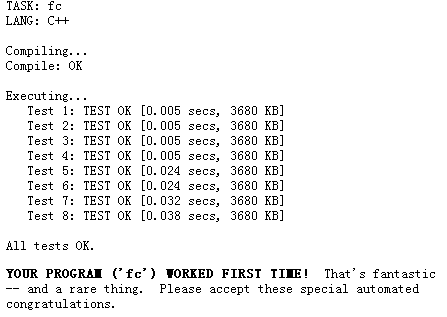
裸的凸包..很好写,废话不说,直接贴代码。
-----------------------------------------------------------------------------
#include<cstdio>
#include<cstring>
#include<iostream>
#include<algorithm>
#include<cmath>
#define rep(i,r) for(int i=0;i<r;i++)
using namespace std;
const int maxn=10000+5;
struct P {
double x,y;
P(double x=0,double y=0):x(x),y(y) {}
P operator + (P o) { return P(x+o.x,y+o.y); }
P operator - (P o) { return P(x-o.x,y-o.y); }
bool operator == (const P &o) const {
return x==o.x && y==o.y;
}
bool operator < (const P &o) const {
return x<o.x || (x==o.x && y<o.y);
}
};
P ans[maxn],p[maxn];
inline double cross(P a,P b) { return a.x*b.y-a.y*b.x; }
inline double dist(P o) { return sqrt(o.x*o.x+o.y*o.y); }
double convexHull(int n) {
sort(p,p+n);
int m=0;
rep(i,n) {
while(m>1 && cross(ans[m-1]-ans[m-2],p[i]-ans[m-2])<=0) m--;
ans[m++]=p[i];
}
int k=m;
for(int i=n-2;i>=0;i--) {
while(m>k && cross(ans[m-1]-ans[m-2],p[i]-ans[m-2])<=0) m--;
ans[m++]=p[i];
}
if(n>1) m--;
double length=0;
rep(i,m) length+=dist(ans[i]-ans[i+1]);
return length;
}
int main()
{
freopen("fc.in","r",stdin); freopen("fc.out","w",stdout);
int n;
cin>>n;
rep(i,n) scanf("%lf%lf",&p[i].x,&p[i].y);
printf("%.2lf
",convexHull(n));
return 0;
}
-----------------------------------------------------------------------------
Fencing the Cows
Hal Burch
Farmer John wishes to build a fence to contain his cows, but he's a bit short on cash right. Any fence he builds must contain all of the favorite grazing spots for his cows. Given the location of these spots, determine the length of the shortest fence which encloses them.
PROGRAM NAME: fc
INPUT FORMAT
The first line of the input file contains one integer, N. N (0 <= N <= 10,000) is the number of grazing spots that Farmer john wishes to enclose. The next N line consists of two real numbers, Xi and Yi, corresponding to the location of the grazing spots in the plane (-1,000,000 <= Xi,Yi <= 1,000,000). The numbers will be in decimal format.
SAMPLE INPUT (file fc.in)
4
4 8
4 12
5 9.3
7 8
OUTPUT FORMAT
The output should consists of one real number, the length of fence required. The output should be accurate to two decimal places.
SAMPLE OUTPUT (file fc.out)
12.00