,222,33,44,55,66)
print(t[0:4:2]) # (11, 33)
3、长度
t = (11,22,33,'xxx',[44,55])
print(len(t)) # 5
4、成员运算in和not in
t = (11,22,33,[44,55,666])
print([44,55,666] in t) # True
print([44,55,666] not in t) # False
#5、循环
t = (11,22,33,[44,55,666])
for x in t:
print(x)
需要掌握的操作
t = (11,22,33,[44,55,666])
print(t.count(33)) # 1
print(t.index(33,1,4)) # 2
4、该类型总结
字典
1、用途
按照key:value的方法存放多个值,其中key对value应该有描述性的效果
2、定义方式
在{ } 内用逗号分隔开对个元素,每个元素都是key:value的组合,其中value可移植任意类型,但key必须是不可变类型,通常是字符串类型,key不能重复
d = {1:11111,1.1:2222,'k1':333,(1,2,3):444}
print(d[1]) # 11111
print(d[1.1]) # 2222
print(d['k1']) # 333
print(d[(1,2,3)]) # 444
数据类型转换
res = dict([('name','egon'),('age',18),('gender','male')])
print(res) # {'name': 'egon', 'age': 18, 'gender': 'male'}
res = dict(a=1,b = 2, c = 3)
print(res) # {'a': 1, 'b': 2, 'c': 3}
创造空字典
d = {}
d = dict()
print(type(d)) # <class 'dict'>
res = {}.fromkeys(['name','age','gender'],None)
print(res) # {'name': None, 'age': None, 'gender': None}
注意:
res = {}.fromkeys(['name','age','gender'],[])
res['name'].append(111)
print(res) # {'name': [111], 'age': [111], 'gender': [111]}
3、常用操作+内置方法
优先掌握的操作
1、按key存取值:可存可取
d = {'k1':111,'k2':222}
print(d['k1']) # 111
d['k1'] = 333
print(d) # {'k1': 333, 'k2': 222}
d['k3'] = 444
print(d) # {'k1': 333, 'k2': 222, 'k3': 444}
2、长度len
d = {'k1':111,'k2':222}
print(len(d)) # 2
3、成员运算in和not in # 判断的是key
d = {'name':'egon','age':18}
print('name' in d) # True
4、删除
d = {'name':'egon','age':18}
del d['name']
print(d) # {'age': 18}
res = d.pop('name')
print(res) # egon
item = d.popitem() # 随机删除,把删除元素以元组形式返回
print(item) # ('age', 18)
5、键keys(),值values(),键值对items()
d = {'name': 'egon', 'age': 18, 'gender': 'male'}
print(d.keys())
print(d.values())
print(d.items())
>>>:
dict_keys(['name', 'age', 'gender'])
dict_values(['egon', 18, 'male'])
dict_items([('name', 'egon'), ('age', 18), ('gender', 'male')])
6、循环
d = {'name': 'egon', 'age': 18, 'gender': 'male'}
for k in d.keys():
print(k)
for k in d.values():
print(k)
for k,v in d.items():
print(k,v)
print(list(d.keys()))
print(list(d.values()))
print(list(d.items()))
>>>:
['name', 'age', 'gender']
['egon', 18, 'male']
[('name', 'egon'), ('age', 18), ('gender', 'male')]
7、d.get()
d = {'name': 'egon', 'age': 18, 'gender': 'male'}
print(d['name']) # egon
print(d.get('name')) # egon
print(d['xxx']) # 报错
print(d.get('xxx')) # 返回None
需要掌握的操作
d = {'name': 'egon', 'age': 18, 'gender': 'male'}
d.clear()
print(d) # {}
d.copy() # 浅拷贝
d.setdefault()
key不存在则添加key:value,key如果存在则什么都不做
d = {'name': 'egon', 'age': 18}
d.setdefault('gender','male')
print(d) # {'name': 'egon', 'age': 18, 'gender': 'male'}
d.update # 更新字典
d.update({'k1':111,'name':'xxx'})
print(d) # {'name': 'xxx', 'age': 18, 'gender': 'male', 'k1': 111}
4、该类型总结
5、拓展:python中字典和json的区别
"""
python中,json和dict非常类似,都是key-value的形式,而且json、dict也可以非常方便的通过dumps、loads互转
json:是一种数据格式,是纯字符串。可以被解析成Python的dict或者其他形式。
dict:是一个完整的数据结构,是对Hash Table这一数据结构的一种实现,是一套从存储到提取都封装好了的方案。它使用内置的哈希函数来规划key对应value的存储位置,从而获得O(1)的数据读取速度。
"""
json和dict对比
"""
json的key只能是字符串,python的dict可以是任何可hash对象(hashtable type);
json的key可以是有序、重复的;dict的key不可以重复。
json的value只能是字符串、浮点数、布尔值或者null,或者它们构成的数组或者对象。
json任意key存在默认值undefined,dict默认没有默认值;
json访问方式可以是[],也可以是.,遍历方式分in、of;dict的value仅可以下标访问。
json的字符串强制双引号,dict字符串可以单引号、双引号;
dict可以嵌套tuple,json里只有数组。
json:true、false、null
python:True、False、None
json中文必须是unicode编码,如"u6211".
