Second My Problem First
Time Limit: 12000/4000 MS (Java/Others) Memory Limit: 65536/65536 K (Java/Others)
Total Submission(s): 1673 Accepted Submission(s): 634
Then we define Si = Ai mod B and Ti = Min{ Sk | i-A <= k <= i, k >= 1}
Your task is to calculate the product of Ti (1 <= i <= n) mod B.
Process to end of file.

1 #include<stdio.h> 2 #include<algorithm> 3 #include<iostream> 4 #include<string.h> 5 #include<math.h> 6 #include<stack> 7 #include<set> 8 #include<queue> 9 #include<deque> 10 using namespace std; 11 typedef long long LL; 12 using namespace std; 13 int ans[10000005]; 14 int ic[10000005]; 15 int main(void) 16 { 17 LL n,a,b; 18 while(scanf("%lld %lld %lld",&n,&a,&b)!=EOF) 19 { 20 int i,j; 21 LL ask = 1; 22 ans[1] = a%b; 23 int l = 1,r = 0; 24 for(i = 2; i <= n; i++) 25 ans[i] =(int) ((LL)ans[i-1]*(LL)a%b); 26 for(i = 1; i <= n; i++) 27 { 28 int x = max(i-a,(LL)1); 29 if(l > r) 30 { 31 ic[++r] = i; 32 ask =((LL)ask*(LL)ans[i]%b); 33 } 34 else 35 { 36 while(l <= r) 37 { 38 int c = ic[r]; 39 if(ans[i] <= ans[c]) 40 { 41 r--; 42 } 43 else 44 { 45 ic[++r] = i; 46 break; 47 } 48 if(l > r) 49 { 50 ic[++r] = i; 51 break; 52 } 53 } 54 while(ic[l]<x) 55 { 56 l++; 57 } 58 int maxx =ic[l]; 59 ask = ask*(LL)ans[maxx]%b; 60 } 61 } 62 printf("%lld ",ask); 63 } 64 return 0; 65 }
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 19670 | Accepted: 6360 |
Description
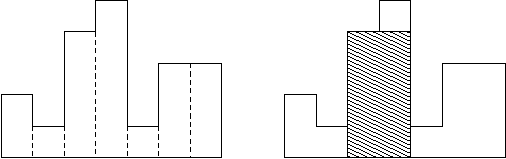
Usually, histograms are used to represent discrete distributions, e.g., the frequencies of characters in texts. Note that the order of the rectangles, i.e., their heights, is important. Calculate the area of the largest rectangle in a histogram that is aligned at the common base line, too. The figure on the right shows the largest aligned rectangle for the depicted histogram.
Input
Output
Sample Input
7 2 1 4 5 1 3 3 4 1000 1000 1000 1000 0
Sample Output
8 4000
Hint

1 #include<stdio.h> 2 #include<algorithm> 3 #include<iostream> 4 #include<string.h> 5 #include<queue> 6 #include<deque> 7 #include<stack> 8 #include<math.h> 9 using namespace std; 10 int ans[100002]; 11 int R[100002]; 12 int L[100002]; 13 stack<int>stac; 14 typedef long long LL; 15 int main(void) 16 { 17 int n; 18 int i,j; 19 while(scanf("%d",&n),n!=0) 20 { 21 for(i = 1; i <= n; i++) 22 scanf("%d",&ans[i]); 23 while(!stac.empty()) 24 stac.pop(); 25 LL maxx = 0; 26 for(i = 1; i <= n;i++) 27 { 28 if(stac.empty()) 29 { 30 L[i] = 1; 31 stac.push(i); 32 } 33 else 34 { 35 while(!stac.empty()) 36 { 37 int x = stac.top(); 38 if(ans[x] > ans[i]) 39 { 40 R[x] = i-1; 41 stac.pop(); 42 } 43 else 44 { 45 L[i] = x+1; 46 stac.push(i); 47 break; 48 } 49 } 50 if(stac.empty()) 51 { 52 L[i] = 1; 53 stac.push(i); 54 } 55 } 56 } 57 while(!stac.empty()) 58 { 59 int x = stac.top(); 60 R[x] = n; 61 stac.pop(); 62 } 63 for(i = 1;i <= n;i++) 64 { 65 maxx = max(maxx,(LL)(R[i]-L[i]+1)*(LL)(ans[i])); 66 } 67 printf("%lld ",maxx); 68 } 69 return 0; 70 }
There are n walruses standing in a queue in an airport. They are numbered starting from the queue's tail: the 1-st walrus stands at the end of the queue and the n-th walrus stands at the beginning of the queue. The i-th walrus has the age equal to ai.
The i-th walrus becomes displeased if there's a younger walrus standing in front of him, that is, if exists such j (i < j), that ai > aj. The displeasure of the i-th walrus is equal to the number of walruses between him and the furthest walrus ahead of him, which is younger than the i-th one. That is, the further that young walrus stands from him, the stronger the displeasure is.
The airport manager asked you to count for each of n walruses in the queue his displeasure.
The first line contains an integer n (2 ≤ n ≤ 105) — the number of walruses in the queue. The second line contains integers ai (1 ≤ ai ≤ 109).
Note that some walruses can have the same age but for the displeasure to emerge the walrus that is closer to the head of the queue needs to be strictly younger than the other one.
Print n numbers: if the i-th walrus is pleased with everything, print "-1" (without the quotes). Otherwise, print the i-th walrus's displeasure: the number of other walruses that stand between him and the furthest from him younger walrus.
6
10 8 5 3 50 45
2 1 0 -1 0 -1
7
10 4 6 3 2 8 15
4 2 1 0 -1 -1 -1
5
10 3 1 10 11
1 0 -1 -1 -1
思路:二分;
题目意思就是找最右边比当前数小的那数与当前数之间隔了多少个数;我们从右边开始,先维护一个单调的队列,当当前的数小于队尾的数的时候
加入队尾,否则不加,这样队列是递减的。这样,如果当前的数大于队尾的数,那么这个数是无用的,因为还未加入的数,要找比他小的肯定是找那个小的数。
那么这样我们就可以二分找答案了。复杂度N*log(N);

