01 字符串的连接
本题要求实现一个函数,将两个字符串连接起来。
函数接口定义:
char *str_cat( char *s, char *t );
函数str_cat
应将字符串t
复制到字符串s
的末端,并且返回字符串s
的首地址。
输入样例:
abc
def
输出样例:
abcdef
abcdef
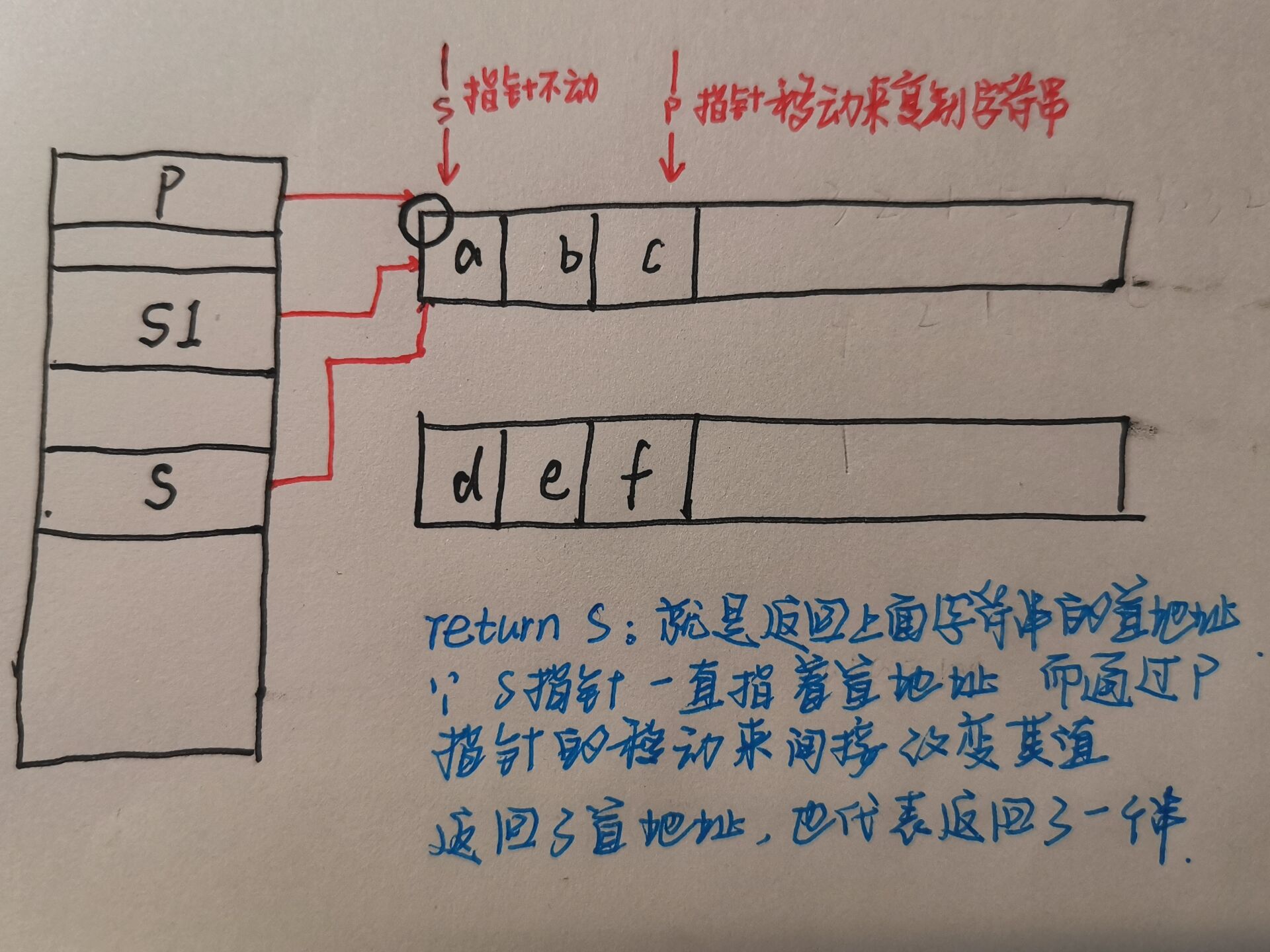
1 #include<stdio.h>
2 #include<string.h>
3 #define MAXS 10
4
5 char *str_cat(char *s,char *t)//内存共享
6 {
7 char * p=s;
8 while(*p!='\0')
9 {
10 p++;
11 }
12 while(*t!='\0')
13 {
14 *p=*t;
15 t++;
16 p++;
17 }
18 *p='\0';
19 return s; //声明一个指针间接改变了s的值,返回一个地址返回了一个串
20 }
21 int main()
22 {
23 char *p;
24 char str1[MAXS+MAXS]={'\0'},str2[MAXS]={'\0'};
25 scanf("%s%s",str1,str2);
26 p=str_cat(str1,str2);
27 printf("%s\n%s\n",p,str1);
28 return 0;
29 }
02 使用函数实现字符串部分复制
本题要求编写函数,将输入字符串t中从第m个字符开始的全部字符复制到字符串s中。
函数接口定义:
void strmcpy( char *t, int m, char *s );
函数strmcpy
将输入字符串char *t
中从第m
个字符开始的全部字符复制到字符串char *s
中。若m
超过输入字符串的长度,则结果字符串应为空串。
输入样例:
7
happy new year
输出样例:
new year
1 #include <stdio.h>
2 #include<string.h>
3 #define MAXN 20
4 void strmcpy( char *t, int m, char *s )//通过指针返回
5 {
6 int strTlen=strlen(t);
7 int i;
8 char *p=s;//通过p指针来移动,间接改变值,然后通过s指针指向的首地址返回。
9 if(m>strTlen)
10 {
11 *p='\0';
12 }
13 else
14 {
15 for(i=m-1;i<strTlen;i++)
16 {
17 *p=t[i];
18 p++;
19 }
20 *p='\0';
21 }
22 }
23
24 /* 由裁判实现,略去不表 */
25
26 int main()
27 {
28 char t[MAXN], s[MAXN];
29 int m;
30
31 scanf("%d\n", &m);
32 gets(t);
33 strmcpy( t, m, s );
34 printf("%s\n", s);//数组首地址
35
36 return 0;
37 }