json的类型是字符串,字典的类型是字典。
"""
json扩展
import json
from datetime import date,datetime
class MyJsonEncoder(json.JSONEncoder):
def default(self, o):
# o其实就是我们即将要序列化的对象
if isinstance(o,date):
return o.strftime('%Y-%m-%d')
if isinstance(o,datetime):
return o.strftime('%Y-%m-%d %X')
return o
d = {'time1':date.today(),'time2':datetime.today()}
res = json.dumps(d,cls=MyJsonEncoder)
print(res)
"""
TypeError: Object of type date is not JSON serializable
"""
集合
1、用途
去重
(1) 无法保证顺序
(2) 只能针对不可元素去重
关系运算
2、定义方式
在{}内用逗号分隔开多个元素,集合内元素的特征有三个:
(1)集合内元素必须是不可变元素
(2)集合内元素不能重复
(3)集合内元素无序
s = {11,11,22,33,33} # s = set(...)
print(s) # {33, 11, 22}
print(type(s)) # <class 'set'>
数据类型转换
res = set('hello')
print(res) # {'l', 'o', 'e', 'h'}
# 定义一个空集合
s = set()
print(type(s)) # <class 'set'>
3、常用操作+内置方法
优先掌握的操作
1、长度len
s1 = {1,2,3}
print(len(s1)) # 3
2、成员运算in和not in
s1 = {1,2,3}
print(3 in s1) # True
print(3 not in s1) # False
1、|并集(合集):两个集合并到一起
2、&交集:取两个集合的共同部分
3、-差集:一个集合减掉与另一个集合共同的部分
4、^对称差集(交叉补集):求两个集合互相减,然后再并到一起
5、==
6、父集:>,>= 当一个集合完全包含了另外一个集合,该集合才能称为爹
s1 = {1,2,3}
s2 = {4,5,6}
print(s1 >= s2) # False
print(s1.issuperset(s2))
7、子集:<,<=
print(s1 <= s2) # False
print(s1.issubset(s2))
举例:
pythons={'alex','egon','yuanhao','wupeiqi','gangdan','biubiu'}
linuxs={'wupeiqi','oldboy','gangdan'}
list1 = pythons | linuxs # {'egon', 'yuanhao', 'wupeiqi', 'oldboy', 'biubiu', 'gangdan', 'alex'}
print(pythons.intersection(linuxs))
print(pythons.intersection_update(linuxs))
list2 = pythons & linuxs # {'wupeiqi', 'gangdan'}
print(pythons.union(linuxs))
list3 = pythons - linuxs # {'yuanhao', 'alex', 'egon', 'biubiu'}
print(pythons.difference(linuxs))
list4 = linuxs - pythons # {'oldboy'}
print(linuxs.difference(pythons))
list5 = pythons ^ linuxs # {'egon', 'oldboy', 'yuanhao', 'biubiu', 'alex'}
print(pythons.symmetric_difference(linuxs))
需要掌握的操作
s1 = {1,2,3}
# 1、s1.update()
s1.update({3,4,5})
print(s1) # {1, 2, 3, 4, 5}
# 2、s1.add()
s1.add(4)
print(s1) # {1, 2, 3, 4}
# 3、删除
# 3.1 remove(3)
s1.remove(3)
print(s1) # {1, 2}
# 3.2 pop()
res = s1.pop() # 随机删除 返回删除的值
print(s1)
print(res)
# 3.3 discard()
s1.discard(444444) # 删除不存在的元素不会报错
print(s1) # {1, 2, 3}
# 3.4 clear()
s1.clear()
print(s1) # set()
# 4、copy
res = s1.copy()
print(res) # {1, 2, 3}
# 5、isdisjoint()
s1 = {1,2,3}
s2 = {4,5,6}
print(s1.isdisjoint(s2)) # True
4、该类型总结
布尔
"""
布尔值,一个True 一个 False,即判断一个条件成立时,用True标识,不成立则用False标识。
#所有数据类型都自带布尔值
1、None,0,空(空字符串,空列表,空字典等)三种情况下布尔值为False
2、其余均为真
"""
数据类型总结
按存值个数区分
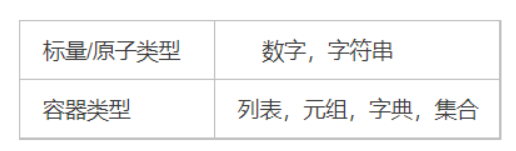
按可变不可变区分
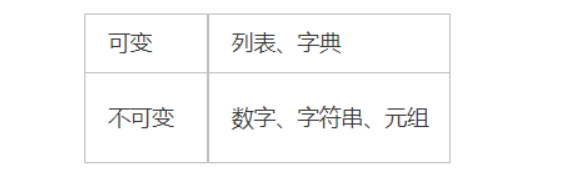
按访问顺序区分
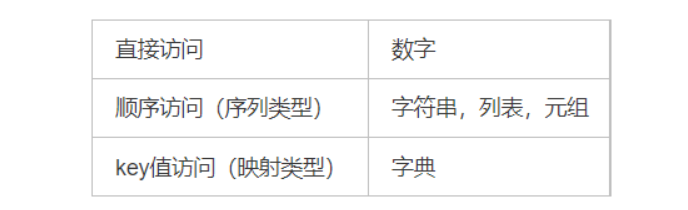