Ultra-QuickSort
Time Limit: 7000MS | Memory Limit: 65536K | |
Total Submissions: 48257 | Accepted: 17610 |
Description
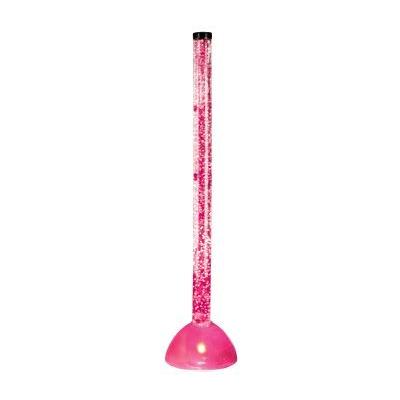
Ultra-QuickSort produces the output
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
The input contains several test cases. Every test case begins with a line that contains a single integer n < 500,000 -- the length of the input sequence. Each of the the following n lines contains a single integer 0 ≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
For every input sequence, your program prints a single line containing an integer number op, the minimum number of swap operations necessary to sort the given input sequence.
Sample Input
5 9 1 0 5 4 3 1 2 3 0
Sample Output
6 0
Source
这道题可以总结的地方不少。
1:对于一组乱序数列,每次只能交换相邻元素,达到有序交换的次数就是原数列中你逆序对的个数。
cf上好像总喜欢出这个题。。。我印象中就出现三次了。。。。。
2:原始数组a[i]和树状数组的t[i]的对应问题(???存在疑问。。。应该只是这道题,而不是一般规律!)
这道题n是500000,如果直接开数组是可以开得下的,不需要离散化。但是树状数组的下标对应的是原始数组的值!也就是t[i]的下表最大可能为999,999,999 ! 显然存不下,需要离散化。
3:学习了离散化的又一种写法。
1 /************************************************************************* 2 > File Name: code/poj/2299.cpp 3 > Author: 111qqz 4 > Email: rkz2013@126.com 5 > Created Time: 2015年08月04日 星期二 12时27分32秒 6 ************************************************************************/ 7 8 #include<iostream> 9 #include<iomanip> 10 #include<cstdio> 11 #include<algorithm> 12 #include<cmath> 13 #include<cstring> 14 #include<string> 15 #include<map> 16 #include<set> 17 #include<queue> 18 #include<vector> 19 #include<stack> 20 #define y0 abc111qqz 21 #define y1 hust111qqz 22 #define yn hez111qqz 23 #define j1 cute111qqz 24 #define tm crazy111qqz 25 #define lr dying111qqz 26 using namespace std; 27 #define REP(i, n) for (int i=0;i<int(n);++i) 28 typedef long long LL; 29 typedef unsigned long long ULL; 30 const int inf = 0x7fffffff; 31 const int N=5E5+7; 32 int n; 33 int t[N]; 34 int ref[N]; 35 36 struct Q 37 { 38 int val,id; 39 }q[N]; 40 41 42 bool cmp(Q a,Q b) 43 { 44 if (a.val<b.val) return true; 45 return false; 46 } 47 int lowbit( int x) 48 { 49 return x&(-x); 50 } 51 void update ( int x,int c) 52 { 53 for ( int i = x ; i < N ; i = i + lowbit(i)) 54 { 55 t[i] = t[i] + c; 56 } 57 } 58 LL Sum( int x) 59 { 60 LL res = 0; 61 for ( int i = x; i >= 1 ; i = i - lowbit(i)) 62 { 63 res = res + t[i]; 64 } 65 return res; 66 } 67 int main() 68 { 69 while (~scanf("%d",&n)&&n) 70 { 71 memset(t,0,sizeof(t)); 72 for ( int i = 1 ; i <= n ; i++ ) 73 { 74 scanf("%d",&q[i].val); //离散化的时候相对大小不能打乱 75 q[i].id = i; 76 } 77 sort(q+1,q+n+1,cmp); 78 for ( int i = 1 ; i <= n ; i++ ) 79 { 80 ref[q[i].id] = i; 81 } 82 LL ans = 0; 83 for ( int i = 1 ; i <= n ; i++ ) 84 { 85 update(ref[i],1); 86 ans = ans + i-Sum(ref[i]); 87 // cout<<"ans:"<<ans<<endl; 88 89 } 90 cout<<ans<<endl; 91 } 92 return 0; 93 }