排序原理:
1.把所有的元素分为两组,已经排序的和未排序的;
2.找到未排序的组中的第一个元素,向已经排序的组中进行插入;
3.倒叙遍历已经排序的元素,依次和待插入的元素进行比较,直到找到一个元素小于等于待插入元素,那么就把待插入元素放到这个位置,其他的元素向后移动一位;
排序过程:
例:{4,3,2,10,12,1,5,6}
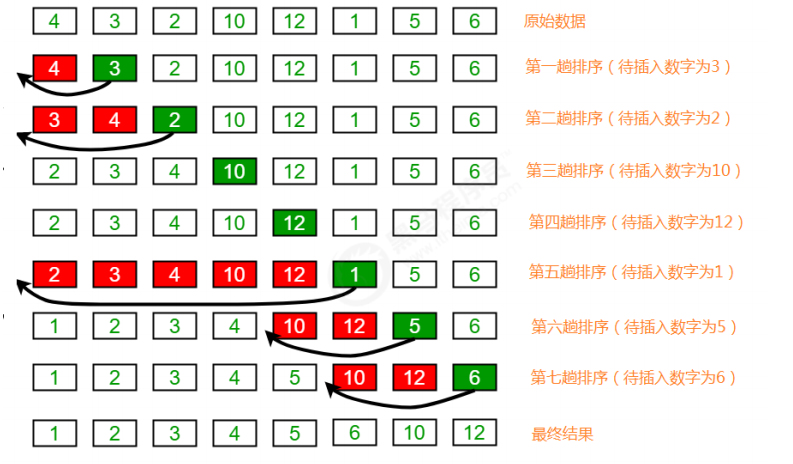
package com.sort;
/*--------------
* Author:Real_Q
* Date:2021-01-06
* Time:13:49
* Description:插入排序
* 小------>大
* {4,3,2,10,12,1,5,6}
---------------*/
/*
分析:
1.数组分两段,有序为一段,无序为一段
2..从无序中拿到一个数,插入有序序列中
//获得数据
//与有序序列比较大小,交换位置,直到位置合适停止
3.完成排序
*/
public class InsertSort {
//排序
public static void insertSort(Comparable[] comparables) {
//刚开始,有序段为第一个元素,无序段为第一个元素后面的所有元素
for (int i = 0; i < comparables.length -1 ; i++) {
//倒叙遍历与有序段进行比较,交换位置
for (int j = i + 1; j > 0; j--) {
//i是无序段元素,j是有序段元素
if (Comparable(comparables[j-1], comparables[j])) {
exchange(comparables, j, j-1);
} else {
break;
}
}
}
}
//比较大小
public static boolean Comparable(Comparable comparable1, Comparable comparable2) {
return comparable1.compareTo(comparable2) > 0;
}
//交换元素
public static void exchange(Comparable[] comparable, int leftIndex, int rightIndex) {
Comparable temp;
temp = comparable[leftIndex];
comparable[leftIndex] = comparable[rightIndex];
comparable[rightIndex] = temp;
}
}
测试类:
import java.util.Arrays;
import static com.sort.InsertSort.insertSort;
public class TestInsert {
public static void main(String[] args) {
Integer[] array = {4,3,2,10,12,1,5,6};
insertSort(array);
System.out.println(Arrays.toString(array));
}
}