题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1016
Prime Ring Problem
Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)
Problem Description
A ring is compose of n circles as shown in diagram. Put
natural number 1, 2, ..., n into each circle separately, and the sum of numbers
in two adjacent circles should be a prime.
Note: the number of first circle should always be 1.
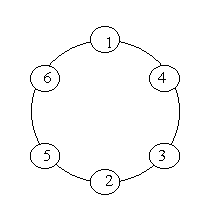
Note: the number of first circle should always be 1.
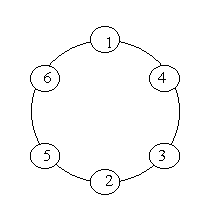
Input
n (0 < n < 20).
Output
The output format is shown as sample below. Each row
represents a series of circle numbers in the ring beginning from 1 clockwisely
and anticlockwisely. The order of numbers must satisfy the above requirements.
Print solutions in lexicographical order.
You are to write a program that completes above process.
Print a blank line after each case.
You are to write a program that completes above process.
Print a blank line after each case.
Sample Input
6
8
Sample Output
Case 1:
1 4 3 2 5 6
1 6 5 2 3 4
Case 2:
1 2 3 8 5 6 7 4
1 2 5 8 3 4 7 6
1 4 7 6 5 8 3 2
1 6 7 4 3 8 5 2
Source
Recommend
题目大意:有一个整数n,把从1到n的数字无重复的排列成环,且使每相邻两个数(包括首尾)的和都为素数,称为素数环。
为了简便起见,我们规定每个素数环都从1开始。有多组测试数据,每组输入一个n(0<n<20),n=0表示输入结束。
输出每组第一行输出对应的Case序号,从1开始。如果存在满足题意叙述的素数环,从小到大输出。
解题思路:回溯的思想就是了,一个dfs搞定,最坑爹的是,每输完一组末尾都要加上换行,我没加结果提交wa,明明是pe,各种改,
彻底无爱了,Orz~~~
代码如下:

1 #include <iostream> 2 #include <cstring> 3 using namespace std; 4 5 #define maxn 40 6 int vis[21], x[21], T, n; 7 int prime[maxn] = { 1, 1, 0 }; 8 void init() 9 { 10 int i, j; 11 for (i = 2; i <= maxn; i++){ 12 if (!prime[i]){ 13 for (j = 2; i*j <= maxn; j++) 14 prime[i*j] = 1; 15 } 16 } 17 } 18 19 void dfs(int cur){ 20 if (cur == n&&!prime[1 + x[n - 1]]){ 21 for (int i = 0; i < n; i++){ 22 if (i) cout << ' '; 23 cout << x[i]; 24 } 25 cout << endl; 26 } 27 else for (int i = 2; i <= n; i++){ 28 if (!vis[i] && !prime[i + x[cur - 1]]){ 29 x[cur] = i; 30 vis[i] = 1; 31 dfs(cur + 1); 32 vis[i] = 0; 33 } 34 } 35 } 36 37 int main(){ 38 init(); 39 while (cin >> n){ 40 cout << "Case " << ++T << ':' << endl; 41 if (n == 1) 42 cout << 1 << endl; 43 else if (n & 1) 44 cout << endl; 45 else{ 46 memset(vis, 0, sizeof(vis)); 47 x[0] = 1; 48 dfs(1); 49 } 50 cout << endl;//没加这一句pe来个wa,我也是醉了,各种改,无爱了~~~~ 51 } 52 return 0; 53 }