1. 将新闻的正文内容保存到文本文件。 def getNewsDetail(content): f=open('gzcc.txt','a',encoding='utf-8') f.write(content) f.close()
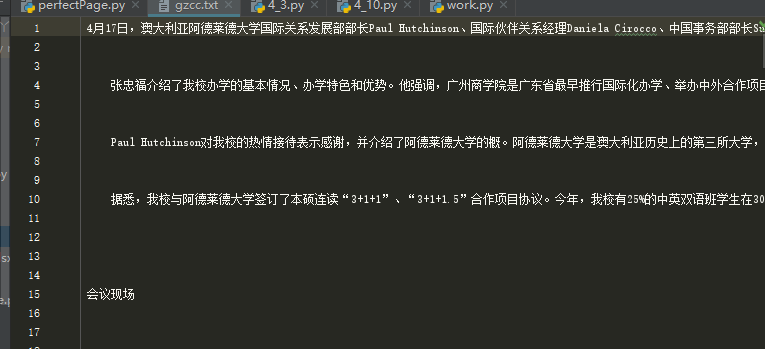
2. 将新闻数据结构化为字典的列表: #获取新闻详情信息 def getNewsDetail(newsUrl): resd=requests.get(newsUrl) resd.encoding='utf-8' soupd=BeautifulSoup(resd.text,'html.parser')#打开新闻详情页 news={} news['title ']= soupd.select('.show-title')[0].text info = soupd.select('.show-info')[0].text news['dt'] = datetime.strptime(info.lstrip('发布时间:')[0:19], '%Y-%m-%d %H:%M:%S') if info.find('来源:') > 0:#作者:审核:来源:摄影: news['source'] = info[info.find('来源:'):].split()[0].lstrip('来源:') else: news['source'] = 'none' news['content'] = soupd.select('.show-content')[0].text.strip() getNewsDetail(news) news['click'] = getClickCount(newsUrl) news['click']=getClickCount(newsUrl) news['newsUrl']=newsUrl return(news) # dt:代表时间,titlr:代表标题,a:代表链接,source:none,click:代表点击次数 3. 安装pandas,用pandas.DataFrame(newstotal),创建一个DataFrame对象df. import pandas newstotal = [{}] df = pandas.DataFrame(newstotal) 4. 通过df将提取的数据保存到csv或excel 文件。 import openpyxl df.to_excel('gzccnews.xlsx') 5. 用pandas提供的函数和方法进行数据分析: print(df[['title','clickCount','source']][:6]) print(df[(df['clickCount']>3000)&(df['source']=='学校综合办')]) sou = ['国际学院','学生工作处'] print(df[df['source'].isin(sou)]) # 进取2018年3月的新闻 df1 = df.set_index('time') print(df1['2018-03']) 6. 保存到sqlite3数据库 import sqlite3 with sqlite3.connect('gzccnewsdb.sqlite') as db: df3.to_sql('gzccnews05',con = db, if_exists='replace') 7. 从sqlite3读数据 with sqlite3.connect('gzccnewsdb.sqlite') as db: df2 = pandas.read_sql_query('SELECT * FROM gzccnews05',con=db) print(df2) 8. df保存到mysql数据库 #安装SQLALchemy #安装PyMySQL #MySQL里创建数据库:create database gzccnews charset utf8; import pymysql from sqlalchemy import create_engine conn = create_engine('mysql+pymysql://root:root@localhost:3306/gzccnews?charset=utf8') pandas.io.sql.to_sql(df, 'gzccnews', con=conn, if_exists='replace') #MySQL里查看已保存了数据。(通过MySQL Client或Navicate。)