Crossings
Time Limit: 2 Sec
Memory Limit: 256 MB
题目连接
http://codeforces.com/gym/100463
Description
In this problem, you have to analyze a particular sorting algorithm. The algorithm processes a sequence of n distinct integers by swapping two adjacent sequence elements until the sequence is sorted in ascending order. For the input sequence
9 1 0 5 4 ,
Ultra-QuickSort produces the output
0 1 4 5 9 .
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Ultra-QuickSort produces the output
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
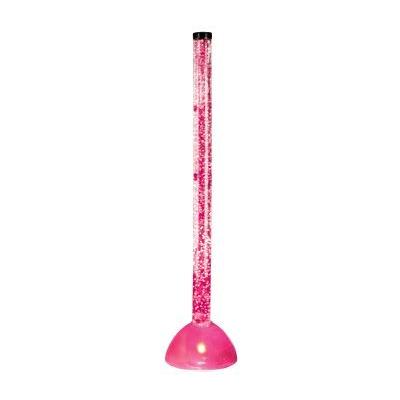
Input
The input contains several test cases. Every test case begins with a line that contains a single integer n < 500,000 -- the length of the input sequence. Each of the the following n lines contains a single integer 0 ≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
For every input sequence, your program prints a single line containing an integer number op, the minimum number of swap operations necessary to sort the given input sequence.
Sample Input
5 9 1 0 5 4 3 1 2 3 0
Sample Output
6 0
HINT
题意
逆序对求解
题解:
树状数组水
代码
1 #include <cstdio> 2 #include <cmath> 3 #include <cstring> 4 #include <ctime> 5 #include <iostream> 6 #include <algorithm> 7 #include <set> 8 #include <vector> 9 #include <queue> 10 #include <map> 11 #include <stack> 12 #define MOD 1000000007 13 #define maxn 32001 14 using namespace std; 15 typedef __int64 ll; 16 inline ll read() 17 { 18 ll x=0,f=1; 19 char ch=getchar(); 20 while(ch<'0'||ch>'9') 21 { 22 if(ch=='-')f=-1; 23 ch=getchar(); 24 } 25 while(ch>='0'&&ch<='9') 26 { 27 x=x*10+ch-'0'; 28 ch=getchar(); 29 } 30 return x*f; 31 } 32 //******************************************************************* 33 34 struct ss 35 { 36 int v,index; 37 } in[500005]; 38 int c[500005]; 39 int a[500005]; 40 int n; 41 bool cmp(ss s1,ss s2) 42 { 43 return s1.v<s2.v; 44 } 45 int lowbit(int x) 46 { 47 return x&(-x); 48 } 49 int getsum(int x) 50 { 51 int sum=0; 52 while(x>0) 53 { 54 sum+=c[x]; 55 x-=lowbit(x); 56 } 57 return sum; 58 } 59 void update(int x,int value) 60 { 61 while(x<=n) 62 { 63 c[x]+=value; 64 x+=lowbit(x); 65 } 66 } 67 int main() 68 { 69 70 while(scanf("%d",&n)!=EOF) 71 { 72 if(n==0) break; 73 memset(c,0,sizeof(c)); 74 for(int i=1; i<=n; i++) 75 { 76 in[i].v=read(); 77 in[i].index=i; 78 } 79 sort(in+1,in+n+1,cmp); 80 for(int i=1; i<=n; i++)a[in[i].index]=i; //离散化处理 81 ll ans=0; 82 for(int i=1; i<=n; i++) 83 { 84 update(a[i],1); 85 ans+=i-getsum(a[i]); 86 } 87 cout<<ans<<endl; 88 } 89 90 return 0; 91 }