1 #include<stdio.h> 2 #include<algorithm> 3 #include<iostream> 4 #include<string.h> 5 #include<queue> 6 #include<deque> 7 #include<stack> 8 #include<math.h> 9 using namespace std; 10 int ans[100005]; 11 int bns[100005]; 12 int id[100005]; 13 int ask[100005]; 14 int main(void) 15 { 16 int n; 17 while(scanf("%d",&n)!=EOF) 18 { 19 int i,j; 20 for(i = 0; i < n; i++) 21 { 22 scanf("%d",&ans[i]); 23 } 24 int head = 0; 25 for(i = n-1; i >= 0; i--) 26 { 27 if(head == 0) 28 { 29 bns[head] = ans[i]; 30 id[head] = i; 31 head++; 32 } 33 else 34 { 35 if(bns[head-1]>ans[i]) 36 { 37 bns[head] = ans[i]; 38 id[head] = i; 39 head++; 40 } 41 } 42 int l = 0; 43 int r = head-1; 44 int ic = -1; 45 while(l<=r) 46 { 47 int mid = (l+r)/2; 48 if(bns[mid] < ans[i]) 49 { 50 ic = mid; 51 r = mid-1; 52 } 53 else 54 { 55 l = mid+1; 56 } 57 } 58 if(ic == -1) 59 ask[i] = -1; 60 else ask[i] = id[ic]-i-1; 61 } 62 printf("%d",ask[0]); 63 for(i = 1; i < n; i++) 64 { 65 printf(" %d",ask[i]); 66 } 67 } 68 return 0; 69 }
Problem 1894 志愿者选拔

Accept: 1895 Submit: 5852
Time Limit: 1500 mSec Memory Limit : 32768 KB
Problem Description
参加志愿者选拔的同学们排队接受面试官们的面试。参加面试的同学们按照先来先面试并且先结束的原则接受面试官们的考查。
面试中每个人的人品是主要考查对象之一。(提高人品的方法有扶老奶奶过街,不闯红灯等)
作为主面试官的John想知道当前正在接受面试的同学队伍中人品值最高的是多少。于是他请你帮忙编写一个程序来计算。
Input
接下来的数据中有三种情况:
输入 | 含义 | |
1 | C NAME RP_VALUE | 名字为NAME的人品值为RP_VALUE的同学加入面试队伍。(名字长度不大于5,0 <= RP_VALUE <= 1,000,000,000) |
2 | G | 排在面试队伍最前面的同学面试结束离开考场。 |
3 | Q | 主面试官John想知道当前正在接受面试的队伍中人品最高的值是多少。 |
所有参加面试的同学总人数不超过1,000,000
Output
Sample Input
Sample Output

1 #include<stdio.h> 2 #include<algorithm> 3 #include<iostream> 4 #include<string.h> 5 #include<queue> 6 #include<deque> 7 #include<stack> 8 #include<math.h> 9 using namespace std; 10 typedef long long LL; 11 char str[1000]; 12 char a[10000]; 13 int cnt[1000005]; 14 int quq[1000006]; 15 int main(void) 16 { 17 int n; 18 scanf("%d",&n); 19 while(n--) 20 { 21 int cn = 0; 22 int hh = 0; 23 int head = 1; 24 int rail = 0; 25 while(scanf("%s",str),str[0]!='E'&&str[1]!='N'&&str[2]!='D') 26 { 27 if(str[0]=='C') 28 { 29 scanf("%s",a); 30 scanf("%d",&cnt[cn]); 31 //printf("%d ",cnt[cn]); 32 if(head > rail) 33 { 34 quq[++rail] = cn; 35 } 36 else 37 { 38 int x = cnt[quq[rail]]; 39 while(x <= cnt[cn]) 40 { 41 rail--; 42 if(rail<head) 43 break; 44 x = cnt[quq[rail]]; 45 } 46 quq[++rail] = cn; 47 } 48 cn++; 49 } 50 else if(str[0]=='G') 51 { 52 if(rail >= head) 53 { 54 int x = quq[head]; 55 //printf("%d %d ",x,hh); 56 if(x == hh) 57 { 58 head++; 59 } 60 hh++; 61 } 62 } 63 else if(str[0]=='Q') 64 { if(head>rail)printf("-1 "); 65 else printf("%d ",cnt[quq[head]]); 66 } 67 } 68 } 69 return 0; 70 }