诡异的楼梯
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 131072/65536 K (Java/Others)
Total Submission(s): 3323 Accepted Submission(s): 746
Problem Description
Hogwarts正式开学以后,Harry发现在Hogwarts里,某些楼梯并不是静止不动的,相反,他们每隔一分钟就变动一次方向.
比 如下面的例子里,一开始楼梯在竖直方向,一分钟以后它移动到了水平方向,再过一分钟它又回到了竖直方向.Harry发现对他来说很难找到能使得他最快到达 目的地的路线,这时Ron(Harry最好的朋友)告诉Harry正好有一个魔法道具可以帮助他寻找这样的路线,而那个魔法道具上的咒语,正是由你纂写 的.
比 如下面的例子里,一开始楼梯在竖直方向,一分钟以后它移动到了水平方向,再过一分钟它又回到了竖直方向.Harry发现对他来说很难找到能使得他最快到达 目的地的路线,这时Ron(Harry最好的朋友)告诉Harry正好有一个魔法道具可以帮助他寻找这样的路线,而那个魔法道具上的咒语,正是由你纂写 的.
Input
测试数据有多组,每组的表述如下:
第 一行有两个数,M和N,接下来是一个M行N列的地图,'*'表示障碍物,'.'表示走廊,'|'或者'-'表示一个楼梯,并且标明了它在一开始时所处的位 置:'|'表示的楼梯在最开始是竖直方向,'-'表示的楼梯在一开始是水平方向.地图中还有一个'S'是起点,'T'是目标,0<=M,N& lt;=20,地图中不会出现两个相连的梯子.Harry每秒只能停留在'.'或'S'和'T'所标记的格子内.
第 一行有两个数,M和N,接下来是一个M行N列的地图,'*'表示障碍物,'.'表示走廊,'|'或者'-'表示一个楼梯,并且标明了它在一开始时所处的位 置:'|'表示的楼梯在最开始是竖直方向,'-'表示的楼梯在一开始是水平方向.地图中还有一个'S'是起点,'T'是目标,0<=M,N& lt;=20,地图中不会出现两个相连的梯子.Harry每秒只能停留在'.'或'S'和'T'所标记的格子内.
Output
只有一行,包含一个数T,表示到达目标的最短时间.
注意:Harry只能每次走到相邻的格子而不能斜走,每移动一次恰好为一分钟,并且Harry登上楼梯并经过楼梯到达对面的整个过程只需要一分钟,Harry从来不在楼梯上停留.并且每次楼梯都恰好在Harry移动完毕以后才改变方向.
注意:Harry只能每次走到相邻的格子而不能斜走,每移动一次恰好为一分钟,并且Harry登上楼梯并经过楼梯到达对面的整个过程只需要一分钟,Harry从来不在楼梯上停留.并且每次楼梯都恰好在Harry移动完毕以后才改变方向.
Sample Input
5 5
**..T
**.*.
..|..
.*.*.
S....
**..T
**.*.
..|..
.*.*.
S....
Sample Output
7
地图如下:
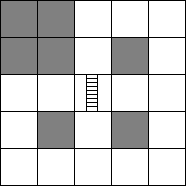
Hint
Hint地图如下:
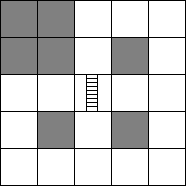
两次弄错题义,悲剧啊,首先是楼梯是每一秒钟发生变化,在走到楼梯周围时要求楼梯是对着你的。然后就是人可以在原地停留,等待楼梯的转动。所以到达楼梯时,就有多种选择了,BFS结构被打破,所以加上优先队列。
代码如下:
#include <cstdio> #include <cstring> #include <iostream> #include <queue> using namespace std; char map[25][25]; int hash[2][25][25], dir[4][2]= { 1, 0, -1, 0, 0, 1, 0, -1 }, sx, sy; struct Node { int x, y, step; bool operator < ( const Node &t ) const { return t.step< step; } }info; int BFS( ) { priority_queue< Node >q; info.x= sx, info.y= sy, info.step= 0; memset( hash, 0, sizeof( hash ) ); hash[0][sx][sy]= 1; q.push( info ); while( !q.empty() ) { Node pos= q.top(); q.pop(); if( map[ pos.x ][ pos.y ]== 'T' ) { return pos.step; } for( int i= 0; i< 4; ++i ) { int x= pos.x+ dir[i][0], y= pos.y+ dir[i][1], step= pos.step+ 1; if( map[x][y]!= 0&& map[x][y]!= '*' ) { int k= ( step )% 2; // 求出该点奇偶性 if( ( map[x][y]== '.'|| map[x][y]== 'T' )&& !hash[k][x][y] ) { info.x= x, info.y= y, info.step= step; hash[k][x][y]= 1; q.push( info ); } else if( i> 1&& !hash[k][ x ][ y+ dir[i][1] ] ) { if( map[x][y]== '-' ) { if( k== 1 ) { info.x= x, info.y= y+ dir[i][1], info.step= step; hash[k][x][ y+ dir[i][1] ]= 1; q.push( info ); } else if( k== 0 ) { info.x= x, info.y= y+ dir[i][1], info.step= step+ 1; hash[k][x][ y+ dir[i][1] ]= 1; q.push( info ); } } if( map[x][y]== '|' ) { if( k== 0 ) { info.x= x, info.y= y+ dir[i][1], info.step= step; hash[k][x][ y+ dir[i][1] ]= 1; q.push( info ); } else if( k== 1 ) { info.x= x, info.y= y+ dir[i][1], info.step= step+ 1; hash[k][x][ y+ dir[i][1] ]= 1; q.push( info ); } } } else if( i<= 1&& !hash[k][x+ dir[i][0]][y] ) { if( map[x][y]== '-' ) { if( k== 0 ) { info.x= x+ dir[i][0], info.y= y, info.step= step; hash[k][x+ dir[i][0]][y]= 1; q.push( info ); } else if( k== 1 ) { info.x= x+ dir[i][0], info.y= y, info.step= step+ 1; hash[k][ x+ dir[i][0] ][y]= 1; q.push( info ); } } if( map[x][y]== '|' ) { if( k== 1 ) { info.x= x+ dir[i][0], info.y= y, info.step= step; hash[k][x+ dir[i][0]][y]= 1; q.push( info ); } else if( k== 0 ) { info.x= x+ dir[i][0], info.y= y, info.step= step+ 1; hash[k][ x+ dir[i][0] ][y]= 1; q.push( info ); } } } } } } } int main() { int N, M; while( scanf( "%d %d", &N, &M )== 2 ) { memset( map, 0, sizeof( map ) ); for( int i= 1; i<= N; ++i ) { scanf( "%s", map[i]+ 1 ); for( int j= 1; j<= M; ++j ) { if( map[i][j]== 'S' ) { sx= i, sy= j; } } } printf( "%d\n", BFS( ) ); } return 0; }